Selenium 简明教程
Selenium WebDriver - Key Up/Down
可以使用 * Actions Class* 的帮助执行 Key Up 和 Key Down 操作。keyUp() 和 keyDown() 方法用于执行 Key Up 和 Key Down 操作。
Selenium Webdriver can be used to perform Key Up and Key Down operations with help of the Actions Class. The methods keyUp() and keyDown() are used to perform Key Up and Key Down operations.
可以使用 Selenium 中的 Keys 类执行复制和粘贴操作。用于复制和粘贴的键可以分别使用 Ctrl + C 和 Ctrl + V 完成。这些待按下的键作为参数发送给 sendKeys() 方法。
The copy and paste operations can be performed by using the Keys class in Selenium. The keys used to copy and paste can be done using the Ctrl + C and Ctrl + V respectively. These keys to be pressed are sent as parameters to the sendKeys() method.
Identification of Elements on a Web Page
右击网页,然后在 Chrome 浏览器中单击“检查”按钮。要调查该网页上的元素,请单击可见 HTML 代码顶部的左上箭头,如下所示。
Right click on the web page, and click on the Inspect button in the Chrome browser. For investigating an element on that web page, click on the left upward arrow, at the top of the visible HTML code as highlighted below.
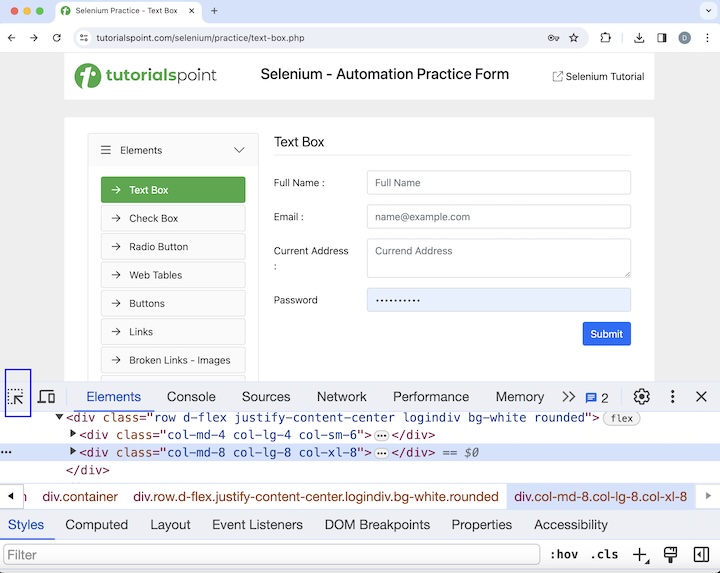
Copy and Paste Text
在下面的页面中,在第一个编辑框中的 Full Name 旁边输入文本 - Copy ,然后将相同的文本复制并粘贴到 Email 旁边的另一个输入框中。
In the below page, enter the text - Copy beside the Full Name in the first edit box, then copy and paste the same text in another input box beside the Email.
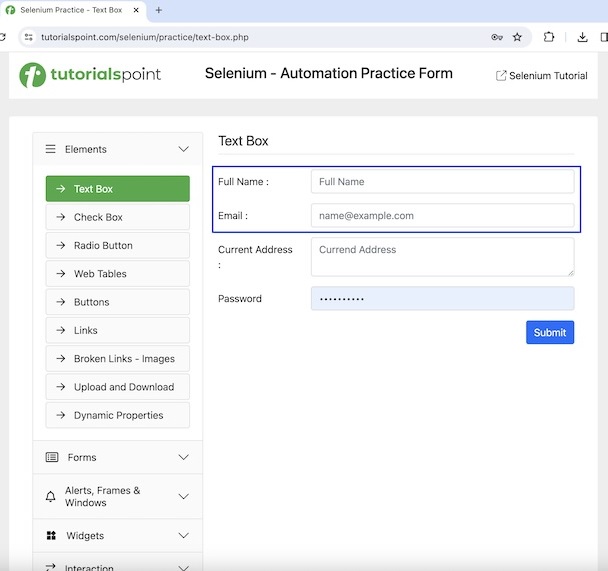
Syntax
MAC 电脑上的语法 −
Syntax on a MAC machine −
WebDriver driver = new ChromeDriver();
// Identify the first input box with xpath locator
WebElement e = driver.findElement(By.xpath("<value of xpath>"));
// enter some text
e.sendKeys("Copy");
// Identify the second input box with xpath locator
WebElement s = driver.findElement(By.xpath("<value of xpath>"));
// Actions class methods to select text
Actions a = new Actions(driver);
a.keyDown(Keys.COMMAND);
a.sendKeys("a");
a.keyUp(Keys.COMMAND);
a.build().perform();
// Actions class methods to copy text
a.keyDown(Keys.COMMAND);
a.sendKeys("c");
a.keyUp(Keys.COMMAND);
a.build().perform();
// Action class methods to tab and reach to the next input box
a.sendKeys(Keys.TAB);
a.build().perform();
// Actions class methods to paste text
a.keyDown(Keys.COMMAND);
a.sendKeys("v");
a.keyUp(Keys.COMMAND);
a.build().perform();
Windows 电脑上的语法 −
Syntax on Windows machine −
WebDriver driver = new ChromeDriver();
// Identify the first input box with xpath locator
WebElement e = driver.findElement(By.xpath("<value of xpath>"));
// enter some text
e.sendKeys("Copy");
// Identify the second input box with xpath locator
WebElement s = driver.findElement(By.xpath("<value of xpath>"));
// Actions class methods to select text
Actions a = new Actions(driver);
a.keyDown(Keys.CONTROL);
a.sendKeys("a");
a.keyUp(Keys.CONTROL);
a.build().perform();
// Actions class methods to copy text
a.keyDown(Keys.CONTROL);
a.sendKeys("c");
a.keyUp(Keys.CONTROL);
a.build().perform();
// Action class methods to tab and reach to next input box
a.sendKeys(Keys.TAB);
a.build().perform();
// Actions class methods to paste text
a.keyDown(Keys.CONTROL);
a.sendKeys("v");
a.keyUp(Keys.CONTROL);
a.build().perform();
Example
package org.example;
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.interactions.Actions;
import java.util.concurrent.TimeUnit;
public class CopyAndPasteAction {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 10 secs
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
// Opening the webpage
driver.get("https://www.tutorialspoint.com/selenium/practice/text-box.php");
// Identify the first input box
WebElement e = driver.findElement(By.xpath("//*[@id='fullname']"));
// enter text
