Android 简明教程
Android - Testing
Android 框架包括一个集成的测试框架,可帮助您测试应用程序的所有方面,而 SDK 工具包含用于设置和运行测试应用程序的工具。无论是使用带 ADT 的 Eclipse 工作还是从命令行工作,SDK 工具均可帮助您在模拟器或目标设备内设置和运行测试。
Testing Tools in android
有很多可用于测试 Android 应用程序的工具。有些是正式工具,如 JUnit 和 Monkey,而另一些是可用于测试 Android 应用程序的第三方工具。在本章中,我们将讲解这两种用于测试 Android 应用程序的工具。
-
JUnit
-
Monkey
JUnit
您可以使用 JUnit TestCase 类对不调用 Android API 的类执行单元测试。TestCase 也是 AndroidTestCase 的基类,您可以使用它来测试依赖 Android 的对象。除了提供 JUnit 框架外,AndroidTestCase 还提供与 Android 相关的设置、终止和帮助器方法。
为了使用 TestCase,请将您的类扩展为 TestCase 类并实现一个名为 setUp() 的方法调用。其语法如下所示:
public class MathTest extends TestCase {
protected double fValue1;
protected double fValue2;
protected void setUp() {
fValue1= 2.0;
fValue2= 3.0;
}
}
对于每个测试,实现一个与固定装置交互的方法。使用 assertTrue(String, boolean) 调用一个布尔值来验证预期结果与断言相符。
public void testAdd() {
double result= fValue1 + fValue2;
assertTrue(result == 5.0);
}
assert 方法将您预期从测试中得到的值与实际结果进行比较,如果比较失败,则抛出一个异常。
在定义方法后,您可以运行它们。其语法如下所示:
TestCase test= new MathTest("testAdd");
test.run();
Monkey
UI/应用程序演练器 Monkey(通常称为“monkey”)是一个命令行工具,它向设备发送伪随机序列的按键、触摸和手势。您可以使用 Android 调试桥 (adb) 工具运行它。
使用它来对您的应用程序进行压力测试并报告遇到的错误。您可以通过每次使用相同的随机数种子运行该工具来重复一系列事件。
Monkey features
Monkey 有很多功能,但都可以归纳为以下四类。
-
Basic configuration options
-
Operational constraints
-
Event types and frequencies
-
Debugging options
Monkey Usage
为了使用 monkey,请打开命令提示符,然后导航到以下目录。
android ->sdk ->platform-tools
进入该目录后,使用 PC 连接您的设备,然后运行以下命令。
adb shell monkey -p your.package.name -v 500
可以将此命令分解为以下步骤。
-
adb - Android 调试桥。一种用于从台式机或笔记本电脑连接和向 Android 手机发送命令的工具。
-
shell - shell 只是设备上将我们的命令转换为系统命令的一个接口。
-
monkey - monkey 是测试工具。
-
v - v 代表详细方法。
-
500- 是发送来进行测试的频率计数,或事件数量。
这一点还显示在图 − 中

在上述命令中,您在默认安卓 UI 应用程序上运行 monkey 工具。现在,为了在您的应用程序中运行它,您需要执行以下操作。
最后,您将完成,如下所示
这一点还显示在下面的图中。通过输入该命令,实际上您正在生成 500 个随机事件来进行测试。
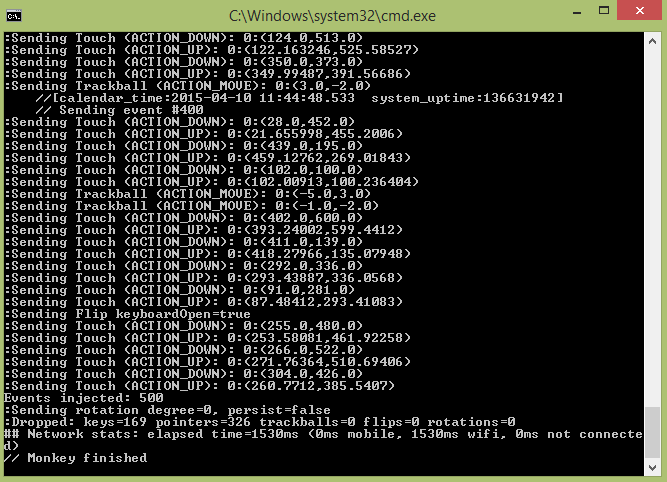
Example
下面的示例演示了使用 Testing 的方法。它生成了一个可用于 Monkey 的基本应用程序。
要尝试此示例,您需要在实际设备上运行此示例,然后按照开始中说明的 Monkey 步骤进行操作。
Steps |
Description |
1 |
您将使用 Android Studio 在包 com.tutorialspoint.myapplication 下创建一个 Android 应用程序。 |
2 |
修改 src/MainActivity.java 文件以添加 Activity 代码。 |
3 |
修改布局 XML 文件 res/layout/activity_main.xml,添加任何所需的 GUI 组件。 |
4 |
创建一个 src/second.java 文件来添加 Activity 代码。 |
5 |
修改布局 XML 文件 res/layout/view.xml,如果需要,则添加任何 GUI 组件。 |
6 |
运行该应用程序,选择一个正在运行的安卓设备,并在该设备上安装应用程序并验证结果。 |
以下是 MainActivity.java 的内容。
package com.tutorialspoint.myapplication;
import android.content.Intent;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.Button;
public class MainActivity extends AppCompatActivity {
Button b1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
b1=(Button)findViewById(R.id.button);
}
public void button(View v){
