Javafx 简明教程
JavaFX - Drawing a Rounded Rectangle
在几何学中, rounded rectangle 被定义为一个包含圆弧边缘而不是锐角边缘的二维图形。它是通过将四个不同的圆形放置在尖角矩形上,圆心作为它们的中心而获得的。此圆角矩形的边缘是针对这些圆绘制的公共切线,如下图所示。
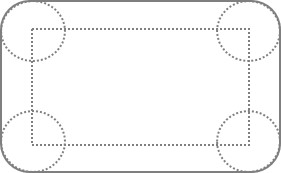
在圆角矩形中,长度和宽度与包裹在其中的尖角矩形相同。
Rounded Rectangle in JavaFX
在 JavaFX 中,您可以绘制带有锐角边缘或圆弧边缘的矩形,如下面的图表所示。
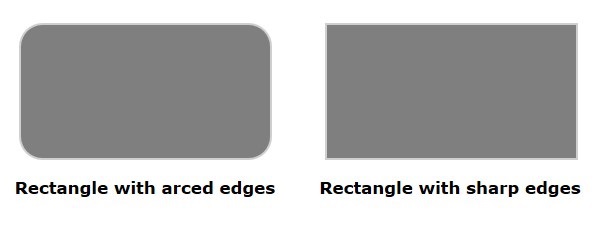
带圆弧边缘的矩形被称为圆角矩形,因为它不包含顶点。此图形主要用于设计和光学学的各种应用,并有两个附加属性,即:
-
arcHeight − 圆角矩形角处的圆弧的垂直直径。
-
arcWidth − 圆角矩形角处的圆弧的水平直径。

默认情况下,JavaFX 创建一个边角锐利的矩形,除非使用 setter 方法 setArcHeight() 和 setArcWidth() 将弧形的高度和宽度设为正值 (>0)。
Drawing Rounded Rectangle
为了在 JavaFX 中绘制圆角矩形,你还需要在 javafx.scene.shape 包中实例化名为 Rectangle 的类。此外,你还要设圆角矩形的弧形属性,如下所示:
rectangle.setX(150.0f);
rectangle.setY(75.0f);
rectangle.setWidth(300.0f);
rectangle.setHeight(150.0f);
rectangle.setArcWidth(30.0);
rectangle.setArcHeight(20.0);
按照本教程 Drawing a Rectangle 章节中提及的所有其他步骤启动一个带圆角矩形的 JavaFX 应用程序。
Example
下面是一个使用 JavaFX 生成了一个圆角矩形的程序。将此代码保存在一个名为 RoundedRectangle.java 的文件中。
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.stage.Stage;
import javafx.scene.shape.Rectangle;
public class RoundedRectangle extends Application {
@Override
public void start(Stage stage) {
//Drawing a Rectangle
Rectangle rectangle = new Rectangle();
//Setting the properties of the rectangle
rectangle.setX(150.0f);
rectangle.setY(75.0f);
rectangle.setWidth(300.0f);
rectangle.setHeight(150.0f);
//Setting the height and width of the arc
rectangle.setArcWidth(30.0);
rectangle.setArcHeight(20.0);
//Creating a Group object
Group root = new Group(rectangle);
//Creating a scene object
Scene scene = new Scene(root, 600, 300);
//Setting title to the Stage
stage.setTitle("Drawing a Rectangle");
//Adding scene to the stage
stage.setScene(scene);
//Displaying the contents of the stage
stage.show();
}
public static void main(String args[]){
launch(args);
}
}
使用以下命令,从命令提示符编译并执行已保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls RoundedRectangle.java
java --module-path %PATH_TO_FX% --add-modules javafx.controls RoundedRectangle
在执行过程中,以上程序生成一个显示一个圆角矩形的 JavaFX 窗口,如下所示。

Example
让我们看另一个使用 JavaFX 绘制圆角矩形的示例。此外,在此示例中让我们应用一些 CSS,比如添加一个背景颜色。将此代码保存在一个名为 CSSRoundedRectangle.java 的文件中。
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.stage.Stage;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
public class CSSRoundedRectangle extends Application {
@Override
public void start(Stage stage) {
//Drawing a Rectangle
Rectangle rectangle = new Rectangle();
//Setting the properties of the rectangle
rectangle.setX(50.0f);
rectangle.setY(75.0f);
rectangle.setWidth(200.0f);
rectangle.setHeight(150.0f);
//Setting the height and width of the arc
rectangle.setArcWidth(30.0);
rectangle.setArcHeight(20.0);
//Colour the rounded rectangle
rectangle.setFill(Color.DARKBLUE);
//Creating a Group object
Group root = new Group(rectangle);
//Creating a scene object
Scene scene = new Scene(root, 300, 300);
scene.setFill(Color.RED);
//Setting title to the Stage
stage.setTitle("Drawing a Rectangle");
//Adding scene to the stage
stage.setScene(scene);
//Displaying the contents of the stage
stage.show();
}
public static void main(String args[]){
launch(args);
}
}
使用以下命令,从命令提示符编译并执行已保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls CSSRoundedRectangle.java
java --module-path %PATH_TO_FX% --add-modules javafx.controls CSSRoundedRectangle
在执行过程中,以上程序生成一个显示一个圆角矩形的 JavaFX 窗口,如下所示。
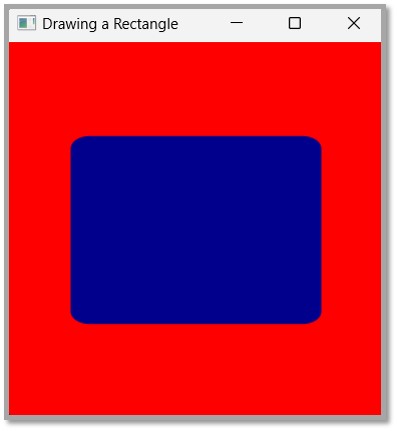