Matplotlib 简明教程
Matplotlib - Event Handling
在一般编程中, event 定义为对象状态的变化,它发生在用户与图形用户界面组件交互时,触发应用程序的响应。考虑以下操作:单击按钮、移动鼠标、在键盘上打字、从列表中选择一项或滚动页面 - 其中每项活动都是一个事件,描述源的状态变化。
而 event handling 是交互式软件应用程序的主干。它是控制对这些事件的响应的机制,确定在特定事件发生时应该发生什么。
Event Handling in Matplotlib
Matplotlib 与各种用户界面工具包配合使用,包括 wxPython、Tkinter、Qt、GTK 和 MacOSX。为了确保对不同界面中的交互式功能(如平移和缩放)提供一致的支持,Matplotlib 使用 GUI-neutral 事件处理 API。该 API 最初基于 GTK 模型,它是 Matplotlib 支持的第一个用户界面。
Connecting to Events
在 Matplotlib 中 event handling 背后的主要思想是将回调函数连接到事件。在特定事件(例如鼠标单击或按键)发生时,将执行回调函数。此机制使您能够响应用户交互并实现自定义行为。
如果需要,您可以使用从 mpl_connect 方法获取的连接 ID 断开回调。
fig.canvas.mpl_disconnect(cid)
Example
此示例演示了在 Matplotlib 图上打印鼠标点击位置和已按按钮的基本实现。
import matplotlib.pyplot as plt
import numpy as np
# Generate sample data
x = np.linspace(0, 10, 100)
y = np.sin(x)
# Create a Matplotlib figure and axis
fig, ax = plt.subplots(figsize=(7, 4))
# Plot the data
ax.plot(x, y)
# Define a callback function to handle events
def onclick(event):
print('%s click: button=%d, x=%d, y=%d, xdata=%f, ydata=%f' %
('double' if event.dblclick else 'single', event.button,
event.x, event.y, event.xdata, event.ydata))
# Connect the event handler to the figure canvas
cid = fig.canvas.mpl_connect('button_press_event', onclick)
plt.show()
在执行上述程序后,你将获得以下输出 -
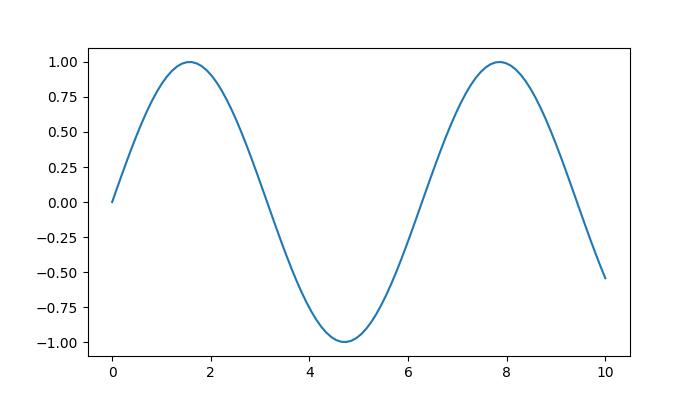
single click: button=1, x=271, y=266, xdata=3.220737, ydata=0.485644
single click: button=1, x=218, y=226, xdata=2.146083, ydata=0.200062
single click: button=3, x=218, y=226, xdata=2.146083, ydata=0.200062
single click: button=1, x=360, y=245, xdata=5.025346, ydata=0.335713
观看下面的视频来观察此示例的工作。

Common Events in Matplotlib
Matplotlib 支持多种事件,每个事件由特定类表示 −
-
button_press_event − 单击鼠标按钮时触发。
-
button_release_event − 松开鼠标按钮时触发。
-
close_event − 关闭图形时触发。
-
draw_event − 已绘制画布时触发,但屏幕小组件未更新时触发。
-
key_press_event − 按下键时触发。
-
key_release_event − 松开键时触发。
-
motion_notify_event − 移动鼠标时触发。
-
pick_event − 选中画布中的艺术家时触发。
-
resize_event − 调整图形画布大小时触发。
-
scroll_event − 滚动鼠标滚轮时触发。
-
figure_enter_event − 鼠标进入新图形时触发。
-
figure_leave_event − 鼠标离开图形时触发。
-
axes_enter_event − 鼠标进入新坐标轴时触发。
-
axes_leave_event − 鼠标离开坐标轴时触发。
通过使用这些事件,你可以在 matplotlib 中创建动态的互动可视化。
Event Attributes
所有 Matplotlib 事件从 matplotlib.backend_bases.Event 类继承,该类具有 name 、 canvas 和 guiEvent 等属性。 MouseEvent 的常见属性包括 x 、 y 、 inaxes 、 xdata 和 ydata 。
Example
我们来看一个简单的例子,在该例子中,每次按下绘图上的鼠标都会生成线段。
from matplotlib import pyplot as plt
import numpy as np
# LineBuilder Class
# It creats line segments based on mouse clicks.
class LineBuilder:
def __init__(self, line):
self.line = line
self.xs = list(line.get_xdata())
self.ys = list(line.get_ydata())
self.cid = line.figure.canvas.mpl_connect('button_press_event', self)
def __call__(self, event):
if event.inaxes != self.line.axes:
return
self.xs.append(event.xdata)
self.ys.append(event.ydata)
self.line.set_data(self.xs, self.ys)
self.line.figure.canvas.draw()
# Create a figure and axis
fig, ax = plt.subplots(figsize=(7, 4))
# Set the title
ax.set_title('Click to Build Line Segments')
# empty line
line, = ax.plot([0], [0])
# Create an instance for LineBuilder class
linebuilder = LineBuilder(line)
# Show the Plot
plt.show()
执行上述程序后你会得到以下图形,点击该图形观察该例子的执行过程 −
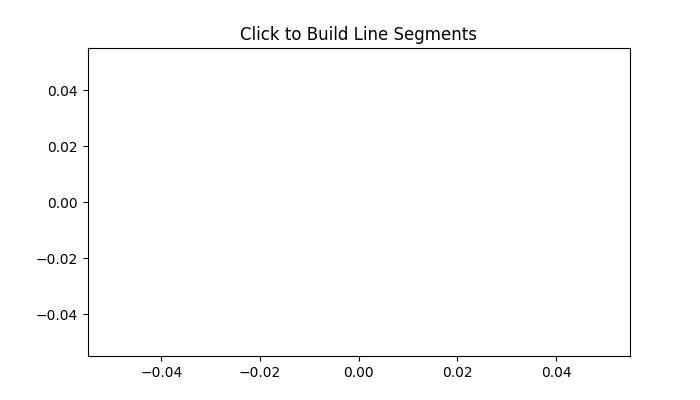
观看下方视频,观察该例子的执行过程。

