Javafx 简明教程
JavaFX - Drawing an Ellipse
椭圆由两点定义,每一点都称为焦点。如果取椭圆上的任何一点,到焦点点的距离的总和是常数。椭圆的大小由这两个距离的总和决定。这些距离的总和等于长轴(椭圆最长直径)的长度。事实上,圆是椭圆的特例。
An Ellipse is defined by two points, each called a focus. If any point on the Ellipse is taken, the sum of the distances to the focus points is constant. The size of the Ellipse is determined by the sum of these two distances. The sum of these distances is equal to the length of the major axis (the longest diameter of the ellipse). A circle is, in fact, a special case of an Ellipse.
椭圆有以下三个属性: −
An Ellipse has three properties which are −
-
Centre − A point inside the Ellipse which is the midpoint of the line segment linking the two foci. The intersection of the major and minor axes.
-
Major axis − The longest diameter of an ellipse.
-
Minor axis − The shortest diameter of an ellipse.

Ellipse in JavaFX
在 JavaFX 中,椭圆由名为 Ellipse 的类表示。此类属于包 javafx.scene.shape 。
In JavaFX, an Ellipse is represented by a class named Ellipse. This class belongs to the package javafx.scene.shape.
通过实例化此类,可以在 JavaFX 中创建椭圆节点。
By instantiating this class, you can create an Ellipse node in JavaFX.
此类具有 4 个双数据类型的属性,即 −
This class has 4 properties of the double datatype namely −
-
centerX − The x coordinate of the center of the ellipse in pixels.
-
centerY − The y coordinate of the center of the ellipse in pixels.
-
radiusX − The width of the ellipse pixels.
-
radiusY − The height of the ellipse pixels.
要绘制椭圆,需要将值传递给这些属性,要么将它们按相同順序传递给此类的构造函数,要么使用设置器方法。
To draw an ellipse, you need to pass values to these properties, either by passing them to the constructor of this class in the same order, or by using setter methods.
Steps to Draw Ellipse
按照以下给出的步骤在 JavaFX 中绘制椭圆。
Follow the steps given below to draw an Ellipse in JavaFX.
Step 1: Creating an Ellipse
可以通过在 start() 方法内部实例化名为 Ellipse 的类在 JavaFX 中创建椭圆,该类属于包 javafx.scene.shape 。你可以按如下方式实例化该类。
You can create an Ellipse in JavaFX by instantiating the class named Ellipse which belongs to a package javafx.scene.shape, inside the start() method. You can instantiate this class as follows.
public class ClassName extends Application {
public void start(Stage primaryStage) throws Exception {
//Creating an Ellipse object
Ellipse ellipse = new Ellipse();
}
}
Step 2: Setting Properties to the Ellipse
指定椭圆中心的 x、y 坐标 →椭圆沿 x 轴和 y 轴(主轴和次轴)的宽度,通过设置 X、Y、RadiusX 和 RadiusY 属性来设置圆的半径。
Specify the x, y coordinates of the center of the Ellipse → the width of the Ellipse along x axis and y axis (major and minor axises), of the circle by setting the properties X, Y, RadiusX and RadiusY.
可以使用它们各自的 setter 方法执行此操作,如下面的代码块所示。
This can be done by using their respective setter methods as shown in the following code block.
ellipse.setCenterX(300.0f);
ellipse.setCenterY(150.0f);
ellipse.setRadiusX(150.0f);
ellipse.setRadiusY(75.0f);
Step 3: Creating a Group Object
在 start() 方法中,通过实例化名为 Group 的类创建一个组对象,该类属于 javafx.scene 包。通过将上一步中创建的 Ellipse(节点)对象作为参数传递给 Group 类的构造函数来实例化此类。应该这样操作才能将其添加到组中,如下面的代码块所示 −
In the start() method, create a group object by instantiating the class named Group, which belongs to the package javafx.scene. This class is instantiated by passing the Ellipse (node) object created in the previous step as a parameter to the constructor of the Group class. This should be done in order to add it to the group as shown in the following code block −
Group root = new Group(ellipse);
Step 4: Launching Application
创建二维对象后,请按照下面给出的步骤正确启动应用程序:
Once the 2D object is created, follow the given steps below to launch the application properly −
-
Firstly, instantiate the class named Scene by passing the Group object as a parameter value to its constructor. To this constructor, you can also pass dimensions of the application screen as optional parameters.
-
Then, set the title to the stage using the setTitle() method of the Stage class.
-
Now, a Scene object is added to the stage using the setScene() method of the class named Stage.
-
Display the contents of the scene using the method named show().
-
Lastly, the application is launched with the help of the launch() method.
