Javafx 简明教程
JavaFX - Intersection Operation
与并集操作类似,交集操作从根本上用于集合论中。顾名思义,该操作被定义为将两个不同的集合交叉到一个集合中。因此,从两个或多个集合中检索共有的元素;忽略重复的元素。然后,这一概念被计算机编程中的各种技术所采用。
例如,它也用于 C、C++、Java、Python 等等的编程语言中,作为一个运算符或方法。同样,JavaFX 也在 2D 形状上提供了交集操作。
Intersection Operation in JavaFX
JavaFX 允许您对 2D 形状执行交集操作,其中,两个或多个形状的区域交叉在一起,并且这些形状的公共区域作为结果被获取。因此,此操作将两个或多个形状作为输入,并将它们之间的交集区域作为结果返回,如下所示。

您可以使用名为 intersect() 的方法对形状执行交集操作。既然这是一个静态方法,您应该使用类名(Shape 或其子类)调用它,如下所示。
Shape shape = Shape.intersect(circle1, circle2);
以下是一个交集操作的示例。在其中,我们绘制两个圆,并对它们执行交集操作。将此代码保存在名为 IntersectionExample.java 的文件中
Example
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
import javafx.scene.shape.Circle;
import javafx.scene.shape.Shape;
public class IntersectionExample extends Application {
@Override
public void start(Stage stage) {
//Drawing Circle1
Circle circle1 = new Circle();
//Setting the position of the circle
circle1.setCenterX(250.0f);
circle1.setCenterY(135.0f);
//Setting the radius of the circle
circle1.setRadius(100.0f);
//Setting the color of the circle
circle1.setFill(Color.DARKSLATEBLUE);
//Drawing Circle2
Circle circle2 = new Circle();
//Setting the position of the circle
circle2.setCenterX(350.0f);
circle2.setCenterY(135.0f);
//Setting the radius of the circle
circle2.setRadius(100.0f);
//Setting the color of the circle
circle2.setFill(Color.BLUE);
//Performing intersection operation on the circle
Shape shape = Shape.intersect(circle1, circle2);
//Setting the fill color to the result
shape.setFill(Color.DARKSLATEBLUE);
//Creating a Group object
Group root = new Group(shape);
//Creating a scene object
Scene scene = new Scene(root, 600, 300);
//Setting title to the Stage
stage.setTitle("Intersection Example");
//Adding scene to the stage
stage.setScene(scene);
//Displaying the contents of the stage
stage.show();
}
public static void main(String args[]){
launch(args);
}
}
使用以下命令,从命令提示符编译并执行已保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls IntersectionExample.java
java --module-path %PATH_TO_FX% --add-modules javafx.controls IntersectionExample
执行上述程序时,生成一个 JavaFX 窗口,显示以下输出 −
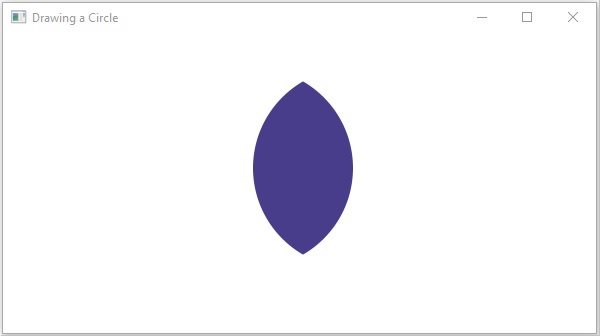
Example
现在,尝试对两个椭圆形状执行交集操作(以检索它们之间的公共区域)。将该文件另存为 EllipseIntersectionOperation.java 。
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
import javafx.scene.shape.Ellipse;
import javafx.scene.shape.Shape;
public class EllipseIntersectionOperation extends Application {
@Override
public void start(Stage stage) {
Ellipse ellipse1 = new Ellipse();
ellipse1.setCenterX(250.0f);
ellipse1.setCenterY(100.0f);
ellipse1.setRadiusX(150.0f);
ellipse1.setRadiusY(75.0f);
ellipse1.setFill(Color.BLUE);
Ellipse ellipse2 = new Ellipse();
ellipse2.setCenterX(350.0f);
ellipse2.setCenterY(100.0f);
ellipse2.setRadiusX(150.0f);
ellipse2.setRadiusY(75.0f);
ellipse2.setFill(Color.RED);
Shape shape = Shape.intersect(ellipse1, ellipse2);
//Setting the fill color to the result
shape.setFill(Color.DARKSLATEBLUE);
//Creating a Group object
Group root = new Group(shape);
//Creating a scene object
Scene scene = new Scene(root, 600, 300);
//Setting title to the Stage
stage.setTitle("Intersection Example");
//Adding scene to the stage
stage.setScene(scene);
//Displaying the contents of the stage
stage.show();
}
public static void main(String args[]){
launch(args);
}
}
使用以下命令,从命令提示符编译并执行已保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls EllipseIntersectionOperation.java
java --module-path %PATH_TO_FX% --add-modules javafx.controls EllipseIntersectionOperation
执行上述程序时,生成一个 JavaFX 窗口,显示以下输出 −
