Selenium 简明教程
Selenium Webdriver - JSON Data File
Selenium Webdriver 可用于与 json 数据文件交互。通常在自动化测试中,需要通过 json 文件向测试用例提供大量数据以验证特定场景或创建数据驱动框架。json 文件包含键值对形式的数据,其扩展名为 .json。
Java 提供了很少的类和方法,使用 JSON Simple 库执行 json 文件的读写数据操作。JSON Simple 是一个用于操作 JSON 对象的轻量级库。
How to Install the JSON Simple?
Step 1 − 从以下链接添加 JSON Simple 依赖项 −
Step 2 − 保存包含所有依赖关系的 pom.xml 并更新 Maven 项目。
Example 1 - Read all Values From a Json File
我们以命名为 Detail.json 文件的下方 json 为例,我们将读取整个 json 文件,并使用 JSONParser 类及其方法 parse() 检索其所有值。
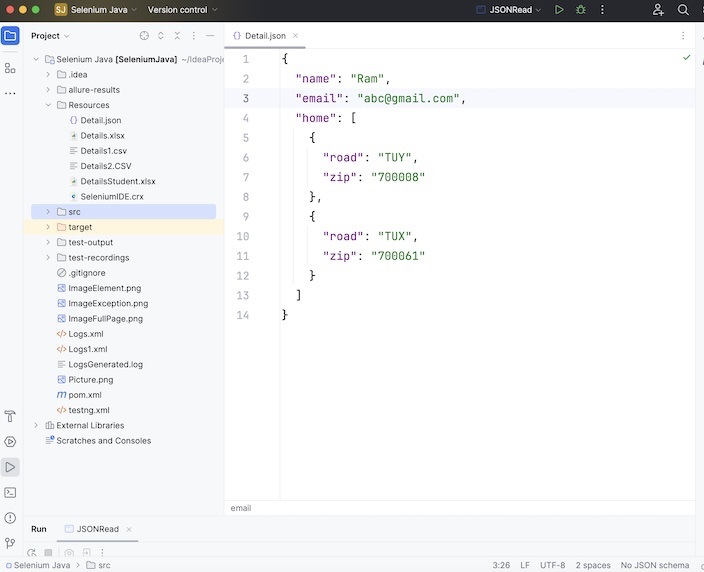
Detail.json 文件的内容为 −
{
"name": "Ram",
"email": "abc@gmail.com",
"home": [
{
"road": "TUY",
"zip": "700008"
},
{
"road": "TUX",
"zip": "700061"
}
]
}
从 Detail.json 文件检索值后,我们将输入 Full Name 和 Email 字段中 name 和 email 键的值。
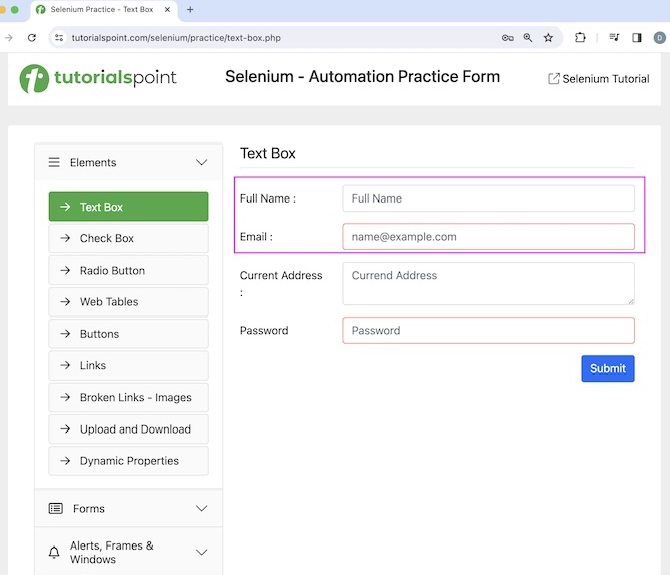
请注意: Details.json 文件位于 Resources 文件夹下,如下图所示。
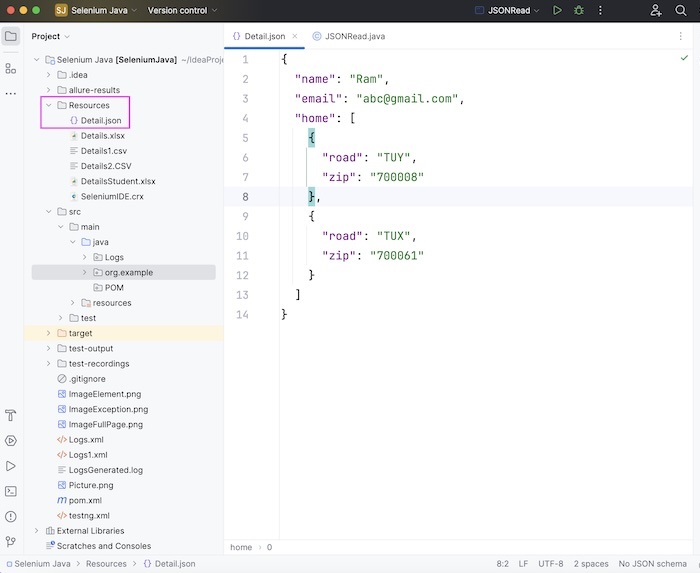
JSONRead.java 类文件上的代码实现。
package org.example;
import org.json.simple.JSONArray;
import org.json.simple.JSONObject;
import org.json.simple.parser.JSONParser;
import org.json.simple.parser.ParseException;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import java.io.FileReader;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
public class JSONRead {
public static void main(String[] args) throws IOException, ParseException {
//object of JSONParser
JSONParser j = new JSONParser();
// load json file to be read
FileReader f = new FileReader("./Resources/Detail.json");
// parse json content
Object o = j.parse(f);
// convert parsing object to JSON object
JSONObject detail = (JSONObject)o;
// get values from JSON file
String name = (String)detail.get("name");
String email = (String)detail.get("email");
System.out.println("First Name: " + name);
System.out.println("Email: " + email);
// get values from JSON array
JSONArray h = (JSONArray)detail.get("home");
// iterate through the JSONArray
for(int i = 0; i < h.size(); i ++){
JSONObject home = (JSONObject) h.get(i);
String road = (String)home.get("road");
String zip = (String)home.get("zip");
System.out.println("Road: " + road);
System.out.println("Zip: " + zip);
}
