Android 简明教程
Android - Bluetooth
在多种方式中,蓝牙是用于在两部不同设备之间发送或接收数据的一种方式。Android 平台包括对蓝牙框架的支持,该框架允许设备通过无线方式与其他蓝牙设备交换数据。
Android 提供蓝牙 API 来执行这些不同操作。
-
扫描其他蓝牙设备
-
获取配对设备列表
-
通过服务发现连接到其他设备
Android 提供了 BluetoothAdapter 类来与蓝牙进行通信。通过调用静态方法 getDefaultAdapter() 来创建此调用的对象。其语法如下所示。
private BluetoothAdapter BA;
BA = BluetoothAdapter.getDefaultAdapter();
为了启用设备的蓝牙功能,请调用带有以下蓝牙常量 ACTION_REQUEST_ENABLE 的 intent。其语法如下。
Intent turnOn = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivityForResult(turnOn, 0);
除了这个常量外,API 还提供了用于支持不同任务的其他常量。它们列在下面。
Sr.No |
Constant & description |
1 |
ACTION_REQUEST_DISCOVERABLE 此常量用于打开蓝牙扫描 |
2 |
ACTION_STATE_CHANGED 此常量将通知蓝牙状态已更改 |
3 |
ACTION_FOUND 此常量用于接收有关已扫描的每个设备的信息 |
在启用蓝牙后,你可以通过调用 getBondedDevices() 方法获取配对设备的列表。它会返回一组蓝牙设备。它的语法为:
private Set<BluetoothDevice>pairedDevices;
pairedDevices = BA.getBondedDevices();
除了配对设备外,API 中还有其他方法可提供对蓝牙的更多控制。它们列在下面。
Sr.No |
Method & description |
1 |
enable() 如果未启用,此方法会启用适配器 |
2 |
isEnabled() 如果适配器启用,此方法会返回 true |
3 |
disable() 此方法禁用适配器 |
4 |
getName() 此方法返回蓝牙适配器的名称 |
5 |
setName(String name) 此方法更改蓝牙名称 |
6 |
getState() 此方法返回蓝牙适配器的当前状态 |
7 |
startDiscovery() 此方法启动蓝牙发现过程 120 秒 |
Example
此示例提供 BluetoothAdapter 类演示,以控制蓝牙并显示蓝牙的配对设备列表。
要尝试此示例,您需要在实际设备上运行此示例。
Steps |
Description |
1 |
你将使用 Android Studio 创建一个 Android 应用程序,即包 com.example.sairamkrishna.myapplication。 |
2 |
修改 src/MainActivity.java 文件以添加代码 |
3 |
修改布局 XML 文件 res/layout/activity_main.xml,如有需要,则添加任何 GUI 组件。 |
4 |
修改 AndroidManifest.xml 以添加必要的权限。 |
5 |
运行该应用程序,选择一个正在运行的安卓设备,并在该设备上安装应用程序并验证结果。 |
以下是 src/MainActivity.java 的内容
package com.example.sairamkrishna.myapplication;
import android.app.Activity;
import android.bluetooth.BluetoothAdapter;
import android.bluetooth.BluetoothDevice;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.ListView;
import android.widget.Toast;
import java.util.ArrayList;
import java.util.Set;
public class MainActivity extends Activity {
Button b1,b2,b3,b4;
private BluetoothAdapter BA;
private Set<BluetoothDevice>pairedDevices;
ListView lv;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
b1 = (Button) findViewById(R.id.button);
b2=(Button)findViewById(R.id.button2);
b3=(Button)findViewById(R.id.button3);
b4=(Button)findViewById(R.id.button4);
BA = BluetoothAdapter.getDefaultAdapter();
lv = (ListView)findViewById(R.id.listView);
}
public void on(View v){
if (!BA.isEnabled()) {
Intent turnOn = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivityForResult(turnOn, 0);
Toast.makeText(getApplicationContext(), "Turned on",Toast.LENGTH_LONG).show();
} else {
Toast.makeText(getApplicationContext(), "Already on", Toast.LENGTH_LONG).show();
}
}
public void off(View v){
BA.disable();
Toast.makeText(getApplicationContext(), "Turned off" ,Toast.LENGTH_LONG).show();
}
public void visible(View v){
Intent getVisible = new Intent(BluetoothAdapter.ACTION_REQUEST_DISCOVERABLE);
startActivityForResult(getVisible, 0);
}
public void list(View v){
pairedDevices = BA.getBondedDevices();
ArrayList list = new ArrayList();
for(BluetoothDevice bt : pairedDevices) list.add(bt.getName());