Example
下面是一个使用 JavaFX 生成椭圆的程序。将此代码保存在名为 EllipseExample.java 的文件中。
Following is a program which generates an Ellipse using JavaFX. Save this code in a file with the name EllipseExample.java.
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.stage.Stage;
import javafx.scene.shape.Ellipse;
public class EllipseExample extends Application {
@Override
public void start(Stage stage) {
//Drawing an ellipse
Ellipse ellipse = new Ellipse();
//Setting the properties of the ellipse
ellipse.setCenterX(300.0f);
ellipse.setCenterY(150.0f);
ellipse.setRadiusX(150.0f);
ellipse.setRadiusY(75.0f);
//Creating a Group object
Group root = new Group(ellipse);
//Creating a scene object
Scene scene = new Scene(root, 600, 300);
//Setting title to the Stage
stage.setTitle("Drawing an Ellipse");
//Adding scene to the stage
stage.setScene(scene);
//Displaying the contents of the stage
stage.show();
}
public static void main(String args[]){
launch(args);
}
}
使用以下命令从命令提示符处编译并执行已保存的 Java 文件。
Compile and execute the saved Java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls EllipseExample.java
java --module-path %PATH_TO_FX% --add-modules javafx.controls EllipseExample
在执行时,以上程序生成了一个 JavaFX 窗口,显示一个如下图所示的椭圆。
On executing, the above program generates a JavaFX window displaying an ellipse as shown below.

Example
在下面给出的另一个示例中,我们尝试绘制一个圆形行星的椭圆轨道。将此文件命名为 PlanetOrbit.java 。
In another example given below, let us try to draw an elliptical orbit of a circular planet. Name this file as PlanetOrbit.java.
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.stage.Stage;
import javafx.scene.shape.Ellipse;
import javafx.scene.shape.Circle;
import javafx.scene.paint.Color;
import javafx.animation.PathTransition;
import javafx.application.Application;
import javafx.stage.Stage;
import javafx.util.Duration;
public class PlanetOrbit extends Application {
@Override
public void start(Stage stage) {
//Drawing an orbit
Ellipse orbit = new Ellipse();
orbit.setFill(Color.WHITE);
orbit.setStroke(Color.BLACK);
//Setting the properties of the ellipse
orbit.setCenterX(300.0f);
orbit.setCenterY(150.0f);
orbit.setRadiusX(150.0f);
orbit.setRadiusY(100.0f);
// Drawing a circular planet
Circle planet = new Circle(300.0f, 50.0f, 40.0f);
//Creating the animation
PathTransition pathTransition = new PathTransition();
pathTransition.setDuration(Duration.millis(1000));
pathTransition.setNode(planet);
pathTransition.setPath(orbit);
pathTransition.setOrientation(PathTransition.OrientationType.ORTHOGONAL_TO_TANGENT);
pathTransition.setCycleCount(50);
pathTransition.setAutoReverse(false);
pathTransition.play();
//Creating a Group object
Group root = new Group();
root.getChildren().addAll(orbit, planet);
//Creating a scene object
Scene scene = new Scene(root, 600, 300);
//Setting title to the Stage
stage.setTitle("Drawing a Planet Orbit");
//Adding scene to the stage
stage.setScene(scene);
//Displaying the contents of the stage
stage.show();
}
public static void main(String args[]){
launch(args);
}
}
使用以下命令从命令提示符处编译并执行已保存的 Java 文件。
Compile and execute the saved Java file from the command prompt using the following commands.
javac --module-path %PATH_TO_FX% --add-modules javafx.controls PlanetOrbit.java
java --module-path %PATH_TO_FX% --add-modules javafx.controls PlanetOrbit
在执行时,以上程序生成了一个 JavaFX 窗口,显示一个如下图所示的轨道。
On executing, the above program generates a JavaFX window displaying an orbit as shown below.
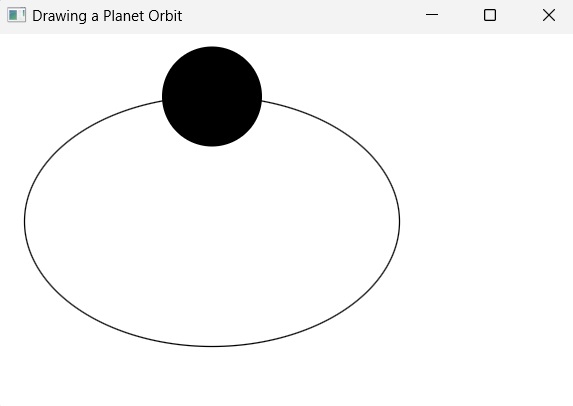