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 12 secs
driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS);
// Opening the webpage
driver.get("https://www.tutorialspoint.com/selenium/practice/text-box.php");
//Identify elements
WebElement n = driver.findElement(By.xpath("//*[@id='fullname']"));
WebElement m = driver.findElement(By.xpath("//*[@id='email']"));
// enter name and email after reading from JSON file
n.sendKeys(name);
m.sendKeys(email);
// get values entered
String enteredName = n.getAttribute("value");
String enteredEmail = m.getAttribute("value");
System.out.println("Entered Name: " + enteredName);
System.out.println("Entered Email: " + enteredEmail);
// quitting browser
driver.quit();
}
}
添加到 pom.xml 的依赖关系。
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>SeleniumJava</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>16</maven.compiler.source>
<maven.compiler.target>16</maven.compiler.target>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java -->
<dependencies>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.11.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.googlecode.json-simple/json-simple -->
<dependency>
<groupId>com.googlecode.json-simple</groupId>
<artifactId>json-simple</artifactId>
<version>1.1.1</version>
</dependency>
</dependencies>
</project>
Output
First Name: Ram
Email: abc@gmail.com
Road: TUY
Zip: 700008
Road: TUX
Zip: 700061
Entered Name: Ram
Entered Email: abc@gmail.com
Process finished with exit code 0
在上述示例中,我们已经读取了整个 json 文件,并在控制台中以消息 First Name: Ram, Email: abc@gmail.com, Road: TUY, Zip: 700008, Road: TUX 和 Zip: 700061 获取了其所有值。然后,将通过读取 JSON 文件获得的姓氏和电子邮件的值输入到网页上的输入框,同时还使用控制台中的消息检索输入的值 - Entered Name: Ram 和 Entered Email: abc@gmail.com 。
最后,收到了消息 Process finished with exit code 0 ,表示代码成功执行。
Example 2 - Write and Read Values in a Json File
我们再举一个例子,在项目中的 Resources 文件夹下创建一个名为 Detail1.json 的 json 文件,并在其中写入一些值。
package org.example;
import org.json.simple.JSONObject;
import org.json.simple.parser.JSONParser;
import org.json.simple.parser.ParseException;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
public class JSONReadWrite {
