Android 简明教程
Android - Twitter Integration
Android 允许您的应用程序连接到 Twitter 并分享数据或任何种类的 Twitter 更新。本章介绍将 Twitter 集成到您的应用程序。
Android allows your application to connect to twitter and share data or any kind of updates on twitter. This chapter is about integrating twitter into your application.
可以通过两种方式集成 Twitter 并在您的应用程序上分享内容。这些方式列在下面 −
There are two ways through which you can integrate twitter and share something from your application. These ways are listed below −
-
Twitter SDK (Twitter4J)
-
Intent Share
Integrating Twitter SDK
这是第一种与 Twitter 连接的方式。您必须注册您的应用程序,然后接收一些应用程序 ID,然后您必须下载 Twitter SDK 并将它添加到您的项目。步骤如下 −
This is the first way of connecting with Twitter. You have to register your application and then receive some Application Id, and then you have to download the twitter SDK and add it to your project. The steps are listed below −
Registering your application
在 dev.twitter.com/apps/new 创建一个新的 Twitter 应用程序,并填写所有信息。如下所示 −
Create a new twitter application at dev.twitter.com/apps/new and fill all the information. It is shown below −
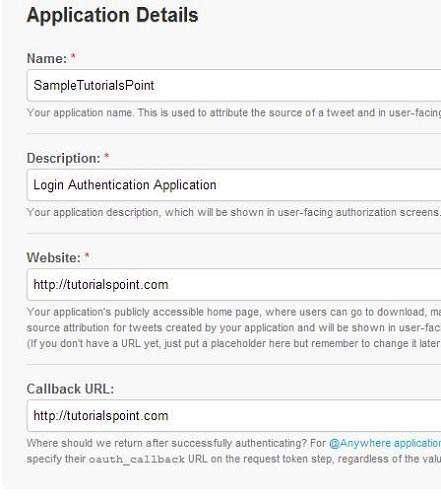
现在在设置标签页下,将访问权限更改为读写并访问消息,并保存设置。如下所示 −
Now under settings tab, change the access to read,write and access messages and save the settings. It is shown below −
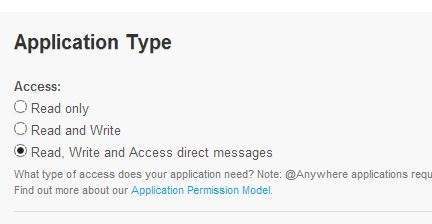
如果一切正常,您将收到一个带有密码的消费者 ID。只需复制应用程序 ID,并将它保存在某个地方。在下图中显示 −
If everything works fine, you will receive an consumer ID with the secret. Just copy the application id and save it somewhere. It is shown in the image below −
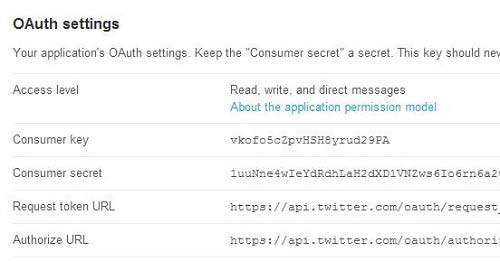
Downloading SDK and integrating it
下载 Twitter SDK here 。将 twitter4J jar 复制到您的项目 libs 文件夹中。
Download twitter sdk here. Copy the twitter4J jar into your project libs folder.
Posting tweets on twitter application
一旦所有内容都已完成,您可以运行可以在 here 中找到的 twitter 4J 示例。
Once everything is complete , you can run the twitter 4J samples which can be found here.
要使用 Twitter,您需要实例化 Twitter 类的对象。可以通过调用静态方法 getsingleton() 来实现。其语法如下。
In order to use twitter, you need to instantiate an object of twitter class.It can be done by calling the static method getsingleton(). Its syntax is given below.
// The factory instance is re-usable and thread safe.
