Javafx 简明教程
JavaFX - Translation Transformation
平移变换只是将一个对象移动到同一平面上不同的位置。你可以通过向原始坐标 (X, Y) 添加平移坐标 (tx, ty) 来平移二维平面中的一个点;这将得到点平移到的新坐标 (X', Y')。
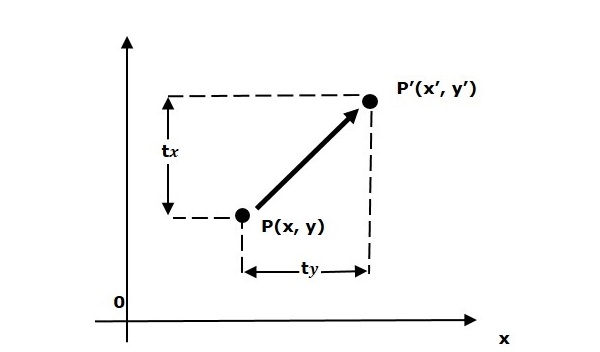
Translation Transformation in JavaFX
在 JavaFX 中,使用平移变换,可以将一个节点从一个位置移动到同一应用程序中的另一个位置。此变换借助 Translate class 中的 javafx.scene.transform 包应用于 JavaFX 节点。这个类表示一个 Affine 对象,它重新定位了 JavaFX 应用程序中的坐标。
Example 1
以下是演示 JavaFX 中平移的程序。在此,我们正在同一位置创建 2 个圆(节点),尺寸相同,但颜色不同(棕褐色和军蓝色)。我们还在 cadetblue 颜色的圆上应用平移。
将这段代码保存在名为 TranslationExample.java 的文件中。
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.scene.transform.Translate;
import javafx.stage.Stage;
public class TranslationExample extends Application {
@Override
public void start(Stage stage) {
//Drawing Circle1
Circle circle = new Circle();
//Setting the position of the circle
circle.setCenterX(150.0f);
circle.setCenterY(135.0f);
//Setting the radius of the circle
circle.setRadius(100.0f);
//Setting the color of the circle
circle.setFill(Color.BROWN);
//Setting the stroke width of the circle
circle.setStrokeWidth(20);
//Drawing Circle2
Circle circle2 = new Circle();
//Setting the position of the circle
circle2.setCenterX(150.0f);
circle2.setCenterY(135.0f);
//Setting the radius of the circle
circle2.setRadius(100.0f);
//Setting the color of the circle
circle2.setFill(Color.CADETBLUE);
//Setting the stroke width of the circle
circle2.setStrokeWidth(20);
//Creating the translation transformation
Translate translate = new Translate();
//Setting the X,Y,Z coordinates to apply the translation
translate.setX(300);
translate.setY(50);
translate.setZ(100);
//Adding transformation to circle2
circle2.getTransforms().addAll(translate);
//Creating a Group object
Group root = new Group(circle,circle2);
//Creating a scene object
Scene scene = new Scene(root, 600, 300);
//Setting title to the Stage
stage.setTitle("Translation transformation example");
//Adding scene to the stage
stage.setScene(scene);
//Displaying the contents of the stage
stage.show();
}
public static void main(String args[]){
launch(args);
}
}
使用以下命令,从命令提示符编译并执行已保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls TranslationExample.java
java --module-path %PATH_TO_FX% --add-modules javafx.controls TranslationExample
执行后,上述程序会生成如下所示的 JavaFX 窗口。

Example 2
在以下示例中,我们演示了 3D 形状上的平移。将这段代码保存在名为 TranslationExample3D.java 的文件中。
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.Cylinder;
import javafx.scene.transform.Translate;
import javafx.stage.Stage;
public class TranslationExample3D extends Application {
@Override
public void start(Stage stage) {
Cylinder cy1 = new Cylinder(50, 100);
Cylinder cy2 = new Cylinder(50, 100);
//Creating the translation transformation
Translate translate = new Translate();
//Setting the X,Y,Z coordinates to apply the translation
translate.setX(200);
translate.setY(150);
translate.setZ(100);
//Adding transformation to circle2
cy2.getTransforms().addAll(translate);
//Creating a Group object
Group root = new Group(cy1,cy2);
//Creating a scene object
Scene scene = new Scene(root, 400, 300);
//Setting title to the Stage
stage.setTitle("Translation transformation example");
//Adding scene to the stage
stage.setScene(scene);
//Displaying the contents of the stage
stage.show();
}
public static void main(String args[]){
launch(args);
}
}
使用以下命令,从命令提示符编译并执行已保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls TranslationExample3D.java
java --module-path %PATH_TO_FX% --add-modules javafx.controls TranslationExample3D
执行后,上述程序会生成如下所示的 JavaFX 窗口。
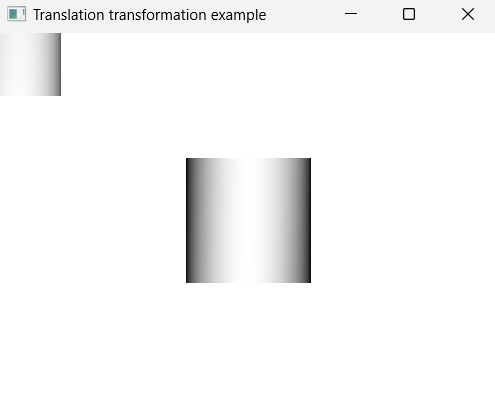
正如我们所看到的,由于圆柱体的原始位置,我们无法像在平移图像中那样清晰地看到完整图像。因此,在像这样的情况下,平移变换就变得有必要。