Matplotlib 简明教程
Matplotlib - Tick Locators
在一般图形和绘图中,刻度在通过小线表示 x 和 y 轴的刻度方面发挥着至关重要的作用,从而清楚地指示相关的值。 Tick locators 另一方面,定义了这些刻度沿轴的位置,提供了刻度的可视表示。
下图表示图形上的主刻度和次刻度 −
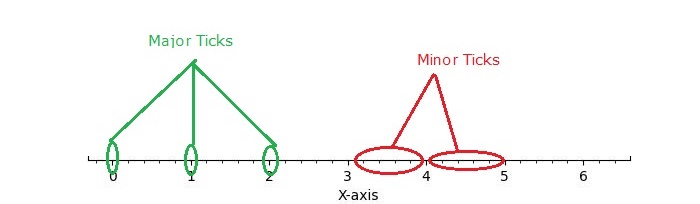
Tick Locators in Matplotlib
matplotlib 通过其刻度定位器提供了一种控制刻度在轴上定位的机制。 matplotlib.ticker 模块包含用于配置刻度定位和格式化的类。这些类包括通用刻度定位器、格式化程序和特定于域的自定义定位器。虽然定位器不知道主刻度或次刻度,但它们被 Axis 类用于支持主刻度和次刻度定位和格式化。
Different Tick Locators
matplotlib 在其 ticker 模块中提供了不同的刻度定位器,允许用户自定义轴上的刻度位置。一些刻度定位器包括 −
-
AutoLocator
-
MaxNLocator
-
LinearLocator
-
LogLocator
-
MultipleLocator
-
FixedLocator
-
IndexLocator
-
NullLocator
-
SymmetricalLogLocator
-
AsinhLocator
-
LogitLocator
-
AutoMinorLocator
-
Defining Custom Locators
Basic Setup
在深入研究具体刻度定位器之前,我们来建立一个通用的设置函数,用刻度绘制绘图。
import matplotlib.pyplot as plt
import numpy as np
import matplotlib.ticker as ticker
def draw_ticks(ax, title):
# it shows the bottom spine only
ax.yaxis.set_major_locator(ticker.NullLocator())
ax.spines[['left', 'right', 'top']].set_visible(False)
ax.xaxis.set_ticks_position('bottom')
ax.tick_params(which='major', width=1.00, length=5)
ax.tick_params(which='minor', width=0.75, length=2.5)
ax.set_xlim(0, 5)
ax.set_ylim(0, 1)
ax.text(0.0, 0.2, title, transform=ax.transAxes,
fontsize=14, fontname='Monospace', color='tab:blue')
现在,让我们探讨每个刻度定位器的作用。
Auto Locator
AutoLocator 和 AutoMinorLocator 分别用于自动确定轴上的主刻度和次刻度的位置。
Example
此示例演示如何使用 AutoLocator 和 AutoMinorLocator 自动处理轴上的主刻度和次刻度的定位。
# Auto Locator
fig, ax = plt.subplots(1,1,figsize=(7,1.5), facecolor='#eaffff')
plt.subplots_adjust(bottom=0.3, top=0.6, wspace=0.2, hspace=0.4)
draw_ticks(ax, title="AutoLocator() and AutoMinorLocator()")
ax.xaxis.set_major_locator(ticker.AutoLocator())
ax.xaxis.set_minor_locator(ticker.AutoMinorLocator())
ax.set_title('Auto Locator and Auto Minor Locator')
plt.show()
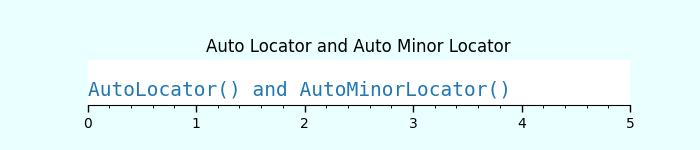
Null Locator
NullLocator 在轴上不放置刻度。
Example
我们来看以下 NullLocator 工作示例。
# Null Locator
fig, ax = plt.subplots(1,1,figsize=(7,1.5), facecolor='#eaffff')
plt.subplots_adjust(bottom=0.3, top=0.6, wspace=0.2, hspace=0.4)
draw_ticks(ax, title="NullLocator()")
ax.xaxis.set_major_locator(ticker.NullLocator())
ax.xaxis.set_minor_locator(ticker.NullLocator())
ax.set_title('Null Locator (No ticks)')
plt.show()
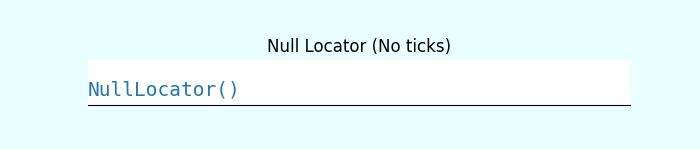
Multiple Locator
MultipleLocator() 类允许以指定基数的倍数放置刻度,同时支持整型和浮点值。
Example
以下示例演示了 MultipleLocator() 类的使用方法。
# Multiple Locator
fig, ax = plt.subplots(1,1,figsize=(7,1.5), facecolor='#eaffff')
plt.subplots_adjust(bottom=0.3, top=0.6, wspace=0.2, hspace=0.4)
draw_ticks(ax, title="MultipleLocator(0.5)")
