Javafx 简明教程
JavaFX - TabPane
TabPane 充当一个或多个 Tab 对象的容器。术语 tab 指代显示区域顶部的一个部分,它表示另一个网页或内容。某个网页的内容仅在其对应的选项卡被点击时才会变得可见。在下图中,我们可以看到三个打开的选项卡 −
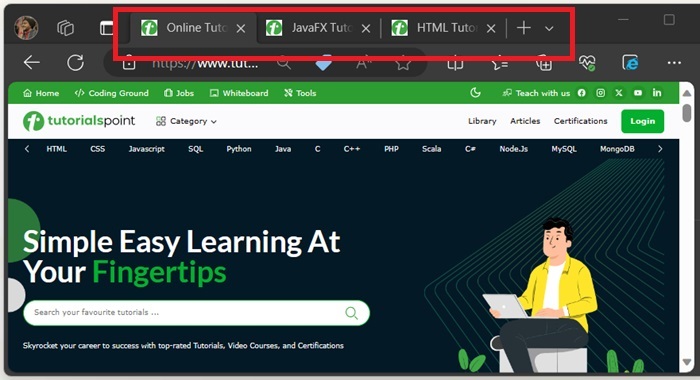
TabPane in JavaFX
在 JavaFX 中,名为 TabPane 的类表示一个选项卡窗格。若要使用选项卡窗格的功能,我们需要创建一个 TabPane 类的实例,并在其内部添加我们想要显示的选项卡。这些选项卡可以包含任何 JavaFX 节点,作为选项卡的内容,例如标签、按钮、图像、文本字段、视频等等。我们可以使用以下任何构造函数来创建选项卡窗格 −
-
TabPane() − 用于创建一个空选项卡窗格。
-
TabPane(Tab tabs) − 这是 TabPane 类的参数化构造函数,它将使用指定选项卡集创建一个新的选项卡窗格。
Steps to create a TabPane in JavaFX
要在 JavaFX 中创建一个 TabPane,请按照以下给定的步骤操作。
Step 1: Create desired number of Tabs
如前所述,选项卡窗格包含一个或多个选项卡。因此,我们的第一步是在选项卡窗格内创建选项卡以供显示。此处,我们将创建三个标签,分别是 Label、Image 和 Video。在 JavaFX 中,通过实例化名为 Tab 的类来创建选项卡,该类属于 javafx.scene.control 包。Tab 对象有一个文本属性,用于设置选项卡的标题。使用以下代码创建选项卡 −
// Creating a Label tab
Tab tab1 = new Tab("Label");
// Creating an Image tab
Tab tab2 = new Tab("Image");
// Creating a Video tab
Tab tab3 = new Tab("Video");
Step 2: Set the content of Tabs
Tab 对象有一个内容属性,用于设置要显示在选项卡正文中的节点。对于此操作,我们使用 setContent() 方法。它与 Tab 对象一起使用,并接受 Node 对象作为其构造函数的参数,如下面的代码所示 −
// setting the Label tab
tab1.setContent(new Label("This is the first tab"));
// setting the Image tab
tab2.setContent(imageView);
// setting the Video tab
tab3.setContent(vbox);
Step 3: Instantiate the TabPane class
要创建选项卡窗格,请实例化 TabPane 包的 javafx.scene.control 类,而不向其构造函数传递任何参数值,并使用 getTabs() 方法将所有选项卡添加到选项卡窗格中。
// Creating a TabPane
TabPane tabPane = new TabPane();
// Adding all the tabs to the TabPane
tabPane.getTabs().addAll(tab1, tab2, tab3);
Step 4: Launching Application
在创建 TabPane 并向其中添加选项卡后,请按照以下给定步骤正确启动应用程序 −
-
首先,通过将 TabPane 对象作为其构造函数的参数值来实例化名为 Scene 的类。此外,将应用程序屏幕的尺寸作为可选参数传递给此构造函数。
-
然后,使用 Stage 类的 setTitle() 方法设置阶段标题。
-
现在,使用名为 Stage 的类的 setScene() 方法将 Scene 对象添加到阶段。
-
使用名为 show() 的方法显示场景的内容。
-
最后,借助 launch() 方法启动应用程序。
Example
在以下示例中,我们将在 JavaFX 应用程序中创建一个 TabPane。将此代码另存为名称为 JavafxtabsDemo.java 的文件。
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.Tab;
import javafx.scene.control.TabPane;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.media.Media;
import javafx.scene.media.MediaPlayer;
import javafx.scene.media.MediaView;
import javafx.stage.Stage;
import java.io.File;
import javafx.scene.layout.VBox;
import javafx.scene.layout.HBox;
