Javafx 简明教程
JavaFX - Fade Transition
淡入过渡是一种几何过渡,它会改变对象的 opacity 属性。使用淡入过渡,您可以减少对象的 opacity 或增加它。这种过渡被称为几何过渡,因为它涉及对象的几何形状。
A Fade transition is a type of a geometrical transition that changes the opacity property of an object. Using fade transition, you can either reduce the opacity of the object or increase it. This transition is known as a geometrical transition as it deals with the geometry of an object.
在 JavaFX 中,淡入过渡用于将节点的 opacity 从起始值过渡到指定的持续时间内的结束值。这可以使用 FadeTransition 类来完成,该类属于 javafx.animation 包。
In JavaFX, the fade transition is used to transition a node’s opacity from a starting value to an ending value over a specified duration. This can be done using the FadeTransition class belonging to the javafx.animation package.
Fade Transition in JavaFX
您可以使用 FadeTransition 类属性将淡入过渡应用到 JavaFX 对象。您必须使用 fromValue 和 toValue 设置过渡的起始值和结束值。当未指定 fromValue 时,默认情况下会考虑节点的当前 opacity 值;当未指定 toValue 时,byValue 会添加到起始值中。
You can apply the fade transition to a JavaFX object using the FadeTransition class properties. You have to set the starting value and ending value of the transition using fromValue and toValue. When fromValue is not specified, the node’s current opacity value is considered by default; and when toValue is not specified, the byValue is added to the starting value.
Note that Transition properties − fromValue、toValue 和 byValue 在过渡运行时不能更改。但是,如果您尝试在过渡运行时更改属性,则此更改将被忽略。因此,必须停止并重新启动动画才能将新的属性值分配给过渡。也不会抛出任何异常。
Note that Transition properties − fromValue, toValue and byValue, cannot be changed while a transition is running. However, if you attempt to change a property while the transition is running, the change is ignored. Hence, the animation must be stopped and restarted to assign the new property value(s) to the transition. No exception is thrown either.
FadeTransition 类包含以下属性 −
The FadeTransition class contains the following properties −
-
byValue − Specifies the incremented stop opacity value, from the start, of this FadeTransition.
-
duration − The duration of this FadeTransition.
-
fromValue − Specifies the start opacity value for this FadeTransition.
-
node − The target node of this Transition.
-
toValue − Specifies the stop opacity value for this FadeTransition.
Example
以下是演示 JavaFX 中淡入过渡的程序。将此代码保存在名为 FadeTransitionExample.java 的文件中。
Following is the program which demonstrates Fade Transition in JavaFX. Save this code in a file with the name FadeTransitionExample.java.
import javafx.animation.FadeTransition;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.stage.Stage;
import javafx.util.Duration;
public class FadeTransitionExample extends Application {
@Override
public void start(Stage stage) {
//Drawing a Circle
Circle circle = new Circle();
//Setting the position of the circle
circle.setCenterX(300.0f);
circle.setCenterY(135.0f);
//Setting the radius of the circle
circle.setRadius(100.0f);
//Setting the color of the circle
circle.setFill(Color.BROWN);
//Setting the stroke width of the circle
circle.setStrokeWidth(20);
//Creating the fade Transition
FadeTransition fadeTransition = new FadeTransition(Duration.millis(1000));
//Setting the node for Transition
fadeTransition.setNode(circle);
//Setting the property fromValue of the transition (opacity)
fadeTransition.setFromValue(1.0);
//Setting the property toValue of the transition (opacity)
fadeTransition.setToValue(0.3);
//Setting the cycle count for the transition
fadeTransition.setCycleCount(50);
//Setting auto reverse value to false
fadeTransition.setAutoReverse(false);
//Playing the animation
fadeTransition.play();
//Creating a Group object
Group root = new Group(circle);
//Creating a scene object
Scene scene = new Scene(root, 600, 300);
//Setting title to the Stage
stage.setTitle("Fade transition example");
//Adding scene to the stage
stage.setScene(scene);
//Displaying the contents of the stage
stage.show();
}
public static void main(String args[]){
launch(args);
}
}
使用以下命令,从命令提示符编译并执行已保存的 java 文件。
Compile and execute the saved java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls FadeTransitionExample.java
java --module-path %PATH_TO_FX% --add-modules javafx.controls FadeTransitionExample
执行后,上述程序会生成如下所示的 JavaFX 窗口。
On executing, the above program generates a JavaFX window as shown below.
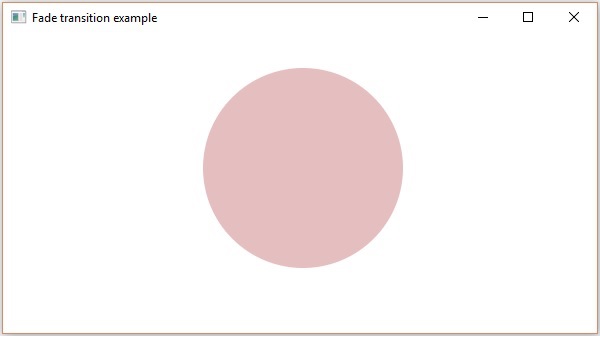