Detecting the Mouse moves
如果您想检测鼠标何时进入或离开图形或轴,可以连接至图形/轴进入/离开事件。
以下的另外一个示例演示了当鼠标进入或离开图形的特定区域时如何更改边框颜色。
import matplotlib.pyplot as plt
def enter_axes(event):
event.inaxes.patch.set_facecolor('yellow')
event.canvas.draw()
def leave_axes(event):
event.inaxes.patch.set_facecolor('white')
event.canvas.draw()
def enter_figure(event):
event.canvas.figure.patch.set_facecolor('red')
event.canvas.draw()
def leave_figure(event):
event.canvas.figure.patch.set_facecolor('grey')
event.canvas.draw()
fig, axs = plt.subplots(2, figsize=(7, 4))
fig.suptitle('Mouse Hover Over Figure or Axes to Trigger Events')
fig.canvas.mpl_connect('figure_enter_event', enter_figure)
fig.canvas.mpl_connect('figure_leave_event', leave_figure)
fig.canvas.mpl_connect('axes_enter_event', enter_axes)
fig.canvas.mpl_connect('axes_leave_event', leave_axes)
plt.show()
在执行上述程序后,你将获得以下输出 -
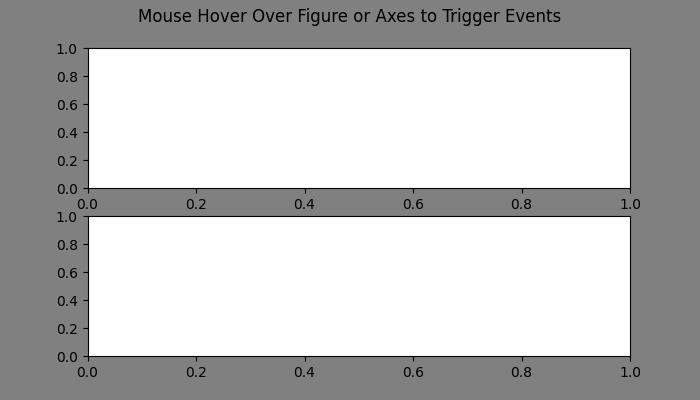
观看下面的视频来观察此示例的工作。

以下的另外一个示例演示了如何使用 Matplotlib 显示鼠标释放事件坐标
from matplotlib import pyplot as plt
plt.rcParams['backend'] = 'TkAgg'
plt.rcParams["figure.figsize"] = [7, 4]
plt.rcParams["figure.autolayout"] = True
# Define a callback function to handle events
def onclick(event):
print(event.button, event.xdata, event.ydata)
# Create a Matplotlib figure and axis
fig, ax = plt.subplots()
# Plot the data
ax.plot(range(10))
# Connect the event handler to the figure canvas
fig.canvas.mpl_connect('button_release_event', onclick)
# Show the Plot
plt.show()
执行上述代码,我们将得到以下输出 −
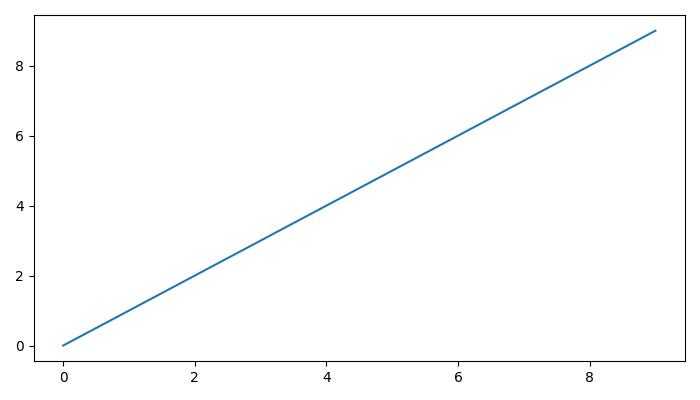
观看下面的视频来观察此示例的工作。
