Javafx 简明教程
JavaFX - Stacked Bar Chart
堆叠条形图是条形图的变体,其中绘制表示某个类别的条形状的数据值。根据哪个轴是类别轴的不同,条形可以是垂直的或水平的。每个序列的条形叠放在前一个序列的顶部。
StackedBarChart is a variation of a BarChart, which plots bars indicating data values for a category. The bars can be vertical or horizontal depending on which axis is the category axis. The bar for each series is stacked on top of the previous series.
以下是表示人口增长的堆叠条形图。
The following is a Stacked Bar Chart, which depicts the population growth.
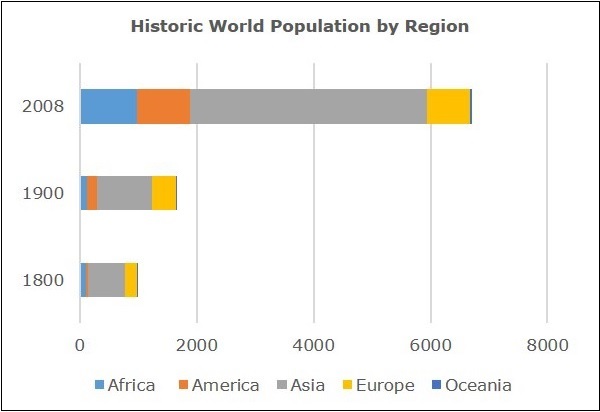
Stacked Bar Chart in JavaFX
在 JavaFX 中,堆叠条形图由名为 StackedBarChart 的类表示。此类属于 javafx.scene.chart 包。通过实例化此类,可以在 JavaFX 中创建堆叠条形图节点。
In JavaFX, a Stacked Bar Chart is represented by a class named StackedBarChart. This class belongs to the package javafx.scene.chart. By instantiating this class, you can create a StackedBarChart node in JavaFX.
若要在 JavaFX 中生成堆叠条形图,请按照下面给出的步骤操作。
To generate a Stacked Bar Chart in JavaFX, follow the steps given below.
Step 1: Defining the Axis
定义堆叠条形图的 X 轴和 Y 轴并向它们设置标签。在我们的示例中,X 轴代表大洲,y 轴用百万表示人口。
Define the X and Y axis of the stacked bar chart and set labels to them. In our example, X axis represents the continents and the y axis represents the population in millions.
public class ClassName extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
//Defining the x axis
CategoryAxis xAxis = new CategoryAxis();
xAxis.setCategories(FXCollections.<String>observableArrayList(Arrays.asList
("Africa", "America", "Asia", "Europe", "Oceania")));
xAxis.setLabel("category");
//Defining the y axis
NumberAxis yAxis = new NumberAxis();
yAxis.setLabel("Population (In millions)");
}
}
Step 2: Creating the Stacked Bar Chart
通过实例化包 javafx.scene.chart 的名为 StackedBarChart 的类创建折线图。将表示在上一步中创建的 X 轴和 Y 轴的对象传递给此类的构造函数。
Create a line chart by instantiating the class named StackedBarChart of the package javafx.scene.chart. To the constructor of this class, pass the objects representing the X and Y axis created in the previous step.
//Creating the Bar chart
StackedBarChart<String, Number> stackedBarChart =
new StackedBarChart<>(xAxis, yAxis);
stackedBarChart.setTitle("Historic World Population by Region");
Step 3: Preparing the Data
实例化 XYChart.Series 类并将数据(系列、x 和 y 坐标)添加到此类的可观察列表中,如下所示 −
Instantiate the XYChart.Series class and add the data (a series of, x and y coordinates) to the Observable list of this class as follows −
//Prepare XYChart.Series objects by setting data
XYChart.Series<String, Number> series1 = new XYChart.Series<>();
series1.setName("1800");
series1.getData().add(new XYChart.Data<>("Africa", 107));
series1.getData().add(new XYChart.Data<>("America", 31));
series1.getData().add(new XYChart.Data<>("Asia", 635));
series1.getData().add(new XYChart.Data<>("Europe", 203));
series1.getData().add(new XYChart.Data<>("Oceania", 2));
XYChart.Series<String, Number> series2 = new XYChart.Series<>();
series2.setName("1900");
series2.getData().add(new XYChart.Data<>("Africa", 133));
series2.getData().add(new XYChart.Data<>("America", 156));
series2.getData().add(new XYChart.Data<>("Asia", 947));
series2.getData().add(new XYChart.Data<>("Europe", 408));
series1.getData().add(new XYChart.Data<>("Oceania", 6));
XYChart.Series<String, Number> series3 = new XYChart.Series<>();
series3.setName("2008");
series3.getData().add(new XYChart.Data<>("Africa", 973));
series3.getData().add(new XYChart.Data<>("America", 914));
series3.getData().add(new XYChart.Data<>("Asia", 4054));
series3.getData().add(new XYChart.Data<>("Europe", 732));
series1.getData().add(new XYChart.Data<>("Oceania", 34));
Step 4: Add Data to the Stacked Bar Chart
将上一步中准备的数据系列添加到条形图,如下所示 −
Add the data series prepared in the previous step to the bar chart as follows −
//Setting the data to bar chart
stackedBarChart.getData().addAll(series1, series2, series3);
Step 5: Creating a Group Object
在 start() 方法中,通过实例化名为 Group 的类创建组对象。这属于包 javafx.scene 。
In the start() method, create a group object by instantiating the class named Group. This belongs to the package javafx.scene.
