Matplotlib 简明教程
Matplotlib - Multipage PDF
multipage PDF (便携式文档格式)是一种文件类型,可以在单个文档中存储多个页面或图像。PDF 内的每个页面可以有不同的内容,如绘图、图像或文本。
A multipage PDF(Portable Document Format) is a type of file that can store multiple pages or images in a single document. Each page within the PDF can have different content, such as plots, images, or text.
Matplotlib 通过 backend_pdf.PdfPages 模块支持创建多页 PDF。此功能允许用户在同一 PDF 文件中的多个页面中保存绘图和可视化效果。
Matplotlib provides support for creating multipage PDFs through its backend_pdf.PdfPages module. This feature allows users to save plots and visualizations across multiple pages within the same PDF file.
在某些情况下,需要将几个绘图保存在一个文件中。虽然许多图像文件格式如 PNG、SVG 或 JPEG 通常每个文件仅支持一个图像,但 Matplotlib 提供了一种创建多页输出的解决方案。PDF 是一种受支持的格式,允许用户有效组织和共享可视化效果。
In certain situations, it becomes necessary to save several plots in one file. While many image file formats like PNG, SVG, or JPEG typically support only a single image per file, Matplotlib provides a solution for creating multipage output. PDF is one such supported format, allowing users to organize and share visualizations effectively.
Creating a Basic multipage PDF
要使用 Matplotlib 在 PDF 文档中将绘图保存在多个页面中,可以使用 PdfPages 类。此类简化了生成包含多个页面的 PDF 文件的过程,每个页面都包含不同的可视化效果。
To save plots on multiple pages in a PDF document using Matplotlib, you can make use of the PdfPages class. This class simplifies the process of generating a PDF file with several pages, each containing different visualizations.
Example
让我们从一个基本示例开始,演示如何使用 Matplotlib 创建多页 PDF。此示例一次将多个图形保存在一个 PDF 文件中。
Let’s start with a basic example demonstrating how to create a multipage PDF with Matplotlib. This example saves multiple figures in one PDF file at once.
from matplotlib.backends.backend_pdf import PdfPages
import numpy as np
import matplotlib.pyplot as plt
# sample data for plots
x1 = np.arange(10)
y1 = x1**2
x2 = np.arange(20)
y2 = x2**2
# Create a PdfPages object to save the pages
pp = PdfPages('Basic_multipage_pdf.pdf')
def function_plot(X,Y):
plt.figure()
plt.clf()
plt.plot(X,Y)
plt.title('y vs x')
plt.xlabel('x axis', fontsize = 13)
plt.ylabel('y axis', fontsize = 13)
pp.savefig()
# Create and save the first plot
function_plot(x1,y1)
# Create and save the second plot
function_plot(x2,y2)
pp.close()
执行上述程序后,将在脚本保存的目录中生成“Basic_multipage_pdf.pdf”。
On executing the above program, 'Basic_multipage_pdf.pdf' will be generated in the directory where the script is saved.
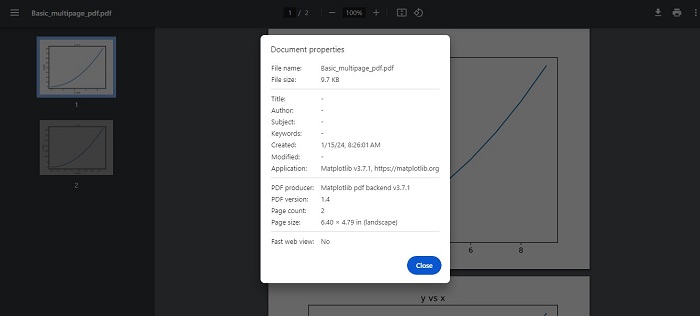
Adding Metadata and Annotations
Matplotlib 还支持向多页 PDF 添加元数据和注释。元数据可以包括标题、作者和创建日期等信息,提供有关 PDF 内内容的其他上下文或详细信息。
Matplotlib also supports the addition of metadata and annotations to a multipage PDF. Metadata can include information such as the title, author, and creation date, providing additional context or details about the content within the PDF.
Example
这是一个高级示例,它创建了一个包含元数据和注释的多页 PDF。
Here is an advanced example, that creates a multipage PDF with metadata and annotations.
import datetime
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.backends.backend_pdf import PdfPages
# Create the PdfPages object to save the pages
with PdfPages('Advanced_multipage_pdf.pdf') as pdf:
# Page One
plt.figure(figsize=(3, 3))
plt.plot(range(7), [3, 1, 4, 1, 5, 9, 2], 'r-o')
plt.title('Page One')
# saves the current figure into a pdf page
pdf.savefig()
plt.close()
# Page Two
# Initially set it to True. If LaTeX is not installed or an error is caught, change to `False`
# The usetex setting is particularly useful when you need LaTeX features that aren't present in matplotlib's built-in mathtext.
plt.rcParams['text.usetex'] = False
plt.figure(figsize=(8, 6))
x = np.arange(0, 5, 0.1)
plt.plot(x, np.sin(x), 'b-')
plt.title('Page Two')
# attach metadata (as pdf note) to page
pdf.attach_note("plot of sin(x)")
pdf.savefig()
plt.close()
# Page Three
plt.rcParams['text.usetex'] = False
fig = plt.figure(figsize=(4, 5))
plt.plot(x, x ** 2, 'ko')
plt.title('Page Three')
pdf.savefig(fig)
plt.close()
# Set file metadata
d = pdf.infodict()
d['Title'] = 'Multipage PDF Example'
d['Author'] = 'Tutorialspoint'
d['Subject'] = 'How to create a multipage pdf file and set its metadata'
d['Keywords'] = 'PdfPages multipage keywords author title subject'
d['CreationDate'] = datetime.datetime(2024, 1, 15)
d['ModDate'] = datetime.datetime.today()
执行上述程序后,将在脚本保存的目录中生成“Advanced_multipage_pdf.pdf”。您将能够观察以下细节-
On executing the above program, 'Advanced_multipage_pdf.pdf' will be generated in the directory where the script is saved. you will be able to observe the details like below −
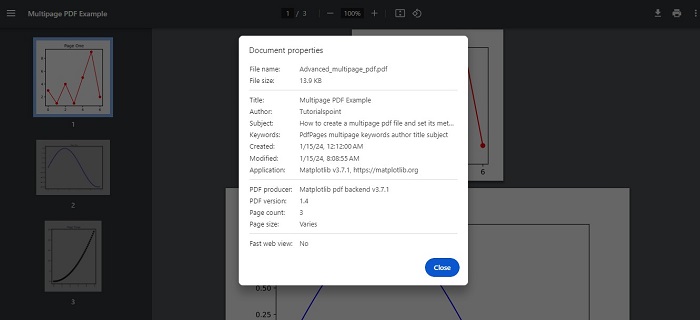