Selenium 简明教程
Selenium WebDriver - Wait Support
Selenium Webdriver 可与 implicit 、 fluent waits 和 @{s11} 等各种等待配合使用,以实现同步并提供等待支持。等待主要应用在测试中,以处理在 Selenium 测试需要其出现在页面或 Dom 中时 Web 元素不可用的情况。
整个页面加载之前通常会存在一些延迟时间,并且 Web 元素在网页上完全可用。Selenium Webdriver 中的等待有助于阻止测试执行,直到元素以其正确状态出现在/消失在网页上。
Basic Waits Available in Selenium Webdriver
Selenium Webdriver 中提供了多种等待。它们如下所示:
Fluent Wait
这是驱动程序等待元素达到特定条件的最高时间。它还决定了驱动程序在定位元素或抛出异常之前进行验证(轮询间隔)的时间间隔。Fluent wait 是自定义显式等待,它提供了一个选项,可以在发生异常时使用自定义消息自动处理特定异常。FluentWait 类用于向测试中添加 Fluent 等待。
Wait wt = new FluentWait(driver)
.withTimeout(20, TimeUnit.SECONDS)
.pollingEvery(5, TimeUnit.SECONDS)
.ignoring(ElementNotInteractableException.class);
wt.until(ExpectedConditions.titleIs("Tutorialspoint"));
在上例中,指定了超时时间和轮询间隔,这意味着驱动程序将等待 20 秒并在超时时间内以 5 秒的间隔进行轮询,直到满足 Tutorialspoint 浏览器标题条件。如果条件在该时间范围内未得到满足,将抛出异常,否则将执行下一步。
Example 1 - Explicit Wait
我们来看一下下图的示例,我们首先单击 Click Me 按钮。
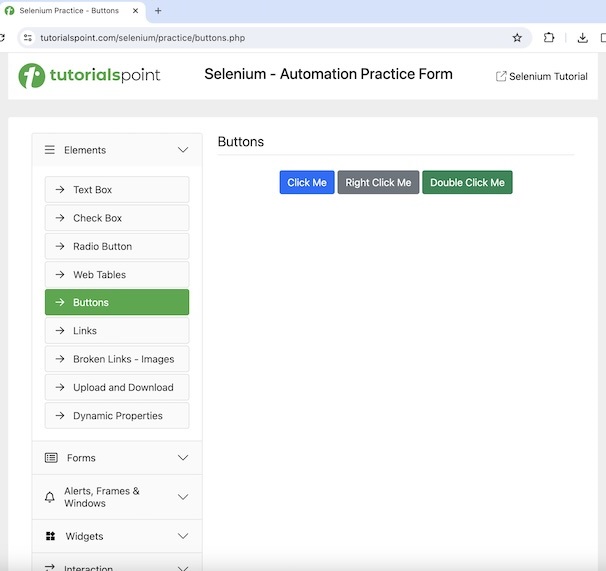
在单击“Click Me”后,我们将使用显式等待并等待文本 You have done a dynamic click 出现在网页上。
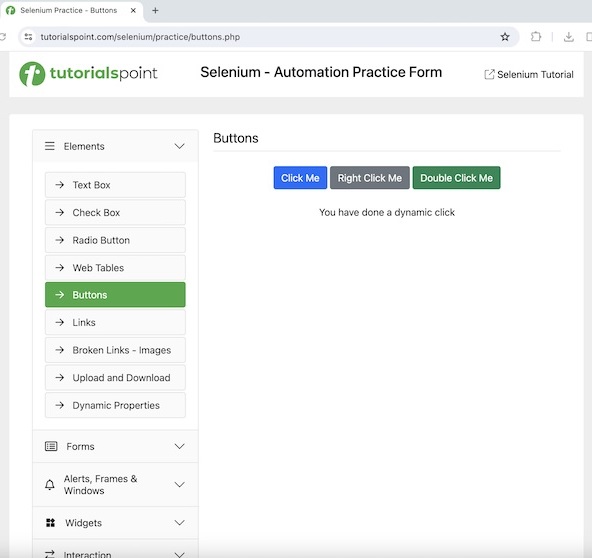
代码实现
package org.example;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import java.time.Duration;
import java.util.concurrent.TimeUnit;
public class ExplicitsWait {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 15 secs
driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
// launching a browser and open a URL
driver.get("https://www.tutorialspoint.com/selenium/practice/buttons.php");
// identify button then click on it
WebElement l = driver.findElement(By.xpath("/html/body/main/div/div/div[2]/button[1]"));
l.click();
// Identify text
WebElement e = driver.findElement(By.xpath("//*[@id='welcomeDiv']"));
// explicit wait to expected condition for presence of a text
WebDriverWait wt = new WebDriverWait(driver, Duration.ofSeconds(2));
wt.until(ExpectedConditions.presenceOfElementLocated(By.xpath("//*[@id='welcomeDiv']")));
// get text
System.out.println("Get text after clicking: " + e.getText());
// Quitting browser
driver.quit();
}
}
Example 2 - Fluent Wait
我们再来看一下以下页面的示例,我们首先单击 Color Change 按钮。

在单击 Color Change 后,我们将使用 Fluent 等待并等待按钮 Visible After 5 Seconds 出现在网页上。
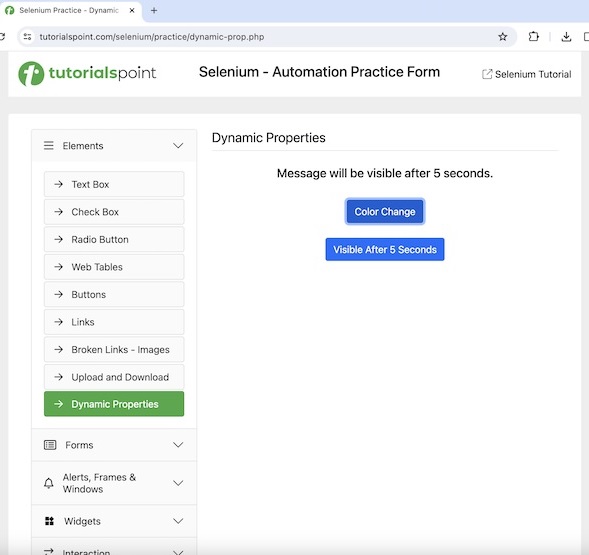
代码实现
package org.example;
import org.openqa.selenium.*;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.FluentWait;
import org.openqa.selenium.support.ui.Wait;
import java.time.Duration;
public class Fluentwts {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// launching a browser and open a URL
driver.get("https://www.tutorialspoint.com/selenium/practice/dynamic-prop.php");
// identify button then click
WebElement l = driver.findElement(By.xpath("//*[@id='colorChange']"));
l.click();
// fluent wait of 6 secs till other button appears
Wait<WebDriver> w = new FluentWait<WebDriver>(driver)
.withTimeout(Duration.ofSeconds(20))
.pollingEvery(Duration.ofSeconds(6))
.ignoring(NoSuchElementException.class);
WebElement m = w.until(ExpectedConditions.visibilityOfElementLocated
(By.xpath("//*[@id='visibleAfter']")));
// checking button presence
System.out.println("Button appeared: " + m.isDisplayed());
// Quitting browser
driver.quit();
}
}