Selenium 简明教程
Selenium WebDriver - CSV Data File
Selenium Webdriver 可用于与 .csv 数据文件进行交互。通常,在自动化测试中,大量数据通过 .csv 文件进行传递,以正常支持数据驱动框架。.csv 文件看起来类似于一个 Excel 文件,文件扩展名为 .csv。
Selenium Webdriver can be used to interact with the csv data file. Often in automation tests, a large amount of data is passed through a csv file to normally support data driven framework. A csv file looks similar to an excel file and it has an extension of .csv.
Java 提供了几个类和方法来使用 OpenCSV 库对 .csv 文件执行读取和写入数据操作。OpenCSV 具有两个最重要的类 - CSVReader 和 CSVWriter,用于对 .csv 文件执行读取和写入操作。
Java provides few classes and methods to carry out read and write data operations on a csv file using the OpenCSV libraries. The OpenCSV has two most important classes - CSVReader, and CSVWriter to perform read, and write operations on a csv file.
How to Install the OpenCSV?
Step 1 − 从链接 OpenCSV 将 OpenCSV 依赖关系添加到 pom.xml 文件。
Step 1 − Add the OpenCSV dependencies to the pom.xml file from the link OpenCSV.
Step 2 − 保存包含所有依赖关系的 pom.xml 并更新 Maven 项目。
Step 2 − Save the pom.xml with all the dependencies and update the maven project.
Read all Values From a CSV
让我们以名为 Details1.csv 文件的 .csv 为例,其中我们将读取整个 .csv 文件,并使用 CSVReader 类及其 readNext() 方法检索所有值。
Let us take an example of the below csv named the Details1.csv file, where we will read the whole csv file and retrieve all its values using the CSVReader class and its method readNext().
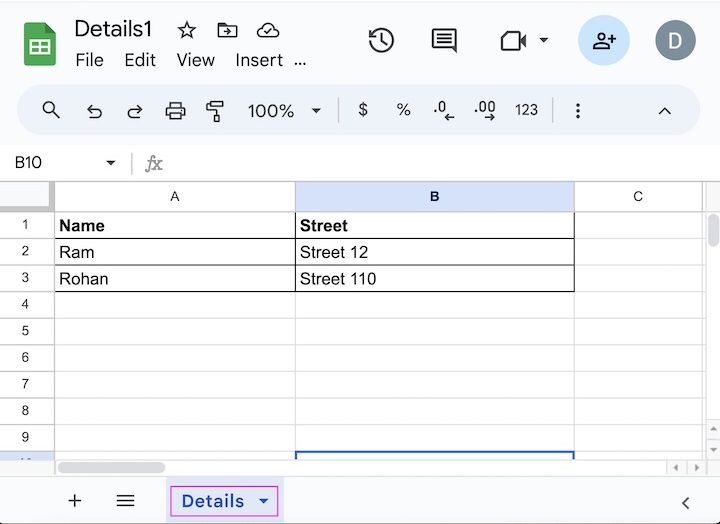
Please Note − 将 Details1.csv 文件放置在项目中的 Resources 文件夹内,如下图所示。
Please Note − The Details1.csv file was placed within the project under the Resources folder as shown in the below image.
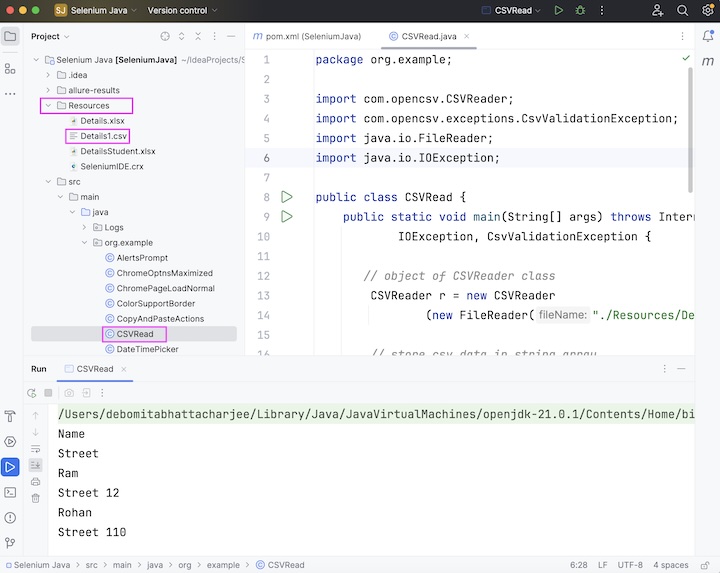
Example
package org.example;
import com.opencsv.CSVReader;
import com.opencsv.exceptions.CsvValidationException;
import java.io.FileReader;
import java.io.IOException;
public class CSVRead {
public static void main(String[] args) throws InterruptedException,
IOException, CsvValidationException {
// object of CSVReader class
CSVReader r = new CSVReader(new FileReader("./Resources/Details1.CSV"));
// store csv data in string array
String [] csvValues;
// iterate through csv till the end of the values
while ((csvValues = r.readNext())!= null){
// iterate through rows
for (String csvValue : csvValues){
System.out.println(csvValue);
}
}
}
}
添加到 pom.xml 的依赖关系。
Dependencies added to pom.xml.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>SeleniumJava</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>16</maven.compiler.source>
<maven.compiler.target>16</maven.compiler.target>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java -->
<dependencies>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.11.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.opencsv/opencsv -->
<dependency>
<groupId>com.opencsv</groupId>
<artifactId>opencsv</artifactId>
<version>5.9</version>
</dependency>
</dependencies>
</project>
Name
Street
Ram
Street 12
Rohan
Street 110
Process finished with exit code 0
在上面的示例中,我们读取了整个 .csv 文件,并在控制台中获取了所有值。
In the above example, we had read the whole csv file and obtained all its value in the console.
