Matplotlib 简明教程
Matplotlib - Font Properties
What are Font properties?
在 Matplotlib 库中,字体属性是决定绘图和图形中文本元素外观和样式的属性。这些属性包括各种方面,如字体系列、大小、粗细、样式和影响文本可视化呈现的其他设置。
In Matplotlib library font properties are attributes that determine the appearance and styling of text elements within plots and figures. These properties include various aspects such as font family, size, weight, style and other settings that affect the visual presentation of text.
Key Font Properties in Matplotlib
Font Family
字体系列指定用于文本元素的字体类型。常见的系列包括衬线体、无衬线体、等宽字体等。
The Font family specifies the typeface or used for text elements. Common families include serif, sans-serif, monospace etc.
-
serif − Fonts with decorative strokes often used for a more traditional or formal appearance.
-
sans-serif − Fonts without decorative strokes known for a clean and modern look which are commonly used for readability.
-
monospace − Fonts where each character occupies the same amount of horizontal space, often used for code or tabular data.
-
Custom or Specific Fonts − Users can also use custom fonts installed on their system or provide font paths for specific typefaces.
Font Size
字体大小决定文本大小(以磅为单位 (pt)),从而影响可读性和可见性。字体大小以磅位(磅)指定,其中 1 磅大约为 1/72 英寸。Matplotlib 将磅作为字体大小的标准单位,从而在不同的设备和显示分辨率之间保持一致性。
The Font size determines the size of the text in points (pt) influencing readability and visibility. Font size is specified in points (pt) where 1 point is approximately 1/72 inch. Matplotlib uses points as a standard unit for font size by allowing for consistency across different devices and display resolutions.
Font Weight
字体粗细控制文本的粗细或加粗程度。选项范围从普通到粗体。它允许用户控制标签、标题、注释和其他文本组件等文本元素的可视化重点。
The Font weight controls the thickness or boldness of the text. Options range from normal to bold. It allows users to control the visual emphasis of text elements such as labels, titles, annotations and other textual components.
Font Weight Options
-
normal − Specifies normal font weight.
-
bold − Specifies a bold font weight.
-
Integer Values − Some fonts support numeric values ranging from 100 to 900 to specify the weight level.
Setting Font Properties in Matplotlib
以下是在 matplotlib 中设置字体属性的方法。
The following are the ways to set the font properties in matplotlib.
Global Configuration (using plt.rcParams)
这用于配置图或图形中所有文本元素的默认字体属性。
This is used to configure default font properties for all text elements in a plot or figure.
import matplotlib.pyplot as plt
# Set global font properties
x = [2,3,4,6]
y = [9,2,4,7]
plt.rcParams['font.family'] = 'sans-serif'
plt.rcParams['font.size'] = 8
plt.rcParams['font.weight'] = 'normal'
plt.rcParams['font.style'] = 'italic'
plt.plot(x,y)
plt.xlabel("x-axis")
plt.ylabel("y-axis")
plt.title("Setting fonts globally")
plt.show()

Individual Text Elements
通过此方法,我们可以为图中的特定文本元素设置字体属性。
Through this method we can set font properties for specific text elements within a plot.
import matplotlib.pyplot as plt
# Set Individual font properties
x = [2,3,4,6]
y = [9,2,4,7]
plt.plot(x,y)
plt.xlabel('X-axis Label', fontsize=14, fontweight='bold', fontstyle='italic')
plt.ylabel('Y-axis Label', fontsize=14, fontweight='bold', fontstyle='normal')
plt.title("Setting fonts Individually")
plt.show()

Importance of Font Properties
以下是字体属性的重要性。
The below are the importance of the font properties.
Readability
正确的字体选择和大小可以提高文本元素的可读性。
Proper font selection and size enhance the legibility of text elements.
Using Font Properties for Customization
-
Adjusting font properties allows users to tailor the appearance of text to match the requirements of the visualization or presentation.
-
Consistent and appropriate use of font properties ensures a visually cohesive and informative display of textual information within plots and figures.
最后,我们可以在 Matplotlib 库中使用的字体属性为用户提供了自定义文本外观的灵活性,确保可视化中的清晰度、可读性和视觉吸引力。这些属性可以精确控制文本元素在图中显示的方式,从而帮助有效地向查看者传达信息。
Finally we can font properties in Matplotlib library provide users with the flexibility to customize text appearance, ensuring clarity, readability and visual appeal in visualizations. These properties enable precise control over how text elements are displayed within plots helping effectively communicate information to viewers.
Multiple font sizes in one label
在此示例中,我们在 Python 中使用 title() 中的 fontsize 参数在一个标签中使用多个字体大小。
In this example we use multiple font sizes in one label in Python with the help of fontsize parameter in title() method.
import numpy as np
from matplotlib import pyplot as plt
plt.rcParams["figure.figsize"] = [7.50, 3.50]
plt.rcParams["figure.autolayout"] = True
x = np.linspace(-5, 5, 100)
y = np.cos(x)
plt.plot(x, y)
fontsize = 20
plt.title("$bf{y=cos(x)}$", fontsize=fontsize)
plt.axis('off')
plt.show()
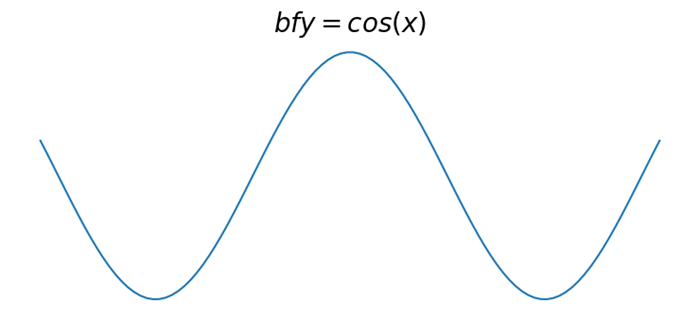
Change the text color of font in the legend
在此示例中,我们将更改图例中字体的文本颜色。
Here in this example we will change the text color of the font in the legend.
