Matplotlib 简明教程
Matplotlib - Grids
总体而言,数据可视化和绘图, grids 指的是绘图区域上的水平和垂直线集合。网格线有助于更好地理解绘图中的数据。通常,这些线与 x 轴和 y 轴上的主要刻度线对齐。它们可以增强绘图的可读性并使其更容易评估值。
参阅以下示例图像 −

主要有两种类型的网格线 -
-
Major Gridlines - 这些是与坐标轴上的主要刻度线对齐的主要网格线。
-
Minor Gridlines - 这些是主要网格线之间的附加网格线,并与坐标轴上的次要刻度线对齐。
Introduction to Grids in Matplotlib
启用网格线是 Matplotlib 中一个简单的过程。pyplot.grid() 方法将主要网格线添加到绘图中,并提供其他自定义选项,包括调整线型、线宽、颜色和透明度。
让我们探索向绘图中添加网格线的不同方法。
Basic Plot with Grids
在 Matplotlib 中,默认网格是一组与 x 轴和 y 轴上的主要刻度线对齐的主要网格线。
Example
在此示例中,我们创建了一个基本的正弦波绘图并添加了默认网格。
import matplotlib.pyplot as plt
import numpy as np
# Create some data
x = np.linspace(0, 2 * np.pi, 100)
y = np.sin(x)
# create a plot
fig, ax = plt.subplots(figsize=(7,4))
# Plot the data
plt.plot(x, y)
# Add grid
ax.grid(True)
# set the title
ax.set_title('Basic Plot with Grids')
# Show the plot
plt.show()
执行上述代码,我们将得到以下输出 −

Customizing Grid
自定义网格线包括线型、线宽、颜色和透明度。
Example
此示例演示如何通过更改其线型、线宽、颜色和透明度来自定义网格线。
import matplotlib.pyplot as plt
import numpy as np
# Create some data
x = np.arange(0, 1, 0.05)
y = x**2
# Create the plot
fig, ax = plt.subplots(figsize=(7,4))
# Plot the data
plt.scatter(x, y)
# Customize grid
ax.grid(True, linestyle='-.', linewidth=1, color='red', alpha=0.9)
# set the title
ax.set_title('Customizing Grids')
# Show the plot
plt.show()
执行上述代码,我们将得到以下输出 −

Adding Minor Gridlines
Example
此示例演示如何将主网格线和次网格线全部添加到绘图中。
import matplotlib.pyplot as plt
import numpy as np
# Create some data
x = np.arange(0, 1, 0.05)
y = x**2
# Create the plot
fig, ax = plt.subplots(figsize=(7,4))
# Plot the data
plt.scatter(x, y)
# Add major grid
ax.grid(True)
# Add minor grid
ax.minorticks_on()
ax.grid(which='minor', linestyle=':', linewidth=0.5, color='red', alpha=0.5)
# set the title
ax.set_title('Major and Minor Gridlines')
# Show the plot
plt.show()
执行上述代码,我们将得到以下输出 −
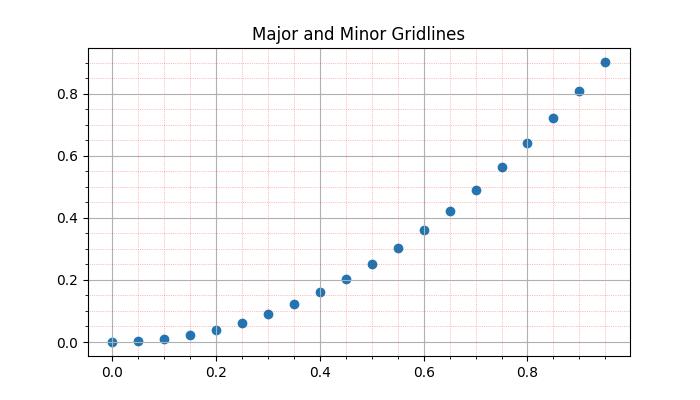
Manually adding the grids
此方法涉及明确指定垂直线和水平线的位置。通过遍历特定的间隔或值,用户可以在所需位置绘制网格线。这涉及使用类似 pyplot.axvline() 和 pyplot.axhline() 的函数分别绘制垂直线和水平线。
Example
以下是一个手动在 x 轴上每三个点绘制垂直网格线的示例。
import matplotlib.pyplot as plt
import numpy as np
# Create some data
x = np.arange(0, 1, 0.05)
y = x**2
# Create the plot
plt.subplots(figsize=(7,4))
# Plot the data
plt.scatter(x, y)
# Set x and y tick locations
plt.xticks(np.arange(0, 1.01, 0.1))
plt.yticks(np.arange(0, 1.01, 0.1))
plt.title('Manually Drawing the Grids ')
# Draw grid lines for every third point on the x-axis
for pt in np.arange(0, 1.01, 0.3):
plt.axvline(pt, lw=0.5, color='black', alpha=0.5)
# Show the plot
plt.show()
执行上述代码,我们将得到以下输出 −