Twitter twitter = TwitterFactory.getSingleton();
要更新状态,您可以调用 updateStatus() 方法。其语法如下 −
In order to update the status , you can call updateStatus() method. Its syntax is given below −
Status status = twitter.updateStatus(latestStatus);
System.out.println("Successfully updated the status to [" + status.getText() + "].");
Intent share
意图共享用于在应用程序之间共享数据。使用此策略,我们不会处理 SDK 事务,而是让 Twitter 应用程序处理。我们只会调用 Twitter 应用程序并将数据传递以供分享。通过此方式,我们可以通过 Twitter 分享一些内容。
Intent share is used to share data between applications. In this strategy, we will not handle the SDK stuff, but let the twitter application handles it. We will simply call the twitter application and pass the data to share. This way, we can share something on twitter.
Android 提供意图库,以在活动和应用程序之间共享数据。要将此作为共享意图使用,我们必须指定共享意图的类型为 ACTION_SEND 。其语法如下 −
Android provides intent library to share data between activities and applications. In order to use it as share intent, we have to specify the type of the share intent to ACTION_SEND. Its syntax is given below −
Intent shareIntent = new Intent();
shareIntent.setAction(Intent.ACTION_SEND);
接下来您需要做的是定义要传递的数据的类型,然后传递数据。其语法如下 −
Next thing you need to is to define the type of data to pass, and then pass the data. Its syntax is given below −
shareIntent.setType("text/plain");
shareIntent.putExtra(Intent.EXTRA_TEXT, "Hello, from tutorialspoint");
startActivity(Intent.createChooser(shareIntent, "Share your thoughts"));
除了这些方法,还有其他允许意图处理的方法。它们列在下面 −
Apart from the these methods, there are other methods available that allows intent handling. They are listed below −
Sr.No |
Method & description |
1 |
addCategory(String category) This method add a new category to the intent. |
2 |
createChooser(Intent target, CharSequence title) Convenience function for creating a ACTION_CHOOSER Intent |
3 |
getAction() This method retrieve the general action to be performed, such as ACTION_VIEW |
4 |
getCategories() This method return the set of all categories in the intent and the current scaling event |
5 |
putExtra(String name, int value) This method add extended data to the intent. |
6 |
toString() This method returns a string containing a concise, human-readable description of this object |
Example
以下是一个使用 IntentShare 在 twitter 上分享数据的示例。它创建一个基本的应用程序,允许你在 twitter 上分享一些文本。
Here is an example demonstrating the use of IntentShare to share data on twitter. It creates a basic application that allows you to share some text on twitter.
要使用此示例,你可以在实际设备或模拟器上运行此示例。
To experiment with this example , you can run this on an actual device or in an emulator.
Steps |
Description |
1 |
You will use Android studio to create an Android application under a package com.example.sairamkrishna.myapplication. |
2 |
Modify src/MainActivity.java file to add necessary code. |
3 |
Modify the res/layout/activity_main to add respective XML components |
4 |
Run the application and choose a running android device and install the application on it and verify the results |
下面是已修改的 MainActivity.java 的内容。
Following is the content of the modified MainActivity.java.
package com.example.sairamkrishna.myapplication;
import android.content.Intent;
import android.net.Uri;
import android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
import java.io.FileNotFoundException;
import java.io.InputStream;
public class MainActivity extends ActionBarActivity {
private ImageView img;
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
img=(ImageView)findViewById(R.id.imageView);
Button b1=(Button)findViewById(R.id.button);
b1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent sharingIntent = new Intent(Intent.ACTION_SEND);
Uri screenshotUri = Uri.parse("android.resource://comexample.sairamkrishna.myapplication/*");
try {
InputStream stream = getContentResolver().openInputStream(screenshotUri);
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
sharingIntent.setType("image/jpeg");
sharingIntent.putExtra(Intent.EXTRA_STREAM, screenshotUri);
startActivity(Intent.createChooser(sharingIntent, "Share image using"));
}
});
}
}
以下是修改后的 xml res/layout/activity_main.xml 内容。
Following is the modified content of the xml res/layout/activity_main.xml.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/textView"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:textSize="30dp"
android:text="Twitter share " />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Tutorials Point"
android:id="@+id/textView2"
android:layout_below="@+id/textView"
android:layout_centerHorizontal="true"
android:textSize="35dp"
android:textColor="#ff16ff01" />
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/imageView"
android:layout_below="@+id/textView2"
android:layout_centerHorizontal="true"
android:src="@drawable/abc"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Share"
android:id="@+id/button"
android:layout_marginTop="61dp"
android:layout_below="@+id/imageView"
android:layout_centerHorizontal="true" />
</RelativeLayout>
下面是 AndroidManifest.xml 文件的内容。
Following is the content of AndroidManifest.xml file.
