Selenium 简明教程
Selenium WebDriver - Date Time Picker
Selenium Webdriver 可用于从日历中选择日期和时间。日历中的日期时间选取器可以在 Web UI 中以多种方式设计。根据 UI,需要设计测试用例。
Selenium Webdriver can be used to select date and time from a calendar. A Date Time Picker in a calendar can be designed in numerous ways on the web UI. Based on the UI, the test case needs to be designed.
What is a Date Time Picker?
日期时间选取器基本上是在待测应用程序中创建日历的用户界面。日期时间选取器的功能是从某个范围内选择特定日期和/或时间。
The Date Time Picker is basically a user interface to create a calendar in the application under test. The functionality of a Date time picker is to select a particular date and/or time from a range.
How to Inspect a Date Time Picker?
要识别下图所示的日历,首先需要点击选择日期字段下的第一个输入框,然后右键单击网页,最后单击 Chrome 浏览器中的检查按钮。要调查页面上的日历,请单击 HTML 代码顶部的左上角箭头,如下所示高亮显示。
To identify a calendar shown in the below image first we would click on the first input box under the Select Date field, and then right click on the web page, and finally click on the Inspect button in the Chrome browser. For investigating on a calendar on a page, click on the left upward arrow, available at the top of the HTML code as highlighted below.

Past Date Selection
我们以页面中日期时间选取器的示例为例,该示例具有选择年份、月份、日期、小时和分钟等选项。它还包含选择追溯或未来日期和时间。让我们选择追溯的日期和时间 - 早上 2023-06-04 09:05,然后验证它们。
Let us take the example of the Date Time Picker in the page below having the option to select year, month, day, hour, minutes, and so on. It also contains the option to select backdated or future date and time as well. Let’s select a back dated date and time - 2023-06-04 09:05 in the morning, and then verify them.

Example
package org.example;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.Select;
import java.util.concurrent.TimeUnit;
public class DatTimePickers {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 20 secs
driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS);
// URL launch for accessing calendar
driver.get("https://www.tutorialspoint.com/selenium/practice/date-picker.php");
// identify element to get calendar
WebElement f = driver
.findElement(By.xpath("//*[@id='datetimepicker2']"));
f.click();
// selecting month June
WebElement month = driver
.findElement(By.xpath("/html/body/div[3]/div[1]/div/div/select"));
Select select = new Select(month);
select.selectByVisibleText("June");
// getting selected month
String selectedMonth = select.getFirstSelectedOption().getText();
// selecting year 2023
WebElement year = driver.findElement(By.xpath("/html/body/div[3]/div[1]/div/div/div/input"));
// removing existing year then entering
year.clear();
year.sendKeys("2023");
// selecting day 4
WebElement day = driver.findElement(By.xpath("//span[contains(@aria-label,'"+selectedMonth+" 4')]"));
day.click();
// selecting AM time
WebElement time = driver.findElement(By.xpath("/html/body/div[3]/div[3]/span[2]"));
if (time.getText().equalsIgnoreCase("PM")){
time.click();
}
// selecting hour
WebElement hour = driver
.findElement(By.xpath("/html/body/div[3]/div[3]/div[1]/input"));
// removing existing hour then entering
hour.clear();
hour.sendKeys("9");
// selecting minutes
WebElement minutes = driver.findElement(By.xpath("/html/body/div[3]/div[3]/div[2]/input"));
// removing existing minutes then entering
minutes.clear();
minutes.sendKeys("5");
// reflecting both date and time
f.click();
// get date and time selected
String v = f.getAttribute("value");
System.out.println("Date and time selected by Date Time Picker: " + v);
// check date and time selected
if (v.equalsIgnoreCase("2023-06-04 09:05")){
System.out.print("Date and Time selected successfully");
} else {
System.out.print("Date and Time selected unsuccessfully");
}
// close browser
driver.quit();
}
}
Date and time selected by Date Time Picker: 2023-06-04 09:05
Date and Time selected successfully
Process finished with exit code 0
在上述示例中,我们已使用日期时间选取器从日历中选择了追溯的日期和时间,然后使用消息 Date and time selected by Date Time Picker: 2023-06-04 09:05 和 Date and Time selected successfully 验证是否在控制台中选择了正确日期。
In the above example, we had selected the back dated date and time from the calendar using a Date Time Picker, and then verified if the proper date is selected in the console with the messages - Date and time selected by Date Time Picker: 2023-06-04 09:05 and Date and Time selected successfully.
