Selenium 简明教程
Selenium - Capture Videos
Selenium Webdriver 可用于在另一个第三方 API 的帮助下捕获视频。捕获视频有助于调试失败的测试脚本。此外,测试执行过程中的捕获视频可与日志一同分享给开发人员,以便更快地解决问题。
Selenium Webdriver can be used to capture videos with the help of another third party API. Capturing videos help to debug test scripts which fail. Also, the captured video of the test execution can be shared along with the logs to developers for a quicker fix to an issue.
测试用例的捕获视频可以作为证据添加到任何测试管理工具,例如 JIRA、ALM 等。它可以与其他项目利益相关者共享,以便更好地理解和可见性。
A captured video of a test case can be added as evidence to any test management tool like JIRA, ALM, and so on. It can be shared with other project stakeholders for better understanding, and visibility.
Monte Screen Recorder API
Selenium Webdriver 默认情况下无法捕获视频。它必须与称为 Monte Screen Recorder 的另一个 API 集成。为了使用 Monte Screen Recorder 来录制视频,我们需要添加与之相关的 Maven 依赖关系。
Selenium Webdriver is not capable of capturing videos by default. It has to be integrated with another API called the Monte Screen Recorder. In order to use the Monte Screen Recorder for the purpose of recording videos, we would need to add the Maven dependency related to it.
Steps to Capture Videos Using Monte Screen Recorder API
Step 1 - 导航到以下链接 -
Step 1 − Navigate to the below link −
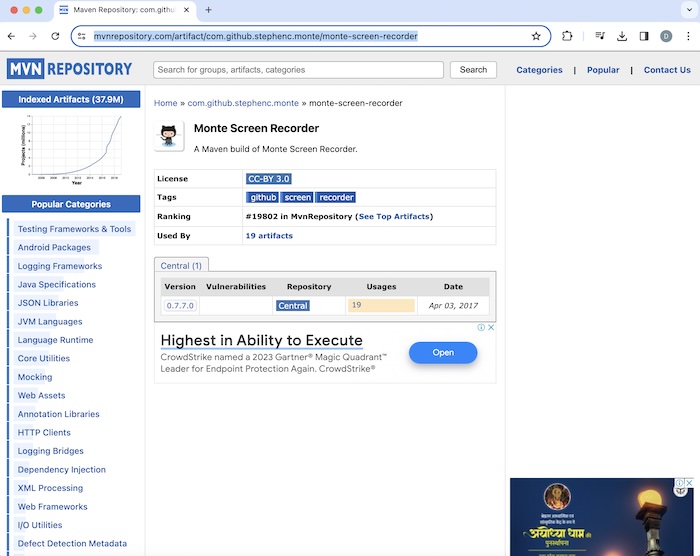
Step 2 − 在版本下面单击 <版本链接>。 在上图中,版本号是 0.7.7.0。
Step 2 − Click on the <version link> below Version. In the above image, the version number is 0.7.7.0.

将 Maven 选项卡下提供的依赖项添加到我们将创建的 Maven 项目的 pom.xml 中。 一旦添加此依赖项,我们就会更新并刷新 Maven 项目。 在上述示例中,以下依赖项如下所示 −
Add the dependency available under the Maven tab within the pom.xml of the Maven project that we would create. Once we would add this dependency, we would update and refresh our maven project. In the above example, the below dependency is given below −
<!-- https://mvnrepository.com/artifact/com.github.stephenc.monte/monte-screen-recorder -->
<dependency>
<groupId>com.github.stephenc.monte</groupId>
<artifactId>monte-screen-recorder</artifactId>
<version>0.7.7.0</version>
</dependency>
Step 3 − 我们将借助以下来源提供的实用程序代码: https://www.randelshofer.ch/monte/ 将 Monte 屏蔽录像机 API 与我们的测试集成。
Step 3 − We would take the help of the utility code available from the source: https://www.randelshofer.ch/monte/ to integrate the Monte Screen Recorder API with our test.