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.sairamkrishna.myapplication" >
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name=".MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
让我们尝试运行你的应用程序。我假设你已将你的安卓移动设备与电脑连接。若要从 Android 工作室运行该应用程序,请打开你的一个项目的活动文件,然后点击工具栏中的“运行”图标。在启动应用程序之前,Android 工作室会显示以下窗口,以选择要在其中运行安卓应用程序的选项。
Let’s try to run your application. I assume you have connected your actual Android Mobile device with your computer. To run the app from Android studio, open one of your project’s activity files and click Run icon from the toolbar. Before starting your application,Android studio will display following window to select an option where you want to run your Android application.

选择您的移动设备作为选项,然后检查您的移动设备,它将显示您的默认屏幕 −
Select your mobile device as an option and then check your mobile device which will display your default screen −
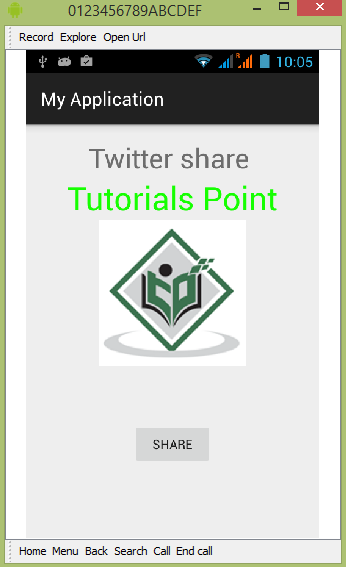
现在,轻触该按钮,你便会看到一个分享提供程序列表。
Now just tap on the button and you will see a list of share providers.
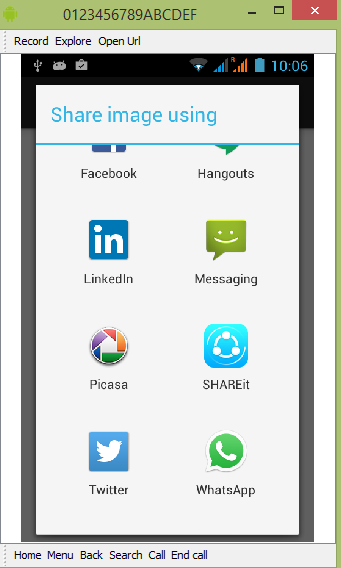
现在,从该列表中选择 twitter,然后写一条消息。如下面的图片所示:
Now just select twitter from that list and then write any message. It is shown in the image below −
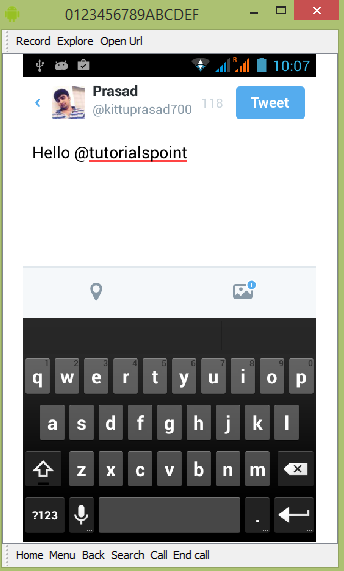
现在,选择 tweet 按钮,然后在你的 twitter 页面上发布。如下所示:
Now just select the tweet button and then it would be post on your twitter page. It is shown below −
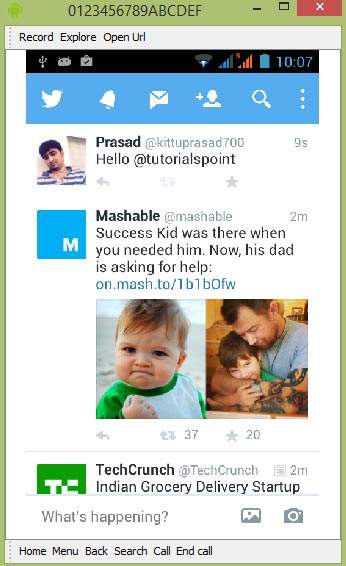