Matplotlib 简明教程
Matplotlib - Image Thumbnail
Thumbnails ,原始图像的较小且压缩版本,是各种应用程序中不可或缺的组件,包括图像预览、Web 开发,以及优化包含繁重图像的网页和应用程序的加载速度。
Thumbnails, smaller and compressed versions of original images, serve as essential components in various applications, including image previews, web development, and optimizing the loading speed of web pages and applications with heavy images.
在本教程中,我们将探讨使用 Matplotlib 来有效地生成图像缩略图。Matplotlib 采用了 Python Pillow library 来进行图像处理,并允许我们轻松地从现有图像生成缩略图。
In this tutorial, we’ll explore using Matplotlib to efficiently generate image thumbnails. Matplotlib takes the support of the Python Pillow library for image processing and allows us to easily generate thumbnails from existing images.
Image thumbnail in Matplotlib
Matplotlib 在其图像模块中提供了 thumbnail() 函数来生成具有可自定义参数的缩略图,使用户能够有效地创建不同目的的图像的缩小版本。
Matplotlib offers the thumbnail() function within its image module to generate thumbnails with customizable parameters, allowing users to efficiently create scaled-down versions of images for various purposes.
以下是函数的语法−
Following is the syntax of the function −
Syntax
matplotlib.image.thumbnail(infile, thumbfile, scale=0.1, interpolation='bilinear', preview=False)
以下是其参数的详细信息 -
Here are the details of its parameters −
-
infile − The input image file. As you know that Matplotlib relies on Pillow for image reading, so it supports a wide range of file formats, including PNG, JPG, TIFF, and others.
-
thumbfile − The filename or file-like object where the thumbnail will be saved.
-
scale − The scale factor for the thumbnail. It determines the size reduction of the thumbnail relative to the original image. A smaller scale factor generates a smaller thumbnail.
-
interpolation − The interpolation scheme used in the resampling process. This parameter specifies the method used to estimate pixel values in the thumbnail.
-
preview − If set to True, the default backend (presumably a user interface backend) will be used, which may raise a figure if show() is called. If set to False, the figure is created using FigureCanvasBase, and the drawing backend is selected as Figure.savefig would normally do.
函数返回一个包含缩略图的 Figure 实例。这个 Figure 对象可以根据需要进一步处理或保存。
The function returns a Figure instance containing the thumbnail. This Figure object can be further manipulated or saved as needed.
Generating Thumbnails for a single image
Matplotlib 支持 png、pdf、ps、eps svg 等多种图像格式,使其可以灵活适用于不同的用例。
Matplotlib supports various image formats like png, pdf, ps, eps svg, and more, making it flexible for different use cases.
Example
以下是一个为单个 .jpg 图像创建缩略图的示例。
Here is an example that creates a thumbnail for a single .jpg image.
import matplotlib.pyplot as plt
import matplotlib.image as mpimg
input_image_path = "Images/Tajmahal.jpg"
output_thumbnail_path = "Images/Tajmahal_thumbnail.jpg"
# Load the original image
img = mpimg.imread(input_image_path)
# Create a thumbnail using Matplotlib
thumb = mpimg.thumbnail(input_image_path, output_thumbnail_path, scale=0.15)
print(f"Thumbnail generated for {input_image_path}. Saved to {output_thumbnail_path}")
如果您访问保存图像的文件夹,则可以看到原始缩略图和输出缩略图,如下所示:
If you visit the folder where the images are saved you can observe both the original and output Thumbnail images as shown below −
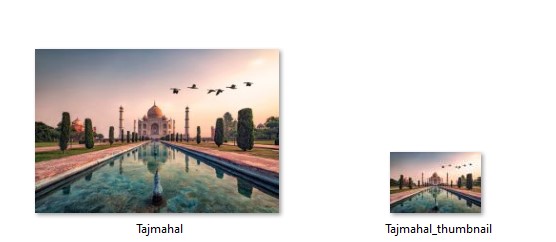
Generating Thumbnails for multiple Images
考虑一种场景,在该场景中,您在目录中有多个图像,并且您想为每个图像生成缩略图。
Consider a scenario where you have multiple images in a directory, and you want to generate thumbnails for each of them.
