Javafx 简明教程
JavaFX - Drawing a Rectangle
一般来说,矩形是一种四边形,具有两对平行且同向的边,所有内角都是直角。
它由两个参数描述,即−
-
height − 矩形的垂直长度称为高度。
-
width − 矩形的水平长度称为宽度。

Rectangle in JavaFX
在 JavaFX 中,矩形由名为 Rectangle 的类表示。此类属于 javafx.scene.shape 包。
通过实例化此类,您可以在 JavaFX 中创建一个矩形节点。
此类具有 4 个双数据类型的属性,即 −
-
X − 矩形的开始点(左上)的 x 坐标。
-
Y − 矩形的开始点(左上)的 y 坐标。
-
Width − 矩形的宽度。
-
height – 矩形的高度。
要绘制一个矩形,需要将值传递给这些属性,方法是在实例化时将这些值传递给此类的构造函数,并且按照相同的顺序。
Steps to Draw a Rectangle
需要按照下面给出的步骤在 JavaFX 中绘制一个矩形。
Step 1: Creating a Rectangle
要在 JavaFX 中创建一个矩形,将包 javafx.scene.shape 中名为 Rectangle 的类的实例化,如下所示 −
//Creating a rectangle object
Rectangle rectangle = new Rectangle();
由于整个代码都是写在 Application 类的 start() 方法中,所以 Rectangle 类也在其中实例化,如下所示 −
public class ClassName extends Application {
public void start(Stage primaryStage) throws Exception {
// Write code here
Rectangle rectangle = new Rectangle();
}
}
Step 2: Setting Properties to the Rectangle
指定要绘制的矩形的起点 (左上角) 的 x、y 坐标、高度和宽度。可以通过分别使用它们的setter方法设置 x、y、height 和 width 属性来执行此操作。
rectangle.setX(150.0f);
rectangle.setY(75.0f);
rectangle.setWidth(300.0f);
rectangle.setHeight(150.0f);
Step 3: Adding Rectangle Object to Group
在此步骤中,将包 javafx.scene 中的 Group 类通过将 Rectangle 对象作为其构造函数的参数值传递来实例化,如下所示 −
Group root = new Group(rectangle);
Step 4: Launching Application
创建二维对象后,请按照下面给出的步骤正确启动应用程序:
-
首先,通过将组对象作为其构造函数的参数值传递来实例化名为 Scene 的类。你也可以将应用程序屏幕的尺寸作为可选参数传递给此构造函数。
-
然后,使用 Stage 类的 setTitle() 方法设置阶段标题。
-
现在,使用名为 Stage 的类的 setScene() 方法将 Scene 对象添加到阶段。
-
使用名为 show() 的方法显示场景的内容。
-
最后,借助 launch() 方法启动应用程序。
Example
以下是生成一个矩形的 JavaFX 程序。将此代码保存在一个名为 RectangleExample.java 的文件中。
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.stage.Stage;
import javafx.scene.shape.Rectangle;
public class RectangleExample extends Application {
@Override
public void start(Stage stage) {
//Drawing a Rectangle
Rectangle rectangle = new Rectangle();
//Setting the properties of the rectangle
rectangle.setX(150.0f);
rectangle.setY(75.0f);
rectangle.setWidth(300.0f);
rectangle.setHeight(150.0f);
//Creating a Group object
Group root = new Group(rectangle);
//Creating a scene object
Scene scene = new Scene(root, 600, 300);
//Setting title to the Stage
stage.setTitle("Drawing a Rectangle");
//Adding scene to the stage
stage.setScene(scene);
//Displaying the contents of the stage
stage.show();
}
public static void main(String args[]){
launch(args);
}
}
使用以下命令,从命令提示符编译并执行已保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls RectangleExample.java
java --module-path %PATH_TO_FX% --add-modules javafx.controls RectangleExample
执行时,上述程序将生成一个 JavaFX 窗口,显示一个矩形,如下所示的屏幕截图。

Example - Drawing a House
让我们看另一个尝试使用矩形绘制房子的示例。在此,我们使用两个矩形和两条线完成卡通房屋的基本版本。我们将命名此文件为 RectangleHouse.java 。
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.stage.Stage;
import javafx.scene.shape.Rectangle;
import javafx.scene.shape.Line;
import javafx.scene.paint.Color;
public class RectangleHouse extends Application {
@Override
public void start(Stage stage) {
//Drawing a Rectangle
Rectangle rectangle1 = new Rectangle();
Rectangle rectangle2 = new Rectangle(200.0f, 120.0f, 50.0f, 30.0f);
rectangle2.setFill(Color.hsb(50, 1, 1));
//Setting the properties of the rectangle
rectangle1.setX(150.0f);
rectangle1.setY(75.0f);
rectangle1.setWidth(150.0f);
rectangle1.setHeight(75.0f);
// Setting the properties of Lines
// without setter methods
Line line1 = new Line(150, 75, 225, 30);
Line line2 = new Line(225, 30, 300, 75);
//Creating a Group object
Group root = new Group();
root.getChildren().addAll(rectangle1, rectangle2, line1, line2);
//Creating a scene object
Scene scene = new Scene(root, 600, 300);
//Setting title to the Stage
stage.setTitle("Drawing a House");
//Adding scene to the stage
stage.setScene(scene);
//Displaying the contents of the stage
stage.show();
}
public static void main(String args[]){
launch(args);
}
}
使用以下命令,从命令提示符编译并执行已保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls RectangleHouse.java
java --module-path %PATH_TO_FX% --add-modules javafx.controls RectangleHouse
执行时,上述程序将生成一个 JavaFX 窗口,显示一个房子,如下所示的屏幕截图。
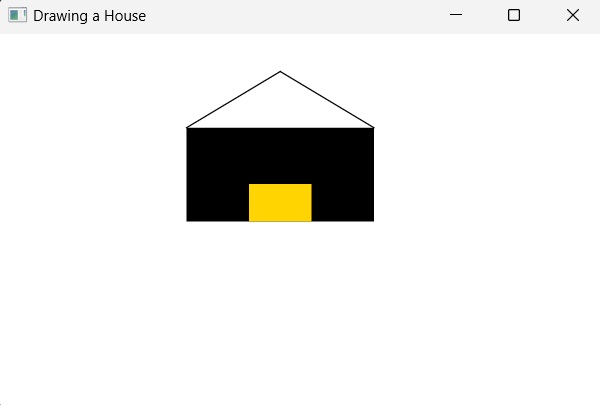