将上一步中创建的堆叠条形图(节点)对象作为参数传递给组类的构造函数。应该这样做以将它添加到组中,如下所示 −
Pass the StackedBarChart (node) object created in the previous step as a parameter to the constructor of the Group class. This should be done in order to add it to the group as follows −
Group root = new Group(stackedBarChart);
Step 6: Launching Application
最后,按照下面给出的步骤正确启动应用程序 −
Lastly, follow the given steps below to launch the application properly −
-
Firstly, instantiate the class named Scene by passing the Group object as a parameter value to its constructor. To this constructor, you can also pass dimensions of the application screen as optional parameters.
-
Then, set the title to the stage using the setTitle() method of the Stage class.
-
Now, a Scene object is added to the stage using the setScene() method of the class named Stage.
-
Display the contents of the scene using the method named show().
-
Lastly, the application is launched with the help of the launch() method.
Example
下表列出了 1800 年、1900 年和 2008 年不同大陆的人口。
The following table lists out the population in various continents in the years 1800, 1900 and 2008.
Africa |
America |
Asia |
Europe |
Oceania |
|
1800 |
107 |
31 |
635 |
203 |
2 |
1900 |
133 |
156 |
947 |
408 |
6 |
2008 |
973 |
914 |
4054 |
732 |
34 |
以下是使用 JavaFX 生成表示上述数据的堆叠条形图的 Java 程序。
Following is a Java program that generates a stacked bar chart depicting the above data, using JavaFX.
将此代码保存在名为 StackedBarChartExample.java 的文件中。
Save this code in a file with the name StackedBarChartExample.java.
import java.util.Arrays;
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.chart.CategoryAxis;
import javafx.stage.Stage;
import javafx.scene.chart.NumberAxis;
import javafx.scene.chart.StackedBarChart;
import javafx.scene.chart.XYChart;
public class StackedBarChartExample extends Application {
@Override
public void start(Stage stage) {
//Defining the axes
CategoryAxis xAxis = new CategoryAxis();
xAxis.setCategories(FXCollections.<String>observableArrayList(Arrays.asList
("Africa", "America", "Asia", "Europe", "Oceania")));
xAxis.setLabel("category");
NumberAxis yAxis = new NumberAxis();
yAxis.setLabel("Population (In millions)");
//Creating the Bar chart
StackedBarChart<String, Number> stackedBarChart =
new StackedBarChart<>(xAxis, yAxis);
stackedBarChart.setTitle("Historic World Population by Region");
//Prepare XYChart.Series objects by setting data
XYChart.Series<String, Number> series1 = new XYChart.Series<>();
series1.setName("1800");
series1.getData().add(new XYChart.Data<>("Africa", 107));
series1.getData().add(new XYChart.Data<>("America", 31));
series1.getData().add(new XYChart.Data<>("Asia", 635));
series1.getData().add(new XYChart.Data<>("Europe", 203));
series1.getData().add(new XYChart.Data<>("Oceania", 2));
XYChart.Series<String, Number> series2 = new XYChart.Series<>();
series2.setName("1900");
series2.getData().add(new XYChart.Data<>("Africa", 133));
series2.getData().add(new XYChart.Data<>("America", 156));
series2.getData().add(new XYChart.Data<>("Asia", 947));
series2.getData().add(new XYChart.Data<>("Europe", 408));
series1.getData().add(new XYChart.Data<>("Oceania", 6));
XYChart.Series<String, Number> series3 = new XYChart.Series<>();
series3.setName("2008");
series3.getData().add(new XYChart.Data<>("Africa", 973));
series3.getData().add(new XYChart.Data<>("America", 914));
series3.getData().add(new XYChart.Data<>("Asia", 4054));
series3.getData().add(new XYChart.Data<>("Europe", 732));
series1.getData().add(new XYChart.Data<>("Oceania", 34));
//Setting the data to bar chart
stackedBarChart.getData().addAll(series1, series2, series3);
//Creating a Group object
Group root = new Group(stackedBarChart);
//Creating a scene object
Scene scene = new Scene(root, 600, 400);
//Setting title to the Stage
stage.setTitle("stackedBarChart");
//Adding scene to the stage
stage.setScene(scene);
//Displaying the contents of the stage
stage.show();
}
public static void main(String args[]){
launch(args);
}
}
使用以下命令,从命令提示符编译并执行已保存的 java 文件。
Compile and execute the saved java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls StackedBarChartExample.java
java --module-path %PATH_TO_FX% --add-modules javafx.controls StackedBarChartExample
执行后,上述程序会生成一个 JavaFX 窗口,显示如下所示的区域图。
On executing, the above program generates a JavaFX window displaying an area chart as shown below.