Example: Capturing Videos (Recording a Test)
让我们看下面页面的示例,我们将在这里记录整个测试,从启动应用程序、单击主 Level 1 标签旁的复选框,以及退出浏览器。 然后我们会停止录制。 一旦测试完成,整个录制就会保存在新创建的 test-recordings 文件夹下的测试项目中。 在这个文件夹下,会有一个名为 - main-<with current date and time> 的 .avi 文件,其中有整个测试记录。
Let us take an example of the page below, where we would record the whole test right from launching the application, clicking the checkbox beside the Main Level 1 label, and quitting the browser. After this, we would stop the recording. Once the test would be completed, the whole recording would be saved in the test project under the newly created test-recordings folder. Under this folder, a .avi file with name - main-<with current date and time> having the whole recording of the test, would be available.

实用程序类 ScreenRecorderUtil.java 的代码实现。
Code Implementation for Utility class ScreenRecorderUtil.java.
package org.example;
import java.awt.AWTException;
import java.awt.Dimension;
import java.awt.GraphicsConfiguration;
import java.awt.GraphicsEnvironment;
import java.awt.Rectangle;
import java.awt.Toolkit;
import java.io.File;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Date;
import org.monte.media.Format;
import org.monte.media.FormatKeys.MediaType;
import org.monte.media.Registry;
import org.monte.media.math.Rational;
import org.monte.screenrecorder.ScreenRecorder;
import static org.monte.media.AudioFormatKeys.*;
import static org.monte.media.VideoFormatKeys.*;
public class ScreenRecorderUtil extends ScreenRecorder {
public static ScreenRecorder screenRecorder;
public String name;
public ScreenRecorderUtil(GraphicsConfiguration cfg, Rectangle captureArea, Format fileFormat,
Format screenFormat, Format mouseFormat, Format audioFormat, File movieFolder, String name)
throws IOException, AWTException {
super(cfg, captureArea, fileFormat, screenFormat, mouseFormat, audioFormat, movieFolder);
this.name = name;
}
@Override
protected File createMovieFile(Format fileFormat) throws IOException {
if (!movieFolder.exists()) {
movieFolder.mkdirs();
} else if (!movieFolder.isDirectory()) {
throw new IOException("\"" + movieFolder + "\" is not a directory.");
}
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd HH.mm.ss");
return new File(movieFolder,
name + "-" + dateFormat.format(new Date()) + "." + Registry.getInstance().getExtension(fileFormat));
}
public static void startRecord(String methodName) throws Exception {
File file = new File("./test-recordings/");
Dimension screenSize = Toolkit.getDefaultToolkit().getScreenSize();
int width = screenSize.width;
int height = screenSize.height;
Rectangle captureSize = new Rectangle(0, 0, width, height);
GraphicsConfiguration gc =
GraphicsEnvironment.getLocalGraphicsEnvironment().getDefaultScreenDevice().getDefaultConfiguration();
screenRecorder = new ScreenRecorderUtil(gc, captureSize,
new Format(MediaTypeKey, MediaType.FILE, MimeTypeKey, MIME_AVI),
new Format(MediaTypeKey, MediaType.VIDEO, EncodingKey, ENCODING_AVI_TECHSMITH_SCREEN_CAPTURE,
CompressorNameKey, ENCODING_AVI_TECHSMITH_SCREEN_CAPTURE, DepthKey, 24, FrameRateKey,
Rational.valueOf(15), QualityKey, 1.0f, KeyFrameIntervalKey, 15 * 60),
new Format(MediaTypeKey, MediaType.VIDEO, EncodingKey, "black", FrameRateKey, Rational.valueOf(30)),
null, file, methodName);
screenRecorder.start();
}
public static void stopRecord() throws Exception {
screenRecorder.stop();
}
}
ScreenRecorderUtil.java 的来源: https://www.randelshofer.ch/monte/
Source for ScreenRecorderUtil.java : https://www.randelshofer.ch/monte/
测试类 - CaptureVid.java 的代码实现。
Code Implementation for test class - CaptureVid.java.