最后,收到了消息 Process finished with exit code 0 ,表示代码成功执行。
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Write and Read Values in a CSV
让我们再举一个示例,我们将在项目中的 Resources 文件夹下创建名为 Details2.csv 的 .csv 文件,并使用 CSVWriter 类及其方法 writeNext() 或 writeAll(),以及 flush() 来写入一些值。最后,使用 CSVReader 类及其 readNext() 方法读取这些值。
Let us take another example where we will create a csv file named Details2.csv under the Resources folder in the project and write some values using the CSVWriter class and its methods: writeNext() or writeAll(), and flush(). Finally, read those values using the CSVReader class and its method readNext().
Example
package org.example;
import com.opencsv.CSVReader;
import com.opencsv.CSVWriter;
import com.opencsv.exceptions.CsvValidationException;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
public class CSVReadWrite {
public static void main(String[] args) throws InterruptedException,
IOException, CsvValidationException {
// object of CSVWriter class
CSVWriter w = new CSVWriter(new FileWriter("./Resources/Details2.CSV"));
// stores values in csv
String [] rows1 = {"Name", "Street"};
String [] rows2 = {"Ram", "Street 12"};
String [] rows3 = {"Rohan", "Street 110"};
// add values to be written to list
List<String[]> write = new ArrayList<>();
write.add(rows1);
write.add(rows2);
write.add(rows3);
// write and flush all values
w.writeAll(write);
w.flush();
CSVReader r = new CSVReader(new FileReader("./Resources/Details2.CSV"));
// store csv data in string array
String [] csvValues;
// iterate through csv till the end of the values
while ((csvValues = r.readNext())!= null){
// iterate through rows
for (String csvValue : csvValues){
System.out.println(csvValue);
}
}
}
}
添加到 pom.xml 的依赖关系。
Dependencies added to pom.xml.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>SeleniumJava</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>16</maven.compiler.source>
<maven.compiler.target>16</maven.compiler.target>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java -->
<dependencies>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.11.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.opencsv/opencsv -->
<dependency>
<groupId>com.opencsv</groupId>
<artifactId>opencsv</artifactId>
<version>5.9</version>
</dependency>
</dependencies>
</project>
Name
Street
Ram
Street 12
Rohan
Street 110
Process finished with exit code 0
在上面的示例中,我们在项目中的 Resources 文件夹下创建了一个名为 Details2.csv 的 .csv 文件,并在其中写入了一些值。然后,我们读取了所有这些值,并最终在控制台中获取了这些值。
In the above example, we created a csv file Details2.csv under the Resources folder inside the project and wrote some values in it. Then we read all those values, and finally obtained them in the console.
最后,收到了消息 Process finished with exit code 0 ,表示代码成功执行。
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
此外,会在项目目录中创建名为 Details2.csv 的 .csv 文件。单击此文件后,我们会获取通过上述代码写入的值。
Also, the csv with the filename Details2.csv got created in the project directory. On clicking it, we would get the values written through the above code.
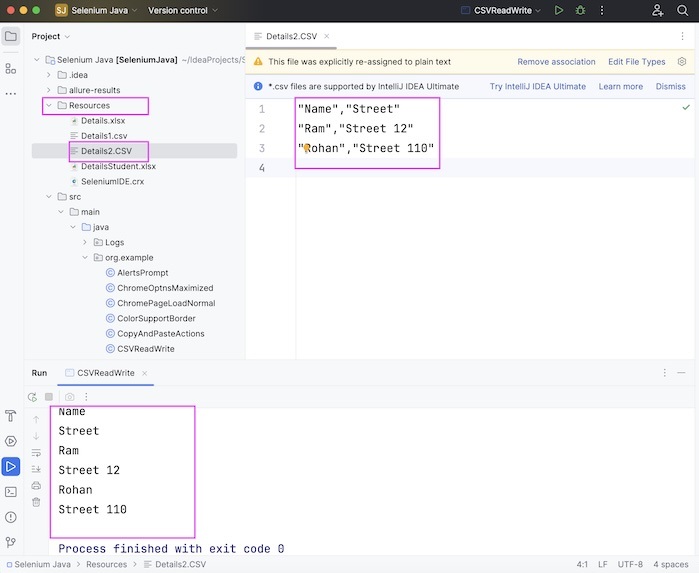
Conclusion
Selenium Webdriver .csv 数据文件教程的全面讲解到此结束。我们从描述 OpenCSV 库是什么、如何安装 OpenCSV,以及介绍如何借助 OpenCSV 和 Selenium Webdriver 读取和写入 .csv 中的值的示例开始。这样便为你在 Selenium Webdriver 中的 .csv 数据文件配备了深入的知识。明智的做法是不断实践你所学的内容,并探索与 Selenium 相关的其他内容,以加深你的理解并拓宽你的视野。
This concludes our comprehensive take on the tutorial on Selenium Webdriver CSV Data File. We’ve started with describing what is an OpenCSV library, how to install OpenCSV, and walked through examples of how to read and write values in csv taking help of OpenCSV along with Selenium Webdriver. This equips you with in-depth knowledge of the CSV Data File in Selenium Webdriver. It is wise to keep practicing what you’ve learned and exploring others relevant to Selenium to deepen your understanding and expand your horizons.