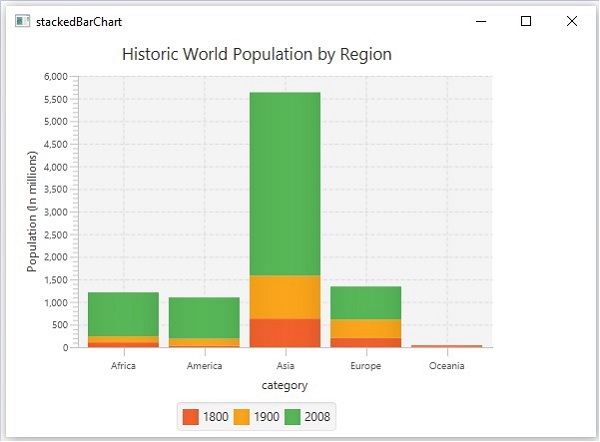
Example
下表描述了某组织在一年第一季度解雇和招募员工的数据。
The following table depicts the data of employees laid off and recruited in an organization, in the first quarter of an year.
Month |
Employees Laid Off |
Employees Recruited |
January |
30 |
12 |
February |
12 |
54 |
March |
31 |
24 |
April |
52 |
32 |
May |
4 |
43 |
June |
10 |
5 |
以下是使用 JavaFX 生成表示上述数据的堆叠条形图的 Java 程序。
Following is a Java program that generates a stacked bar chart depicting the above data, using JavaFX.
将此代码保存在名为 StackedBarChartEmployees.java 的文件中。
Save this code in a file with the name StackedBarChartEmployees.java.
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.chart.StackedBarChart;
import javafx.scene.chart.CategoryAxis;
import javafx.stage.Stage;
import javafx.scene.chart.NumberAxis;
import javafx.scene.chart.XYChart;
public class StackedBarChartEmployees extends Application {
@Override
public void start(Stage stage) {
//Defining the X axis
CategoryAxis xAxis = new CategoryAxis();
//defining the y Axis
NumberAxis yAxis = new NumberAxis();
yAxis.setLabel("Number of Employees");
//Creating the Area chart
StackedBarChart stackedbarChart = new StackedBarChart(xAxis, yAxis);
stackedbarChart.setTitle("Employees Recruitment to Lay off ratio");
//Prepare XYChart.Series objects by setting data
XYChart.Series series1 = new XYChart.Series();
series1.setName("Employees Laid Off");
series1.getData().add(new XYChart.Data("January", 30));
series1.getData().add(new XYChart.Data("February", 12));
series1.getData().add(new XYChart.Data("March", 31));
series1.getData().add(new XYChart.Data("April", 52));
series1.getData().add(new XYChart.Data("May", 4));
series1.getData().add(new XYChart.Data("June", 10));
XYChart.Series series2 = new XYChart.Series();
series2.setName("Employees Recruited");
series2.getData().add(new XYChart.Data("January", 12));
series2.getData().add(new XYChart.Data("February", 54));
series2.getData().add(new XYChart.Data("March", 24));
series2.getData().add(new XYChart.Data("April", 32));
series2.getData().add(new XYChart.Data("May", 43));
series2.getData().add(new XYChart.Data("June", 5));
//Setting the XYChart.Series objects to stacked bar chart
stackedbarChart.getData().addAll(series1,series2);
//Creating a Group object
Group root = new Group(stackedbarChart);
//Creating a scene object
Scene scene = new Scene(root, 600, 400);
//Setting title to the Stage
stage.setTitle("Stacked Bar Chart");
//Adding scene to the stage
stage.setScene(scene);
//Displaying the contents of the stage
stage.show();
}
public static void main(String args[]){
launch(args);
}
}