最后,收到了消息 Process finished with exit code 0 ,表示代码成功执行。
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Future Date Selection
让我们再以页面中日期时间选取器的另一个示例为例,我们将选择未来日期和时间,傍晚的 2025-06-04 21:05,然后验证它们。
Let us take another example of the Date Time Picker in the below page we would select a future dated date and time - 2025-06-04 21:05 in the evening, and then verify them.
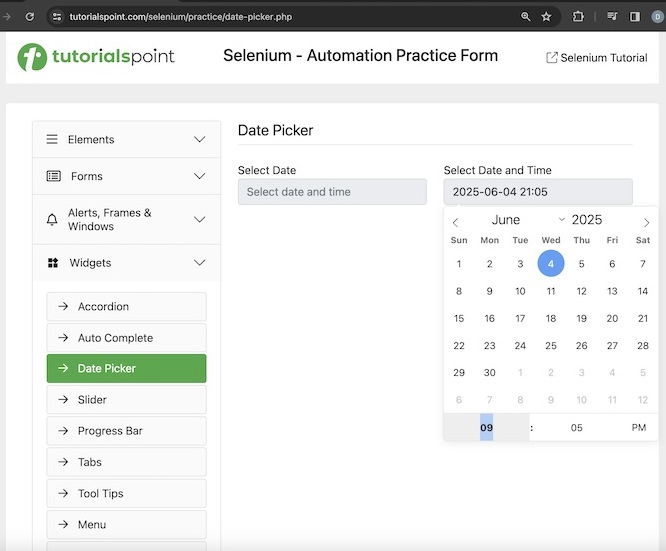
Example
package org.example;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.Select;
import java.util.concurrent.TimeUnit;
public class DatTimePickersForward {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 20 secs
driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS);
// URL launch for accessing calendar
driver.get("https://www.tutorialspoint.com/selenium/practice/date-picker.php");
// identify element to get calendar
WebElement f = driver.findElement(By.xpath("//*[@id='datetimepicker2']"));
f.click();
// selecting month June
WebElement month = driver.findElement(By.xpath("/html/body/div[3]/div[1]/div/div/select"));
Select select = new Select(month);
select.selectByVisibleText("June");
// getting selected month
String selectedMonth = select.getFirstSelectedOption().getText();
// selecting year 2023
WebElement year = driver.findElement(By.xpath("/html/body/div[3]/div[1]/div/div/div/input"));
// removing existing year then entering
year.clear();
year.sendKeys("2025");
// selecting day 4
WebElement day = driver.findElement(By.xpath
("//span[contains(@aria-label,'"+selectedMonth+" 4')]"));
day.click();
// selecting PM time
WebElement time = driver.findElement(By.xpath("/html/body/div[3]/div[3]/span[2]"));
if (time.getText().equalsIgnoreCase("AM")){
time.click();
}
// selecting hour
WebElement hour = driver.findElement(By.xpath("/html/body/div[3]/div[3]/div[1]/input"));
// removing existing hour then entering
hour.clear();
hour.sendKeys("9");
// selecting minutes
WebElement minutes = driver.findElement(By.xpath("/html/body/div[3]/div[3]/div[2]/input"));
// removing existing minutes then entering
minutes.clear();
minutes.sendKeys("5");
// reflecting both date and time
f.click();
// get date and time selected
String v = f.getAttribute("value");
System.out.println("Date and time selected by Date Time Picker: " + v);
// check date and time selected
if (v.equalsIgnoreCase("2025-06-04 21:05")){
System.out.print("Date and Time selected successfully");
} else {
System.out.print("Date and Time selected unsuccessfully");
}
// close browser
driver.quit();
}
}
Date and time selected by Date Time Picker: 2025-06-04 21:05
Date and Time selected successfully
Process finished with exit code 0
在上述示例中,我们已使用日期时间选取器从日历中选择了未来日期和时间,然后使用消息 Date and time selected by Date Time Picker: 2025-06-04 21:05 和 Date and Time selected successfully 验证是否在控制台中选择了正确日期。
In the above example, we had selected the future dated date and time from the calendar using a Date Time Picker, and then verified if the proper date is selected in the console with the messages - Date and time selected by Date Time Picker: 2025-06-04 21:05 and Date and Time selected successfully.