package org.example;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import java.util.concurrent.TimeUnit;
public class CaptureVid {
public static void main(String[] args) throws Exception {
// start screen recording
ScreenRecorderUtil.startRecord("main");
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 15 secs
driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
// Opening the webpage where we will identify checkbox
driver.get("https://www.tutorialspoint.com/selenium/practice/check-box.php");
// Identify element then click
WebElement c = driver.findElement(By.xpath("//*[@id='c_bs_1']"));
c.click();
// verify if checkbox is selected
Boolean result = c.isSelected();
System.out.println("Checkbox is selected: " + result);
//Closing browser
driver.quit();
// stop recording
ScreenRecorderUtil.stopRecord();
}
}
pom.xml 中的依赖项。
Dependencies in pom.xml.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>SeleniumJava</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>16</maven.compiler.source>
<maven.compiler.target>16</maven.compiler.target>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java -->
<dependencies>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.11.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.github.stephenc.monte/monte-screen-recorder -->
<dependency>
<groupId>com.github.stephenc.monte</groupId>
<artifactId>monte-screen-recorder</artifactId>
<version>0.7.7.0</version>
</dependency>
</dependencies>
</project>
请注意,在我们的示例中,实用程序文件 ScreenRecorderUtil.java 和测试类文件 CaptureVid.java 是在同一个包 example 下创建的。
Please note that in our example, the utility file ScreenRecorderUtil.java and test class file CaptureVid.java were created under the same package - example.
pom.xml 中的依赖项。
Dependencies in pom.xml.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>SeleniumJava</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>16</maven.compiler.source>
<maven.compiler.target>16</maven.compiler.target>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java -->
<dependencies>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.11.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.github.stephenc.monte/monte-screen-recorder -->
<dependency>
<groupId>com.github.stephenc.monte</groupId>
<artifactId>monte-screen-recorder</artifactId>
<version>0.7.7.0</version>
</dependency>
</dependencies>
</project>
Output
Checkbox is selected: true
Process finished with exit code 0
在上述示例中,我们首先启动了一个应用程序,然后单击复选框,并使用控制台中的消息验证是否选中了复选框 - Checkbox is selected: true 。
In the above example, we had first launched an application and clicked the checkbox and validated if the checkbox is selected with the message in the console - Checkbox is selected: true.
最后,收到了消息 Process finished with exit code 0 ,表示代码成功执行。
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
此外,文件名为 main-2024-02-26 16.29.avi 、位于文件夹 test-recordings 下的视频记录在项目目录中创建。 一旦我们在测试执行后刷新项目文件夹,就能看到这个文件夹。 单击它,我们可以获得示例中执行的所有测试步骤的视频。
Also, the video recording with the filename main-2024-02-26 16.29.avi under the folder test-recordings got created in the project directory. This folder would be visible once we would refresh the project folder after test execution. On clicking it, we would get the video of all the test steps performed in our example.
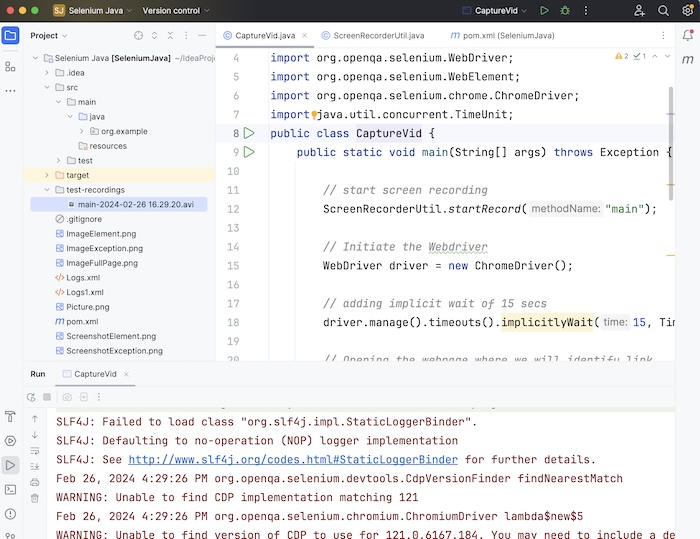
因此,在本教程中,我们讨论了如何使用 Selenium Webdriver 捕捉视频。
Thus, in this tutorial, we had discussed how to capture videos using the Selenium Webdriver.