Python 简明教程
Python - Variables
Python Variables
Python 变量是用于在 Python 程序中存储值的保留内存位置。这意味着当你创建一个变量时,会在内存中保留一些空间。
基于变量的数据类型, Python interpreter 分配内存并决定在保留内存中可以存储什么。所以,通过向 Python 变量分配不同的 data types ,你可以在这些变量中存储整数、小数或字符。
Memory Addresses
属于不同数据类型的数据项存储在计算机的内存中。计算机的存储器位置具有一个数字或地址,在内部以二进制形式呈现。数据也以二进制形式存储,因为计算机按照二进制表示法的原则工作。在以下图表中,字符串 May 和数字 18 显示为存储在存储器位置中。
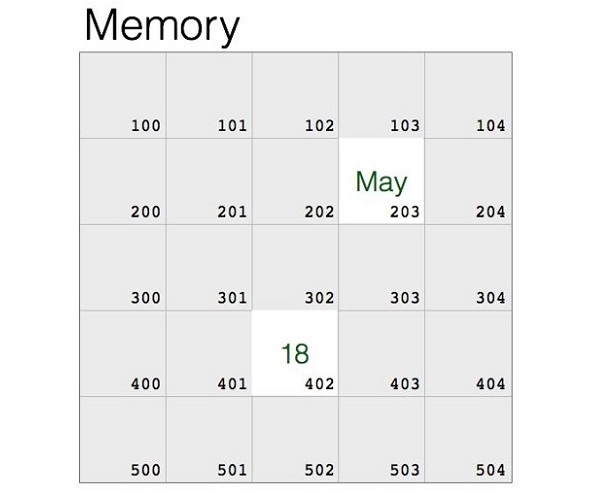
如果您知道汇编语言,您会转换这些数据项和存储器地址,并给出一个机器语言指令。但是,这对于一般人来说并不容易。例如 Python 解释器这样的语言翻译器执行这种类型的转换。它将对象存储在随机选择的存储器位置。Python 的内置 id() 函数返回存储对象的地址。
>>> "May"
May
>>> id("May")
2167264641264
>>> 18
18
>>> id(18)
140714055169352
一旦数据存储在内存中,就应该对它重复访问以便执行某个进程。显然,从其 ID 中提取数据很麻烦。像 Python 这样的高级语言使得为存储器位置提供合适的别名或标签成为可能。
在上述示例中,让我们将 May 的位置标记为 month,并将存储 18 的位置标记为 age。Python 使用赋值运算符 (=) 将对象与标签绑定。
>>> month="May"
>>> age=18
数据项(May)及其名称(month)具有相同的 id()。18 和 age 的 id() 相同。
>>> id(month)
2167264641264
>>> id(age)
140714055169352
标签是一个标识符。通常被称为变量。Python 变量是一个符号名称,它是一个对象的引用或指针。
Creating Python Variables
Python 变量不需要显式声明来保留内存空间,或者你可以说创建变量。当你给 Python 变量赋值时,会自动创建一个 Python 变量。等号 (=)用于向变量赋值。
在 = 运算符左边的运算对象是变量名,在 = 运算符右边的运算对象是存储在变量中的值。例如 -
Printing Python Variables
一旦我们创建了一个 Python 变量并为其赋值,我们就可以使用 print() 函数打印它。以下是先前示例的扩展,并演示了如何在 Python 中打印不同的变量:
Example to Print Python Variables
此示例打印变量。
counter = 100 # Creates an integer variable
miles = 1000.0 # Creates a floating point variable
name = "Zara Ali" # Creates a string variable
print (counter)
print (miles)
print (name)
这里,分别将 100、1000.0 和“Zara Ali”赋值给 counter、miles 和 name 变量。当运行上述 Python 程序时,将产生以下结果 -
100
1000.0
Zara Ali
Deleting Python Variables
你可以使用 del 语句来删除对数字对象的引用。del 语句的语法为 -
del var1[,var2[,var3[....,varN]]]]
你可以使用 del 语句删除单个对象或多个对象。例如 -
del var
del var_a, var_b
Case-Sensitivity of Python Variables
Python 变量区分大小写,这意味着 Age 和 age 是两个不同的变量:
age = 20
Age = 30
print( "age =", age )
print( "Age =", Age )
这将产生以下结果:
age = 20
Age = 30
Python Variables - Multiple Assignment
Python 允许在单个语句中初始化多个变量。在以下情况下,三个变量具有相同的值。
>>> a=10
>>> b=10
>>> c=10
你可以使用如下的单个赋值语句来代替单独的赋值:
>>> a=b=c=10
>>> print (a,b,c)
10 10 10
在下面的情况下,我们有三个具有不同值得变量。
>>> a=10
>>> b=20
>>> c=30
这些单独的赋值语句可以组合为一个。你可以在 = 运算符的左侧写上用逗号分隔的变量名,在右侧写上用逗号分隔的值。
>>> a,b,c = 10,20,30
>>> print (a,b,c)
10 20 30
我们来试着在脚本模式下使用几个示例:-
a = b = c = 100
print (a)
print (b)
print (c)
这将产生以下结果:
100
100
100
