Javafx 简明教程
JavaFX - Drawing a Circle
圆是所有点到固定点(圆心)的固定距离(圆的半径)的轨迹。换句话说,圆是一条形成闭合环路的线,其上的每一点都与中心点保持固定距离。
圆由两个参数定义,即 −
-
Centre − 它是圆内的一个点。圆上的所有点与中心点的距离相等(距离相同)。
-
Radius − 半径是圆心到圆上任意一点的距离。它等于直径的一半。
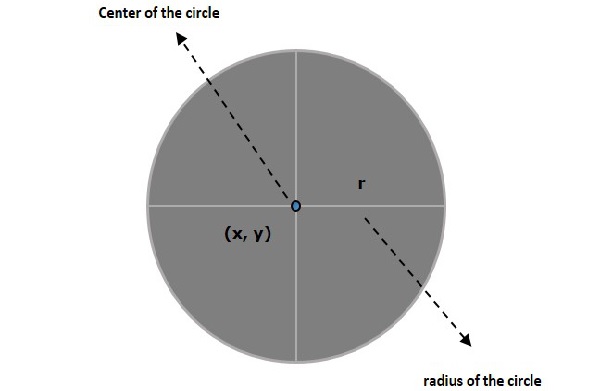
Circle in JavaFX
在 JavaFX 中,圆形由名为 Circle 的类表示。此类属于 javafx.scene.shape 包。
通过实例化此类,你可以在 JavaFX 中创建一个圆形节点。
此类有 3 个双数据类型的属性,分别是:
-
centerX − 圆心 x 坐标。
-
centerY − 圆心 y 坐标。
-
radius − 圆的半径(像素)。
要绘制一个圆,需要将值传递给这些属性,方法是在实例化时按相同顺序将它们传递给此类的构造函数,或使用设置器方法。
Steps to Draw a Circle
按照以下给出的步骤在 JavaFX 中绘制一个圆形。
Step 1: Creating a Circle
可以通过实例化属于包 javafx.scene.shape 的名为 Circle 的类在 JavaFX 中创建一个圆形。整个 JavaFX 代码必须编写在 Application 类的 start() 方法中,如下所示:
public class ClassName extends Application {
public void start(Stage primaryStage) throws Exception {
// Creating a circle object
Circle circle = new Circle();
}
}
Step 2: Setting Properties to the Circle
在 start() 方法中,使用各自的设置器方法设置属性 X、Y 和 radius 的值,指定圆心 x、y 坐标以及圆的半径,如下面的代码块所示。
circle.setCenterX(300.0f);
circle.setCenterY(135.0f);
circle.setRadius(100.0f);
Step 3: Adding Circle Object to Group
通过将圆形对象作为参数值传递给其构造函数,实例化包 javafx.scene 中的 Group 类,如下所示:
Group root = new Group(circle);
Step 4: Launching Application
创建二维对象后,请按照下面给出的步骤正确启动应用程序:
-
首先,通过将组对象作为其构造函数的参数值传递来实例化名为 Scene 的类。你也可以将应用程序屏幕的尺寸作为可选参数传递给此构造函数。
-
然后,使用 Stage 类的 setTitle() 方法设置阶段标题。
-
现在,使用名为 Stage 的类的 setScene() 方法将 Scene 对象添加到阶段。
-
使用名为 show() 的方法显示场景的内容。
-
最后,借助 launch() 方法启动应用程序。
Example
以下是使用 JavaFX 生成圆形的程序。将此代码保存在名为 CircleExample.java 的文件中。
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.stage.Stage;
import javafx.scene.shape.Circle;
public class CircleExample extends Application {
@Override
public void start(Stage stage) {
//Drawing a Circle
Circle circle = new Circle();
//Setting the properties of the circle
circle.setCenterX(300.0f);
circle.setCenterY(135.0f);
circle.setRadius(100.0f);
//Creating a Group object
Group root = new Group(circle);
//Creating a scene object
Scene scene = new Scene(root, 600, 300);