最后,收到了消息 Process finished with exit code 0 ,表示代码成功执行。
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Current Date Selection
让我们举一个日期时间选择器的另一个示例,在下面的页面中,我们将选择一个当前的日期和时间,然后验证它们。请注意,在这个日期时间选择器中,当前日期默认被选中,我们只需要选择当前时间。
Let us take another example of the Date Time Picker in the below page we would select a current dated date and time and then verify them. Please note, in this Date Time Picker, the current date is selected by default, we would need to only select the current time.

Example
package org.example;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
import java.util.concurrent.TimeUnit;
public class DateTimePickersCurrent {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 20 secs
driver.manage().timeouts().implicitlyWait(20, TimeUnit.SECONDS);
// URL launch for accessing calendar
driver.get("https://www.tutorialspoint.com/selenium/practice/date-picker.php");
// get current time
LocalDateTime l = LocalDateTime.now();
DateTimeFormatter formatter =
DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm");
String dateTime = l.format(formatter);
String[] dtTime = dateTime.split(" ");
String time = dtTime[1];
String[] t = time.split(":");
String hour = t[0];
String minute = t[1];
// identify element to get calendar
WebElement f = driver.findElement(By.xpath("//*[@id='datetimepicker2']"));
f.click();
// selecting hour based on current
WebElement hours = driver.findElement(By.xpath("/html/body/div[3]/div[3]/div[1]/input"));
// removing existing hour then entering
hours.clear();
hours.sendKeys(hour);
// selecting minutes based on current minutes
WebElement minutes = driver.findElement(By.xpath("/html/body/div[3]/div[3]/div[2]/input"));
// removing existing minutes then entering
minutes.clear();
minutes.sendKeys(minute);
// reflecting both date and time
f.click();
// get date and time selected
String v = f.getAttribute("value");
System.out.println("Date and time selected by Date Time Picker: " + v);
// check date and time selected
if (v.equalsIgnoreCase(dateTime)){
System.out.print("Date and Time selected successfully");
} else {
System.out.print("Date and Time selected unsuccessfully");
}
// close browser
driver.quit();
}
}
Date and time selected by Date Time Picker: 2024-03-13 13:29
Date and Time selected successfully
Process finished with exit code 0
在上面的示例中,我们已经使用日期时间选择器从日历中选择了当前日期和时间,然后使用消息 Date and time selected by Date Time Picker: 2024-03-13 13:29 和 Date and Time selected successfully 验证控制台中是否选择了适当的日期。
In the above example, we had selected the current date and time from the calendar using a Date Time Picker, and then verified if the proper date is selected in the console with the messages - Date and time selected by Date Time Picker: 2024-03-13 13:29 and Date and Time selected successfully.
最后,收到了消息 Process finished with exit code 0 ,表示代码成功执行。
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Conclusion
这就结束了我们对 Selenium Webdriver 日期时间选择器教程的全面讲解。我们从描述什么是日期时间选择器、如何检查它以及通过 Selenium 选择过去、当前和现在的日期和时间的示例开始。这让你全面了解 Selenium 中的日期时间选择器。明智的做法是继续练习你学到的东西并探索其他与 Selenium 相关的内容以加深你的理解并拓宽你的视野。
This concludes our comprehensive take on the tutorial on Selenium Webdriver Date Time Picker. We’ve started with describing what is a Date Time Picker, how to inspect it and examples to walk through how to select past, current, and present date and time with Selenium. This equips you with in-depth knowledge of the Date Time Picker in Selenium. It is wise to keep practicing what you’ve learned and exploring others relevant to Selenium to deepen your understanding and expand your horizons.