public static void main(String[] args) throws IOException, ParseException {
// object of JSONObject
JSONObject j = new JSONObject();
// generate key-value pairs in JSON file
j.put("Name", "Selenium");
j.put("Language", "Java");
// create JSON file with no duplicate values
FileWriter f = new FileWriter("./Resources/Detail1.json", false);
// write on json file
f.write(j.toJSONString());
// close file after write
f.close();
//object of JSONParser
JSONParser j1 = new JSONParser();
// load json file to be read
FileReader f1 = new FileReader("./Resources/Detail1.json");
// parse json content
Object o = j1.parse(f1);
// convert parsing object to JSON object
JSONObject detail = (JSONObject)o;
// get values from JSON file
String name = (String)detail.get("Name");
String language = (String)detail.get("Language");
System.out.println("Name: " + name);
System.out.println("Language: " + language);
}
}
添加到 pom.xml 的依赖关系。
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>SeleniumJava</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>16</maven.compiler.source>
<maven.compiler.target>16</maven.compiler.target>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java -->
<dependencies>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.11.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.googlecode.json-simple/json-simple -->
<dependency>
<groupId>com.googlecode.json-simple</groupId>
<artifactId>json-simple</artifactId>
<version>1.1.1</version>
</dependency>
</dependencies>
</project>
Output
Name: Selenium
Language: Java
Process finished with exit code 0
在上述示例中,我们在项目中的 Resources 文件夹下创建了一个名为 Detail1.json 的 json 文件并在其中写入了一些值。然后,我们读取所有这些值,并最终在控制台中以消息 – Name: Selenium 和 Language: Java 获得这些值。
最后,收到了消息 Process finished with exit code 0 ,表示代码成功执行。
此外,名为 Detail1.csv 的 json 在项目目录中创建。单击它,我们将获取通过上述代码编写的值。
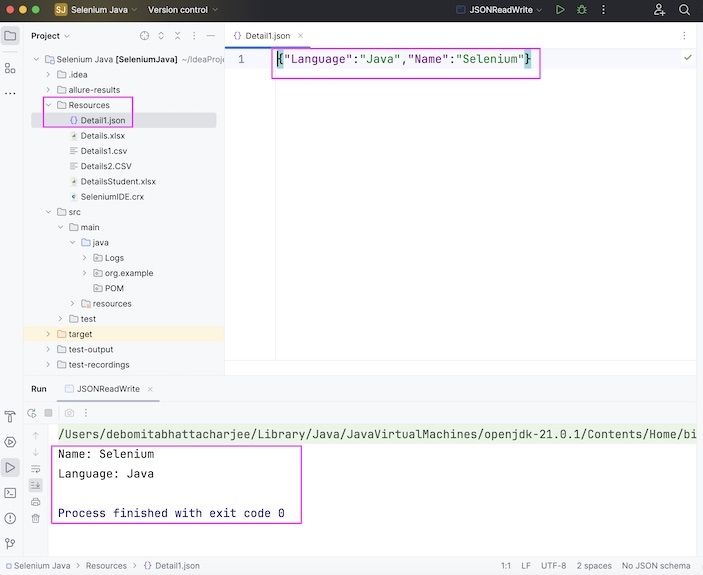
至此,我们结束了 Selenium Webdriver - JSON 数据文件教程的全面介绍。我们首先介绍了什么是 JSON Simple 库,如何安装 JSON Simple,并通过示例演示了如何使用 JSON Simple 读取和写入 json 中的值以及 Selenium Webdriver。这使你掌握了 Selenium Webdriver 中 JSON 数据文件的深入知识。明智的做法是继续练习你所学的内容,并探索与 Selenium 相关的其他内容,以加深你的理解并拓宽你的视野。