Example
以下是一个在目录中为多个 PNG 图像创建缩略图的示例。将以下脚本另存为 generate_thumbnails.py 文件。
Here is an example that creates thumbnails of multiple PNG images in a directory. Save the following script as generate_thumbnails.py file.
from argparse import ArgumentParser
from pathlib import Path
import sys
import matplotlib.image as image
parser = ArgumentParser(description="Generate thumbnails of PNG images in a directory.")
parser.add_argument("imagedir", type=Path)
args = parser.parse_args()
if not args.imagedir.is_dir():
sys.exit(f"Could not find the input directory {args.imagedir}")
outdir = Path("thumbs")
outdir.mkdir(parents=True, exist_ok=True)
for path in args.imagedir.glob("*.png"):
outpath = outdir / path.name
try:
fig = image.thumbnail(path, outpath, scale=0.15)
print(f"Saved thumbnail of {path} to {outpath}")
except Exception as e:
print(f"Error generating thumbnail for {path}: {e}")
然后在命令提示符中按以下方式运行脚本:
Then run the script as follows in command prompt
python generate_thumbnails.py /path/to/all_images
在执行上述程序时,将创建一个名为“thumbs”的目录,并在您的工作目录中为 PNG 图像生成缩略图。如果它遇到格式不同的图像,它将打印一条错误消息并继续处理其他图像。
On executing the above program, will create a directory named "thumbs" and generate thumbnails for PNG images in your working directory. If it encounters an image with a different format, it will print an error message and continue processing other images.
Saved thumbnail of Images\3d_Star.png to thumbs\3d_Star.png
Saved thumbnail of Images\balloons_noisy.png to thumbs\balloons_noisy.png
Saved thumbnail of Images\binary image.png to thumbs\binary image.png
Saved thumbnail of Images\black and white.png to thumbs\black and white.png
Saved thumbnail of Images\Black1.png to thumbs\Black1.png
Saved thumbnail of Images\Blank.png to thumbs\Blank.png
Saved thumbnail of Images\Blank_img.png to thumbs\Blank_img.png
Saved thumbnail of Images\Circle1.png to thumbs\Circle1.png
Saved thumbnail of Images\ColorDots.png to thumbs\ColorDots.png
Saved thumbnail of Images\colorful-shapes.png to thumbs\colorful-shapes.png
Saved thumbnail of Images\dark_img1.png to thumbs\dark_img1.png
Saved thumbnail of Images\dark_img2.png to thumbs\dark_img2.png
Saved thumbnail of Images\decore.png to thumbs\decore.png
Saved thumbnail of Images\deform.png to thumbs\deform.png
Saved thumbnail of Images\Different shapes.png to thumbs\Different shapes.png
Error generating thumbnail for Images\Different shapes_1.png: not a PNG file
Saved thumbnail of Images\Ellipses.png to thumbs\Ellipses.png
Error generating thumbnail for Images\Ellipses_and_circles.png: not a PNG file
Saved thumbnail of Images\images (1).png to thumbs\images (1).png
Saved thumbnail of Images\images (3).png to thumbs\images (3).png
Saved thumbnail of Images\images.png to thumbs\images.png
Saved thumbnail of Images\image___1.png to thumbs\image___1.png
Saved thumbnail of Images\Lenna.png to thumbs\Lenna.png
Saved thumbnail of Images\logo-footer-b.png to thumbs\logo-footer-b.png
Saved thumbnail of Images\logo-w.png to thumbs\logo-w.png
Saved thumbnail of Images\logo.png to thumbs\logo.png
Saved thumbnail of Images\logo_Black.png to thumbs\logo_Black.png