import numpy as np
from matplotlib import pyplot as plt
plt.rcParams["figure.figsize"] = [7.00, 3.50]
plt.rcParams["figure.autolayout"] = True
x = np.linspace(-2, 2, 100)
y = np.exp(x)
plt.plot(x, y, label="y=exp(x)", c='red')
leg = plt.legend(loc='upper left')
for text in leg.get_texts():
text.set_color("green")
plt.show()
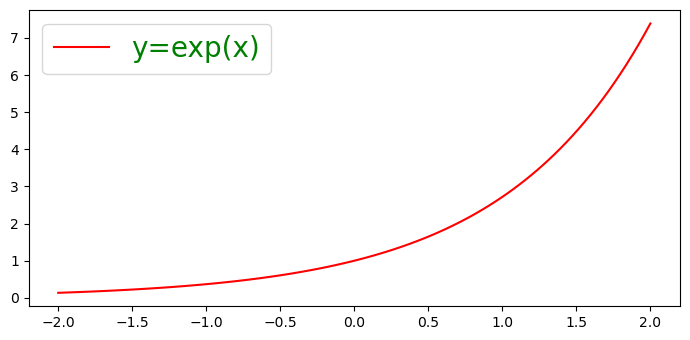
Change the default font color for all text
在此示例中,我们将更改图中所有文本的默认字体颜色。
In this example we will change the default font color for all the text in the plot.
import matplotlib.pyplot as plt
plt.rcParams["figure.figsize"] = [7.50, 3.50]
plt.rcParams["figure.autolayout"] = True
print("Default text color is: ", plt.rcParams['text.color'])
plt.rcParams.update({'text.color': "red",
'axes.labelcolor': "green"})
plt.title("Title")
plt.xlabel("X-axis")
plt.show()
默认文本颜色:黑色
Default text color is: black
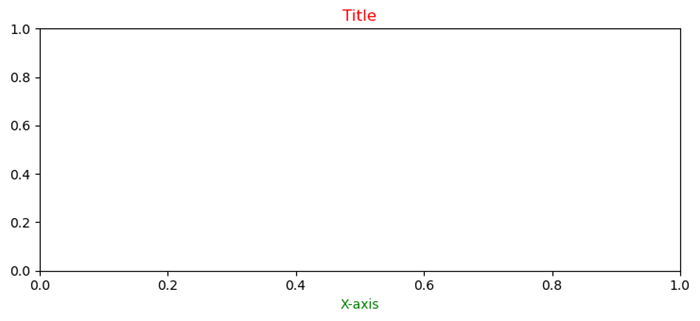
Change the font size of ticks of axes object
在此示例中,我们将更改图中所有文本的默认字体颜色。
In this example we will change the default font color for all the text in the plot.
import numpy as np
from matplotlib import pyplot as plt
plt.rcParams["figure.figsize"] = [7.00, 3.50]
plt.rcParams["figure.autolayout"] = True
x = np.linspace(-2, 2, 10)
y = np.sin(x)
fig, ax = plt.subplots()
ax.plot(x, y, c='red', lw=5)
ax.set_xticks(x)
for tick in ax.xaxis.get_major_ticks():
tick.label.set_fontsize(14)
tick.label.set_rotation('45')
plt.tight_layout()
plt.show()
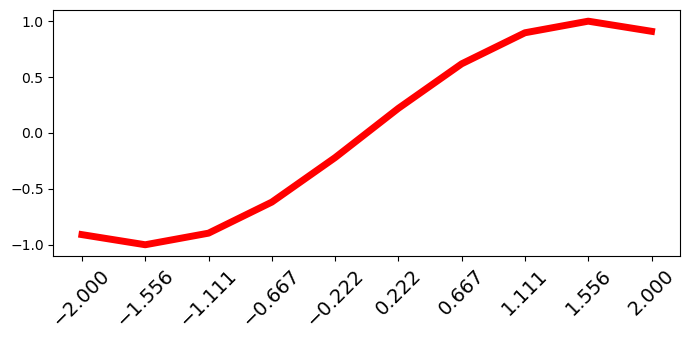
Increase the font size of the seaborn plot legend
在此示例中,我们增大了 Seaborn 图中图例的字体大小,可以使用 fontsize 变量并可以在 legend() 方法参数中使用它。
In this example we increase the font size of the legend in a Seaborn plot, we can use the fontsize variable and can use it in legend() method argument.
import pandas
import matplotlib.pylab as plt
import seaborn as sns
plt.rcParams["figure.figsize"] = [7.00, 3.50]
plt.rcParams["figure.autolayout"] = True
df = pandas.DataFrame(dict(
number=[2, 5, 1, 6, 3],
count=[56, 21, 34, 36, 12],
select=[29, 13, 17, 21, 8]
))
bar_plot1 = sns.barplot(x='number', y='count', data=df, label="count", color="red")
bar_plot2 = sns.barplot(x='number', y='select', data=df, label="select", color="green")
fontsize = 20
plt.legend(loc="upper right", frameon=True, fontsize=fontsize)
plt.show()