此处,使用值 1 创建了一个整数对象,所有三个变量都被分配到相同的内存位置。你还可以将多个对象分配给多个变量。例如:-
a,b,c = 1,2,"Zara Ali"
print (a)
print (b)
print (c)
这将产生以下结果:
1
2
Zara Ali
此处,两个具有值 1 和 2 的整数对象分别被分配给变量 a 和 b,一个具有值“Zara Ali”的字符串对象被分配给变量 c。
Python Variables - Naming Convention
每个 Python 变量应具有唯一名称,如 a、b、c。变量名称可以有意义,如 color、age、name 等。命名 Python 变量时应注意以下某些规则:
-
变量名称必须以字母或下划线字符开头。
-
变量名称不能以数字或任何特殊字符(如 $、(、* % 等)开头。
-
变量名称只能包含字母数字字符和下划线(A-z、0-9 和 _)。
-
Python 变量名称区分大小写,这意味着 Name 和 NAME 在 Python 中是两个不同的变量。
-
Python 保留关键字不能用于命名变量。
如果变量名包含多个单词,我们应该使用以下命名模式:
-
Camel case - 第一个字母是小写,但每个后续单词的第一个字母是大写。例如:kmPerHour、pricePerLitre
-
Pascal case - 每个单词的第一个字母是大写。例如:KmPerHour、PricePerLitre
-
Snake case - 使用单个下划线(_)字符来分隔单词。例如:km_per_hour、price_per_litre
Example
以下是有效的 Python 变量名称:
counter = 100
_count = 100
name1 = "Zara"
name2 = "Nuha"
Age = 20
zara_salary = 100000
print (counter)
print (_count)
print (name1)
print (name2)
print (Age)
print (zara_salary)
这将产生以下结果:
100
100
Zara
Nuha
20
100000
Example
以下是无效的 Python 变量名称:
1counter = 100
$_count = 100
zara-salary = 100000
print (1counter)
print ($count)
print (zara-salary)
这将产生以下结果:
File "main.py", line 3
1counter = 100
^
SyntaxError: invalid syntax
Example
一旦你使用变量来标识数据项,就可以在其 id() 值不重复的情况下重复使用它。在此情况下,我们有矩形的变量高度和宽度。我们可以使用这些变量来计算面积和周长。
>>> width=10
>>> height=20
>>> area=width*height
>>> area
200
>>> perimeter=2*(width+height)
>>> perimeter
60
在编写脚本或程序时,使用变量特别有利。以下脚本也使用上述变量。
#! /usr/bin/python3
width = 10
height = 20
area = width*height
perimeter = 2*(width+height)
print ("Area = ", area)
print ("Perimeter = ", perimeter)
保存带有 .py 扩展名的上述脚本,并从命令行执行它。结果将为:
Area = 200
Perimeter = 60
Constants in Python
Python 没有任何正式定义的常量,然而你可以使用带有下划线的大写名称来表示一个变量应被视为常量。例如,名称 PI_VALUE 表示你不想重新定义或以任何方式更改变量。
Python vs C/C++ Variables
变量的概念在 Python 中与在 C/C 中的工作方式不同。在 C/C 中,变量是已命名的内存位置。如果 a=10 并且 b=10,它们是两个不同的内存位置。让我们假设它们的内存地址分别是 100 和 200。

如果为“a”指派了不同的值(比如 50),地址 100 中的 10 将被覆盖。
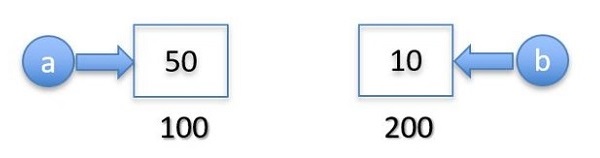
Python 变量指的是对象而不是内存位置。对象只存储在内存中一次。多个变量实际上是同一对象的多个标签。
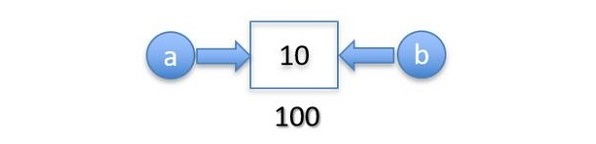
语句 a=50 在内存中的其他位置创建一个新的 int 对象 50,并将对象 10 指向 "b"。
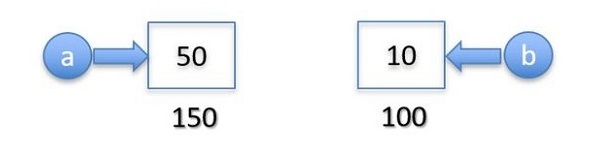
此外,如果你为 b 分配其他值,对象 10 将保持未引用。

Python 的垃圾收集器机制释放了任何未引用对象占用的内存。
如果两个操作数具有相同的 id() 值,Python 的标识运算符 is 返回 True。
>>> a=b=10
>>> a is b
True
>>> id(a), id(b)
(140731955278920, 140731955278920)