Hiding the gridlines
可以通过向 grid() 函数指定布尔值 False 来隐藏或删除绘图中的网格线。
Example
以下是一个隐藏绘图的网格线和轴(X 轴和 Y 轴)的示例。
import numpy as np
import matplotlib.pyplot as plt
# Create a figure
fig = plt.figure(figsize=(7, 4))
# Generate data
x = np.linspace(-10, 10, 50)
y = np.sin(x)
# Plot horizontal line
plt.axhline(y=0, c="green", linestyle="dashdot", label="y=0")
# Plot sine curve
plt.plot(x, y, c="red", lw=5, linestyle="dashdot", label="y=sin(x)")
# Hide gridlines
plt.grid(False)
# Hide axes
plt.axis('off')
# Add legend
plt.legend()
# Show plot
plt.show()
执行上述代码时,您将获得以下输出 -
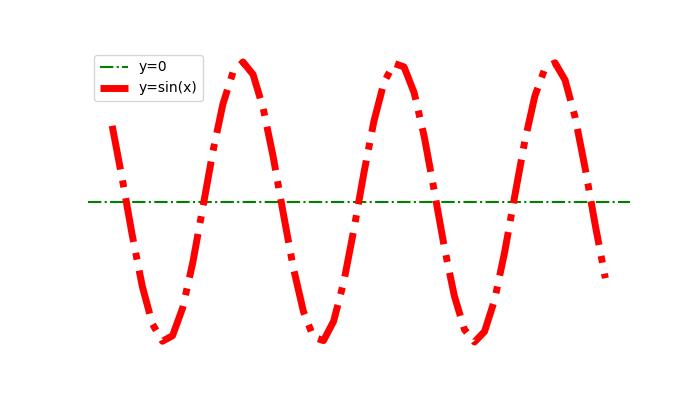
Gridlines Across The Subplots
在多个子图中比较数据时,跨所有子图绘制网格线很有用,以在绘图之间保持可视比较。
Example
以下是一个演示如何跨子图绘制网格线的示例。
import matplotlib.pyplot as plt
# Data
d = [1, 2, 3, 4, 5, 6, 7, 8, 9]
f = [0, 1, 0, 0, 1, 0, 1, 1, 0]
# Create figure and subplots
fig = plt.figure(figsize=(7,4))
fig.set_size_inches(30, 10)
ax1 = fig.add_subplot(211)
ax2 = fig.add_subplot(212)
# Plot data on subplots
ax1.plot(d, marker='.', color='b', label="1 row")
# Draw grid lines behind bar graph
ax2.bar(range(len(d)), d, color='red', alpha=0.5)
# Enable grids on both subplots
ax1.grid()
ax2.grid()
# Display the plot
plt.show()
执行上述代码时,您将获得以下输出 -