ax.xaxis.set_major_locator(ticker.MultipleLocator(0.5))
ax.xaxis.set_minor_locator(ticker.MultipleLocator(0.1))
ax.set_title('Multiple Locator')
plt.show()
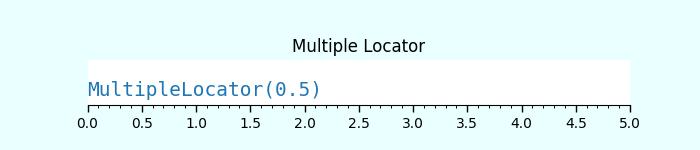
Fixed Locator
FixedLocator() 将刻度放置在指定固定位置。
Example
以下是如何使用 FixedLocator() 类的示例。
# Fixed Locator
fig, ax = plt.subplots(1,1,figsize=(7,1.5), facecolor='#eaffff')
plt.subplots_adjust(bottom=0.3, top=0.6, wspace=0.2, hspace=0.4)
draw_ticks(ax, title="FixedLocator([0, 1, 3, 5])")
ax.xaxis.set_major_locator(ticker.FixedLocator([0, 1, 3, 5]))
ax.xaxis.set_minor_locator(ticker.FixedLocator(np.linspace(0.2, 0.8, 4)))
ax.set_title('Fixed Locator')
plt.show()
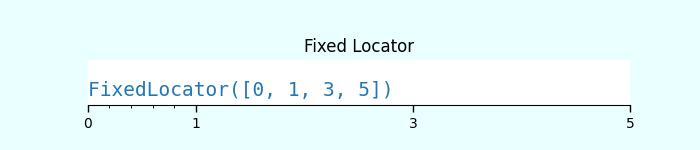
Linear Locator
LinearLocator 在最大值和最小值之间均匀分布刻度。
Example
以下示例应用线性定位器到轴线的主刻度和次刻度。
# Linear Locator
fig, ax = plt.subplots(1,1,figsize=(7,1.5), facecolor='#eaffff')
plt.subplots_adjust(bottom=0.3, top=0.6, wspace=0.2, hspace=0.4)
draw_ticks(ax, title="LinearLocator(numticks=3)")
ax.xaxis.set_major_locator(ticker.LinearLocator(3))
ax.xaxis.set_minor_locator(ticker.LinearLocator(10))
ax.set_title('Linear Locator')
plt.show()
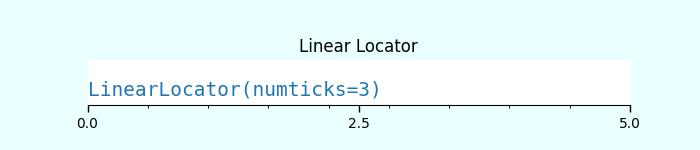
Index Locator
该定位器适用于 x = range(len(y)) 的索引图。
Example
以下示例使用了索引定位器 ( ticker.IndexLocator() 类)。
# Index Locator
fig, ax = plt.subplots(1,1,figsize=(7,1.5), facecolor='#eaffff')
plt.subplots_adjust(bottom=0.3, top=0.6, wspace=0.2, hspace=0.4)
draw_ticks(ax, title="IndexLocator(base=0.5, offset=0.25)")
ax.plot([0]*5, color='white')
ax.xaxis.set_major_locator(ticker.IndexLocator(base=0.5, offset=0.25))
ax.set_title('Index Locator')
plt.show()
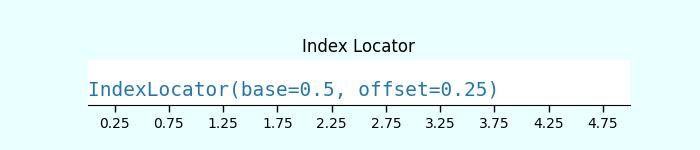
MaxN Locator
Example
以下示例演示如何将 MaxNLocator() 类用于主刻度和次刻度。
# MaxN Locator
fig, ax = plt.subplots(1,1,figsize=(7,1.5), facecolor='#eaffff')
plt.subplots_adjust(bottom=0.3, top=0.6, wspace=0.2, hspace=0.4)
draw_ticks(ax, title="MaxNLocator(n=4)")
ax.xaxis.set_major_locator(ticker.MaxNLocator(4))
ax.xaxis.set_minor_locator(ticker.MaxNLocator(40))
ax.set_title('MaxN Locator')
plt.show()
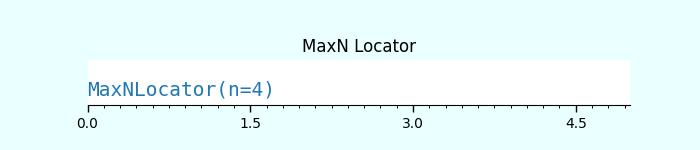
Log Locator
LogLocator 用于以从最小值到最大值的对数方式分布刻度。
Example
我们来看一个使用对数定位器的示例。它显示的对数刻度的次刻度标签。
# Log Locator
fig, ax = plt.subplots(1,1,figsize=(7,1.5), facecolor='#eaffff')
plt.subplots_adjust(bottom=0.3, top=0.6, wspace=0.2, hspace=0.4)
draw_ticks(ax, title="LogLocator(base=10, numticks=15)")
ax.set_xlim(10**3, 10**10)
ax.set_xscale('log')
ax.xaxis.set_major_locator(ticker.LogLocator(base=10, numticks=15))
ax.set_title('Log Locator')
plt.show()
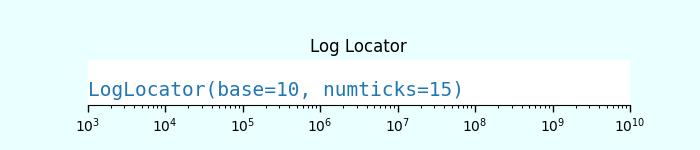