e.sendKeys("Copy");
// select text
Actions act = new Actions(driver);
act.keyDown(Keys.COMMAND);
act.sendKeys("a");
act.keyUp(Keys.COMMAND);
act.build().perform();
// copy text
act.keyDown(Keys.COMMAND);
act.sendKeys("c");
act.keyUp(Keys.COMMAND);
act.build().perform();
// tab to reach to next input box
act.sendKeys(Keys.TAB);
act.build().perform();
// paste text
act.keyDown(Keys.COMMAND);
act.sendKeys("v");
act.keyUp(Keys.COMMAND);
act.build().perform();
// Identify the second input box
WebElement s = driver.findElement(By.xpath("//*[@id='email']"));
// Getting text in the second input box
String text = s.getAttribute("value");
System.out.println("Value copied and pasted: " + text);
// Quit browser
driver.quit();
}
}
Value copied and pasted: Copy
Process finished with exit code 0
在上面的示例中,我们首先在第一个编辑框中输入了文本 Copy ,然后将相同的文本复制并粘贴到第二个编辑框中。最后,我们在第二个输入框中检索输入的文本,作为控制台中的消息 - Value copied and pasted: Copy 。
In the above example, we had first input the text Copy in the first edit box and then copied and pasted the same text in the second edit box. At last, we had retrieved the entered text in the second input box as a message in the console - Value copied and pasted: Copy.
最后,收到了消息 Process finished with exit code 0 ,表示代码成功执行。
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Text in Upper Case
我们再举一个例子,我们将在输入框中以大写字母输入文本 TUTORIALSPOINT ,使用 Actions 类的 Key Up、Key Down 特性。在将值发送到 sendKeys() 方法时,我们将传递 tutorialspoint ,同时按下 SHIFT 键,以将输入的输出作为 TUTORIALSPOINT 输入输入框中。
Let us take another example, where we would enter text TUTORIALSPOINT in an input box in capital letters using the Key Up, Key Down features of Actions class. While sending the value to the sendKeys() method, we would pass tutorialspoint along with pressing SHIFT keys to get the output entered in the input box as TUTORIALSPOINT.
Example
package org.example;
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.interactions.Actions;
import java.util.concurrent.TimeUnit;
public class KeyAction {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 17 secs
driver.manage().timeouts().implicitlyWait(17, TimeUnit.SECONDS);
// Opening the webpage where we will identify an element
driver.get("https://www.tutorialspoint.com/selenium/practice/text-box.php");
// Identify the first input box
WebElement e = driver.findElement(By.xpath("//*[@id='fullname']"));
// Actions class
Actions act = new Actions(driver);
// moving to element and clicking on it
act.moveToElement(e).click();
// key UP and DOWN action for SHIFT
act.keyDown(Keys.SHIFT);
act.sendKeys("Selenium").keyUp(Keys.SHIFT).build().perform();
// get value entered
System.out.println("Text entered: " + e.getAttribute("value"));
// Close browser
driver.quit();
}
}
Text entered: TUTORIALSPOINT
我们用控制台中的消息 Text entered: TUTORIALSPOINT 生成了大写形式的输入文本。
We had obtained the entered text in upper case with the message in the console - Text entered: TUTORIALSPOINT.
Conclusion
以下是我们在 Selenium Webdriver Key Up/Down 教程中全面总结的。我们从描述网页上元素的标识开始,并逐步介绍了如何使用 Selenium Webdriver 处理键上移和键下移方法的示例。这使你掌握了 Selenium Webdriver Key Up/Down 的深入知识。明智的做法是不断练习你学到的内容,并探索其他人与 Selenium 相关的知识,以加深你的理解并扩展你的视野。
This concludes our comprehensive take on the tutorial on Selenium Webdriver Key Up/Down. We’ve started with describing identification of elements on a web page, and walked through examples on how to handle key up and key down methods with Selenium Webdriver. This equips you with in-depth knowledge of the Selenium Webdriver Key Up/Down. It is wise to keep practicing what you’ve learned and exploring others relevant to Selenium to deepen your understanding and expand your horizons.