Matplotlib 简明教程
Matplotlib - Timers
在通用的计算机编程中, timers 指的是允许用户在预定义的时间间隔内安排执行特定任务或代码片段的机制。计时器对于各种应用程序都很有用,它可以实现重复操作、定期更新或基于时间相关条件触发事件的自动化。
Timers in Matplotlib
Matplotlib 计时器是强大的功能,它使您能够将定期事件集成到图表中。并且被设计为独立于特定图形用户界面 (GUI) 后端工作。
若要利用 Matplotlib 计时器功能, figure.canvas.new_timer() 函数将作为集成功能与各种 GUI 事件循环的关键组件。尽管它的调用签名可能看起来不太习惯,但由于这种理解,调用签名至关重要(如果你调用的函数不使用参数或关键字参数,则需要明确指定空的序列和字典)。
以下是语法 −
Syntax
timer = figure.canvas.new_timer(interval=5000, callbacks=[(callback_function, [], {})])
timer.start()
该语法创建了一个间隔为 5 秒的计时器,演示了如何将计时器集成到 Matplotlib 的图表中。
Example
以下是一个示例,演示了在 Matplotlib 中简单使用计时器。它设置了一个计时器,每 5 秒在控制台上打印“Matplotlib Timer Event”。这展示了如何将计时器用于图表中的周期性任务。
import matplotlib.pyplot as plt
# Function to handle the timer event
def handle_timer_event():
print('Matplotlib Timer Event')
# Create a new Matplotlib figure and axis
custom_fig, custom_ax = plt.subplots(figsize=(7, 4))
# Create a timer with a 5000 milliseconds interval
custom_timer = custom_fig.canvas.new_timer(interval=5000, callbacks=[(handle_timer_event, [], {})])
# Start the timer
custom_timer.start()
plt.show()
在执行上述程序后,你将获得以下输出 -
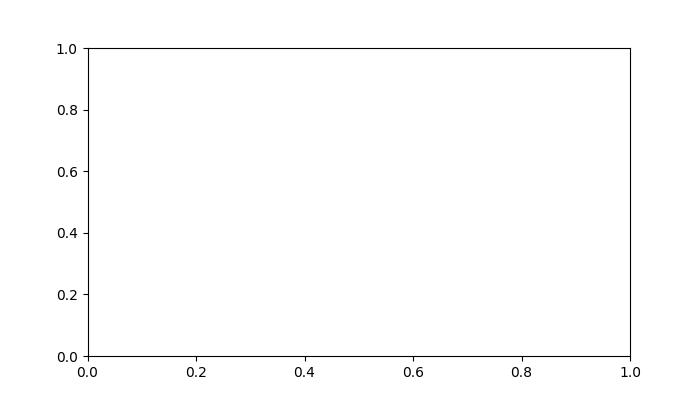
Matplotlib Timer Event
Matplotlib Timer Event
Matplotlib Timer Event
Matplotlib Timer Event
Matplotlib Timer Event
Matplotlib Timer Event
观看下面的视频来观察此示例的工作。

Timer for Real-Time Updates
计时器可用于在图表内实现实时更新,从而增强可视化的动态特性。
Example
在该示例中,一个计时器用于使用当前时间戳以 500 毫秒的间隔更新数字的标题。它显示了如何将计时器用于可视化中的动态、受时间制约的更新。
from datetime import datetime
import matplotlib.pyplot as plt
import numpy as np
# Function to update the title with the current timestamp
def update_title(axes):
axes.set_title(datetime.now())
axes.figure.canvas.draw()
# Create a Matplotlib figure and axis
fig, ax = plt.subplots(figsize=(7, 4))
# Generate sample data
x = np.linspace(0, 10, 100)
ax.plot(x, np.sin(x))
# Create a new timer with an interval of 500 milliseconds
timer = fig.canvas.new_timer(interval=500)
# Add the update_title function as a callback to the timer
timer.add_callback(update_title, ax)
# Start the timer
timer.start()
plt.show()
在执行上述程序后,你将获得以下输出 -
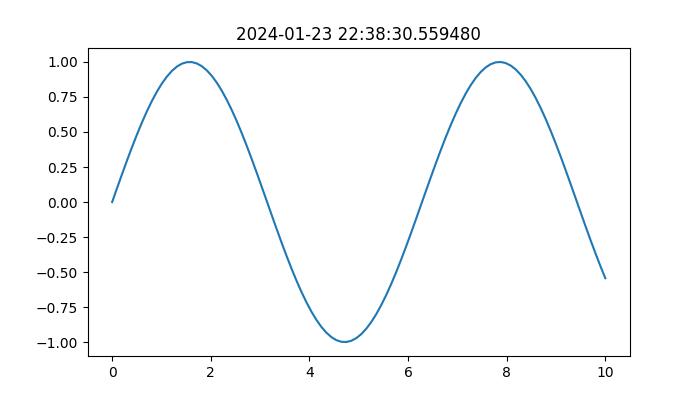
观看下面的视频来观察此示例的工作。

Animated Brownian Walk with Timer
在更高级的场景中,我们使用计时器的优点以动画方式呈现二维布朗运动,从而创建随时间推移产生的具有视觉动态效果的图表。
Example
此示例演示了在创建动画可视化中使用计时器。
import numpy as np
import matplotlib.pyplot as plt
# Callback function for the timer to update line data
def update_line_data(line, x_data, y_data):
x_data.append(x_data[-1] + np.random.normal(0, 1))
y_data.append(y_data[-1] + np.random.normal(0, 1))
line.set_data(x_data, y_data)
line.axes.relim()
line.axes.autoscale_view()
line.axes.figure.canvas.draw()
# Initial data points
x_coords, y_coords = [np.random.normal(0, 1)], [np.random.normal(0, 1)]
# Create a Matplotlib figure and axis
fig, ax = plt.subplots(figsize=(7, 4))
line, = ax.plot(x_coords, y_coords, color='aqua', marker='o')
# Create a new timer with a 100-millisecond interval
animation_timer = fig.canvas.new_timer(interval=1000, callbacks=[(update_line_data, [line, x_coords, y_coords], {})])
animation_timer.start()
# Display the animated plot
plt.show()
在执行上述程序后,你将获得一个带有动画的图形 -

观看下面的视频来观察此示例的工作。