//Setting title to the Stage
stage.setTitle("Drawing a Circle");
//Adding scene to the stage
stage.setScene(scene);
//Displaying the contents of the stage
stage.show();
}
public static void main(String args[]){
launch(args);
}
}
使用以下命令,从命令提示符编译并执行已保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls CircleExample.java
java --module-path %PATH_TO_FX% --add-modules javafx.controls CircleExample
在执行时,上面的程序会生成一个显示圆形的 JavaFX 窗口,如下所示。
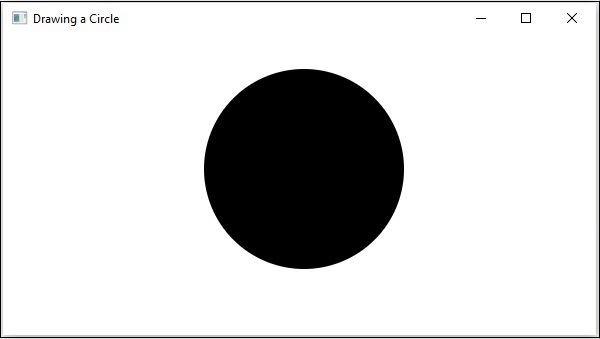
Example
让我们看另一个示例,将圆形绘制成奥运会标志的一部分。将文件命名为 OlympicCircle.java 。
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
import javafx.scene.shape.Circle;
public class OlympicCircle extends Application {
@Override
public void start(Stage stage) {
//Drawing a Circle
Circle circle1 = new Circle(100.0f, 50.0f, 50.0f);
circle1.setStroke(Color.BLUE);
circle1.setFill(Color.WHITE);
Circle circle2 = new Circle(175.0f, 50.0f, 50.0f);
circle2.setStroke(Color.BLACK);
circle2.setFill(Color.WHITE);
Circle circle3 = new Circle(250.0f, 50.0f, 50.0f);
circle3.setStroke(Color.RED);
circle3.setFill(Color.WHITE);
Circle circle4 = new Circle(75.0f, 125.0f, 50.0f);
circle4.setStroke(Color.YELLOW);
circle4.setFill(Color.WHITE);
Circle circle5 = new Circle(150.0f, 125.0f, 50.0f);
circle5.setStroke(Color.GREEN);
circle5.setFill(Color.WHITE);
//Creating a Group object
Group root = new Group();
root.getChildren().addAll(circle1,circle2,circle3,circle4,circle5);
//Creating a scene object
Scene scene = new Scene(root, 600, 300);
//Setting title to the Stage
stage.setTitle("Drawing the Olympics Symbol");
//Adding scene to the stage
stage.setScene(scene);
//Displaying the contents of the stage
stage.show();
}
public static void main(String args[]){
launch(args);
}
}
使用以下命令,从命令提示符编译并执行已保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls OlympicCircle.java
java --module-path %PATH_TO_FX% --add-modules javafx.controls OlympicCircle
在执行时,上面的程序会生成一个显示奥运会标志的 JavaFX 窗口,如下所示。
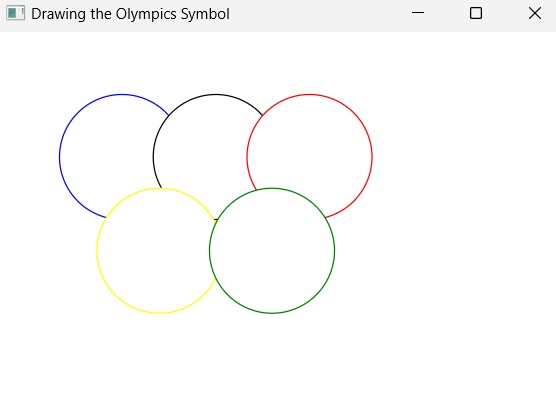