Intent in =new Intent(MainActivity.this,second.class);
startActivity(in);
}
}
以下是 second.java 的内容。
package com.tutorialspoint.myapplication;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
public class second extends Activity{
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.view);
Button b1=(Button)findViewById(R.id.button2);
b1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(second.this,"Thanks",Toast.LENGTH_SHORT).show();
}
});
}
}
以下是 activity_main.xml 的内容。
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent"
android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="UI Animator Viewer"
android:id="@+id/textView"
android:textSize="25sp"
android:layout_centerHorizontal="true" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Tutorials point"
android:id="@+id/textView2"
android:layout_below="@+id/textView"
android:layout_alignRight="@+id/textView"
android:layout_alignEnd="@+id/textView"
android:textColor="#ff36ff15"
android:textIsSelectable="false"
android:textSize="35dp" />
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/imageView"
android:src="@drawable/abc"
android:layout_below="@+id/textView2"
android:layout_centerHorizontal="true" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button"
android:onClick="button"
android:id="@+id/button"
android:layout_below="@+id/imageView"
android:layout_centerHorizontal="true"
android:layout_marginTop="100dp" />
</RelativeLayout>
以下是 view.xml 的内容
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent" android:layout_height="match_parent">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="button"
android:id="@+id/button2"
android:layout_centerVertical="true"
android:layout_centerHorizontal="true" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Tutorials point "
android:id="@+id/textView3"
android:textColor="#ff3aff22"
android:textSize="35dp"
android:layout_above="@+id/button2"
android:layout_centerHorizontal="true"
android:layout_marginBottom="90dp" />
</RelativeLayout>
以下是 Strings.xml 的内容。
<resources>
<string name="app_name">My Application</string>
</resources>
以下是 AndroidManifest.xml 的内容。
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.tutorialspoint.myapplication" >
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name=".MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<activity android:name=".second"></activity>
</application>
</manifest>
让我们尝试运行您的 Android Testing 应用程序。我假设您已将实际 Android 移动设备连接到计算机。要从 Android Studio 运行该应用程序,请打开您项目的其中一个活动文件,然后从工具栏中单击“Run”图标。在启动您的应用程序之前,Android Studio 将显示以下窗口,让您选择想要运行 Android 应用程序的位置。

选择您的移动设备作为选项,然后检查会显示应用程序屏幕的移动设备。现在,只需按照 Monkey 部分顶部的步骤操作,即可对该应用程序执行测试。