Toast.makeText(getApplicationContext(), "Showing Paired Devices",Toast.LENGTH_SHORT).show();
final ArrayAdapter adapter = new ArrayAdapter(this,android.R.layout.simple_list_item_1, list);
lv.setAdapter(adapter);
}
}
以下是 activity_main.xml 的内容。
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:paddingBottom="@dimen/activity_vertical_margin"
tools:context=".MainActivity"
android:transitionGroup="true">
<TextView android:text="Bluetooth Example"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/textview"
android:textSize="35dp"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Tutorials point"
android:id="@+id/textView"
android:layout_below="@+id/textview"
android:layout_centerHorizontal="true"
android:textColor="#ff7aff24"
android:textSize="35dp" />
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/imageView"
android:src="@drawable/abc"
android:layout_below="@+id/textView"
android:layout_centerHorizontal="true"
android:theme="@style/Base.TextAppearance.AppCompat" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Turn On"
android:id="@+id/button"
android:layout_below="@+id/imageView"
android:layout_toStartOf="@+id/imageView"
android:layout_toLeftOf="@+id/imageView"
android:clickable="true"
android:onClick="on" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Get visible"
android:onClick="visible"
android:id="@+id/button2"
android:layout_alignBottom="@+id/button"
android:layout_centerHorizontal="true" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="List devices"
android:onClick="list"
android:id="@+id/button3"
android:layout_below="@+id/imageView"
android:layout_toRightOf="@+id/imageView"
android:layout_toEndOf="@+id/imageView" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="turn off"
android:onClick="off"
android:id="@+id/button4"
android:layout_below="@+id/button"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true" />
<ListView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/listView"
android:layout_alignParentBottom="true"
android:layout_alignLeft="@+id/button"
android:layout_alignStart="@+id/button"
android:layout_below="@+id/textView2" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Paired devices:"
android:id="@+id/textView2"
android:textColor="#ff34ff06"
android:textSize="25dp"
android:layout_below="@+id/button4"
android:layout_alignLeft="@+id/listView"
android:layout_alignStart="@+id/listView" />
</RelativeLayout>
以下是 Strings.xml 的内容
<resources>
<string name="app_name">My Application</string>
</resources>
以下是 AndroidManifest.xml 的内容
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.sairamkrishna.myapplication" >
<uses-permission android:name="android.permission.BLUETOOTH"/>
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name=".MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
我们尝试运行你的应用程序。我假设你已将真实的 Android 手机连接到你的计算机。要从 Android Studio 运行该应用,请打开项目中的一个活动文件,然后从工具栏中单击运行图标。如果你的蓝牙未打开,它将要求你允许启用蓝牙。
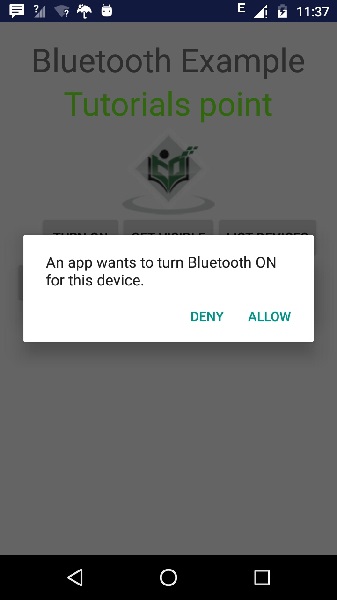
现在,只需选择“获得可见性”按钮即可打开你的可见性。会出现以下屏幕,要求你允许打开发现 120 秒。

现在,只需选择“列出设备”选项。它将在列表视图中列出配对的设备。在我的情况下,我只有一台配对设备。如下所示。

现在只需选择关闭按钮即可关闭蓝牙。关闭蓝牙后,将出现以下消息,指示蓝牙已成功关闭。
