Javafx 简明教程
JavaFX - TilePane Layout
TilePane Layout in JavaFX
在 JavaFX 中, TilePane 是一个布局组件,它以统一的尺寸单元水平或垂直排列其子节点。我们可以控制行或列的数量、单元之间的间隙、窗格的对齐方式以及每个单元的首选大小。 javafx.scene.layout 包中的 TilePane 类表示 TilePane。要创建 TilePane,我们可以使用以下任何构造函数 -
-
TilePane() - 构造一个新的水平 TilePane 布局。
-
TilePane(double hGap, double vGap) - 创建一个具有指定 hGap 和 vGap 的新水平 TilePane 布局。
-
TilePane(double hGap, double vGap, Node childNodes) - 使用指定 hGap、vGap 和节点构造一个水平 TilePane 布局。
-
TilePane(Orientation orientation) - 创建一个具有指定方向的新 TilePane 布局。它可以为水平或垂直。
此类提供 11 个属性,如下所示 -
S.No |
properties & Description |
1 |
alignment*This property represents the alignment of the pane and its value can be set by using the *setAlignment() 方法。 |
2 |
hgap 此属性为 double 类型,它表示一行中每个单元之间的水平间隙。 |
3 |
vgap 此属性为 double 类型,它表示一行中每个单元之间的垂直间隙。 |
4 |
orientation 此属性表示一行中单元的方向。 |
5 |
prefColumns 此属性为 double 类型,它表示水平单元窗格的首选列数。 |
6 |
prefRows 此属性为 double 类型,它表示垂直单元窗格的首选行数。 |
7 |
prefTileHeight 此属性为 double 类型,它表示每个单元的首选高度。 |
8 |
prefTileWidth 此属性为 double 类型,它表示每个单元的首选宽度。 |
9 |
tileHeight 此属性为 double 类型,它表示每个单元的实际高度。 |
10 |
tileWidth 此属性为 double 类型,它表示每个单元的实际宽度。 |
11 |
*tileAlignment*此属性为 double 类型,表示每个子项在其平铺中的默认对齐方式。 |
Example
以下程序是平铺窗格布局的示例。在其中,我们正在创建一个包含 7 个按钮的平铺窗格。默认情况下,其方向为水平。使用名称 TilePaneExample.java. 将此代码保存在文件中
import javafx.application.Application;
import javafx.geometry.Orientation;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.TilePane;
import javafx.stage.Stage;
public class TilePaneExample extends Application {
@Override
public void start(Stage stage) {
//Creating an array of Buttons
Button[] buttons = new Button[] {
new Button("SunDay"),
new Button("MonDay"),
new Button("TuesDay"),
new Button("WednesDay"),
new Button("ThursDay"),
new Button("FriDay"),
new Button("SaturDay")
};
//Creating a Tile Pane
TilePane tilePane = new TilePane();
//Setting the alignment for the Tile Pane
tilePane.setTileAlignment(Pos.CENTER_LEFT);
//Setting the preferred columns for the Tile Pane
tilePane.setPrefRows(4);
//Adding the array of buttons to the pane
tilePane.getChildren().addAll(buttons);
//Creating a scene object
Scene scene = new Scene(tilePane, 400, 300);
//Setting title to the Stage
stage.setTitle("Tile Pane Example");
//Adding scene to the stage
stage.setScene(scene);
//Displaying the contents of the stage
stage.show();
}
public static void main(String args[]){
launch(args);
}
}
使用以下命令,从命令提示符编译并执行已保存的 java 文件。
javac --module-path %PATH_TO_FX% --add-modules javafx.controls TilePaneExample.java
java --module-path %PATH_TO_FX% --add-modules javafx.controls TilePaneExample
执行后,上述程序会生成如下所示的 JavaFX 窗口。
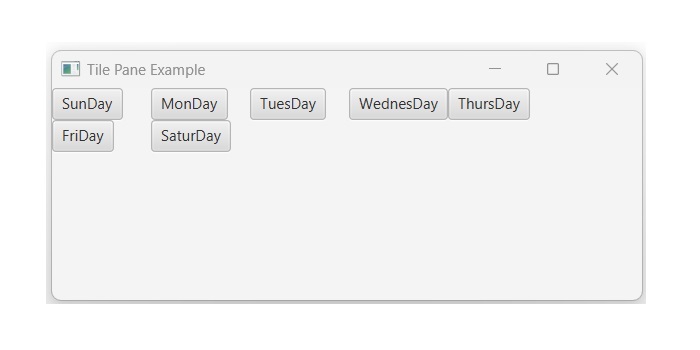
Setting the Orientation of TilePane to Vertical
要使用 JavaFX 设置 TilePane 的方向,我们要么使用名为 setOrientation() 的内置方法,要么使用其接受方向作为参数值的带参构造函数。以下 JavaFX 代码演示了如何将 TilePane 的方向设置为垂直。使用名称 JavafxTilepane 将此 JavaFX 代码保存在文件中。
Example
import javafx.application.Application;
import javafx.geometry.Orientation;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.TilePane;
import javafx.stage.Stage;
public class JavafxTilepane extends Application {
@Override
public void start(Stage stage) {
// Creating a TilePane with vertical orientation
TilePane tileP = new TilePane(Orientation.VERTICAL);
// Setting the preferred number of rows to three
tileP.setPrefRows(3);
// Setting the hGap and vGap between tiles
tileP.setHgap(10);
tileP.setVgap(10);
// Setting the alignment of the pane and the tiles
tileP.setAlignment(Pos.CENTER);
tileP.setTileAlignment(Pos.CENTER);
// To add 10 buttons to the pane
for (int i = 1; i <= 10; i++) {
Button button = new Button("Button " + i);
tileP.getChildren().add(button);
}
// Create a scene and stage
Scene scene = new Scene(tileP, 400, 300);
stage.setTitle("TilePane in JavaFX");
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
要从命令提示符编译并执行已保存的 Java 文件,请使用以下命令−
javac --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxTilepane.java
java --module-path %PATH_TO_FX% --add-modules javafx.controls JavafxTilepane
当我们执行上述代码时,它将生成以下输出 −