import javafx.scene.control.Button;
import javafx.geometry.Pos;
public class JavafxtabsDemo extends Application {
@Override
public void start(Stage stage) {
// Creating a Label tab
Tab tab1 = new Tab("Label");
tab1.setContent(new Label("This is the first tab"));
// Creating an Image tab
Tab tab2 = new Tab("Image");
Image image = new Image("tutorials_point.jpg");
ImageView imageView = new ImageView(image);
tab2.setContent(imageView);
// Creating a Video tab
Tab tab3 = new Tab("Video");
// Passing the video file to the File object
File videofile = new File("sampleTP.mp4");
// creating a Media object from the File Object
Media videomedia = new Media(videofile.toURI().toString());
// creating a MediaPlayer object from the Media Object
MediaPlayer mdplayer = new MediaPlayer(videomedia);
// creating a MediaView object from the MediaPlayer Object
MediaView viewmedia = new MediaView(mdplayer);
//setting the fit height and width of the media view
viewmedia.setFitHeight(350);
viewmedia.setFitWidth(350);
// creating video controls using the buttons
Button pause = new Button("Pause");
Button resume = new Button("Resume");
// creating an HBox
HBox box = new HBox(20);
box.setAlignment(Pos.CENTER);
box.getChildren().addAll(pause, resume);
// function to handle play and pause buttons
pause.setOnAction(act -> mdplayer.pause());
resume.setOnAction(act -> mdplayer.play());
// creating the root
VBox vbox = new VBox(20);
vbox.setAlignment(Pos.CENTER);
vbox.getChildren().addAll(viewmedia, box);
tab3.setContent(vbox);
// Creating a TabPane
TabPane tabPane = new TabPane();
// Adding all the tabs to the TabPane
tabPane.getTabs().addAll(tab1, tab2, tab3);
// Create a Scene with the TabPane as its root
Scene scene = new Scene(tabPane, 400, 400);
// Set the Title on the Stage
stage.setTitle("TabPane in JavaFX");
// Set the Scene on the Stage
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
要从命令提示符编译并执行已保存的 Java 文件,请使用以下命令−
javac --module-path %PATH_TO_FX% --add-modules javafx.controls,javafx.media JavafxtabsDemo.java
java --module-path %PATH_TO_FX% --add-modules javafx.controls,javafx.media JavafxtabsDemo
当我们执行上述代码时,它将生成一个 TabPane,其中包含三个选项卡,分别是 Label、Image 和 Video。
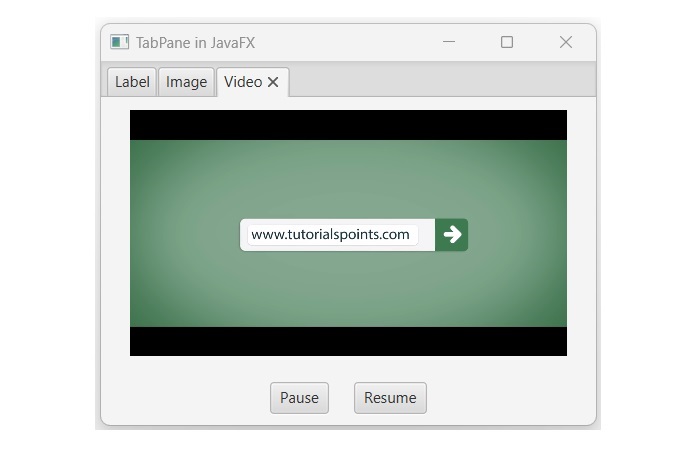