Selenium 简明教程
Selenium WebDriver - First Test Script
在我们开始使用 Selenium 的第一个测试脚本之前,我们应确保在系统中安装了 Java 和 Maven。
Before starting with our first test script on Selenium, we should ensure that Java, and Maven have been installed in our system.
Prerequisites Steps To Create the First Test Script
Step 1 − 通过从命令提示符运行命令 java 确认 Java 安装。
Step 1 − Confirm Java installation by running the command: java, from the command prompt.
Step 2 − 通过运行命令确认已安装的 Java 版本:
Step 2 − Confirm the version of the Java installed by running the command:
java –version
Step 3 − 通过运行命令确认已安装的 Maven 版本 −
Step 3 − Confirm the version of the Maven installed by running the command −
mvn –version
Step 4 − 下载并安装任何代码编辑器,比如从链接 Download Eclipse Technology 下载 Eclipse。
Step 4 − Download and install any code editor, say the Eclipse from the link Download Eclipse Technology.
若要获取有关 Eclipse 及其安装过程的更多信息,请访问链接 Eclipse Tutorial 。
To get more information about the Eclipse and its installation process, please visit the link Eclipse Tutorial.
Steps To Create the First Test Script
Step 1 − 初始化一个驱动程序会话并在浏览器上执行一些活动,比如说在 Chrome 浏览器上使用如下代码启动一个网页 −
Step 1 − Initiate a driver session and perform some activity on the browser, say launching an web page on the Chrome browser with the help of the below code −
Webdriver driver = new ChromeDriver();
driver.get("https://www.tutorialspoint.com/selenium/practice/text-box.php");
Step 2 − 使用 getCurrentUrl() 方法检索在 Chrome 浏览器上启动的当前 URL 的一些信息。
Step 2 − Retrieve some information about the current URL that is launched on the Chrome browser with the help of the getCurrentUrl() method.
driver.getCurrentUrl();
Step 3 − 添加一些同步技术,以便 Selenium 工具与浏览器操作之间的交互保持同步。Selenium 中有几种同步方法,如隐式、显式和流畅等待。
Step 3 − Add some synchronization techniques so that the interactions between the Selenium tool and browser actions would be in sync. There are several synchronization methods available in Selenium, like the implicit, explicit, and fluent waits.
虽然隐式等待是一类全局等待(适用于文本案例中的所有步骤),但显式和流畅等待应用于浏览器上的特定元素。对这里我们的第一个测试脚本,我们只应用隐式等待。
While implicit wait is a kind of global wait (applicable to all the steps in a text case), explicit and fluent waits are applied to a specific element on the browser. For our first test script here, we would only apply an implicit wait.
添加 15 秒隐式等待的语法 −
Syntax to add an implicit wait of 15 seconds −
driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
若要获取有关 Selenium 中不同等待的更多信息,请访问链接 Selenium Webdriver Wait Support 。
To get more information about the different waits in Selenium, please visit the link Selenium Webdriver Wait Support.
Step 4 − 对同一 webdriver 会话中的 webelement 执行任何任务,为此,通过任何 Selenium Locators (如 id、name、class、tagname、链接文本、部分链接文本、xpath 和 css 选择器)以及 findElement() 方法唯一地标识元素。如果有多个具有相同定位器值元素,则只应标识第一个匹配元素。
Step 4 − Perform any task on a webelement in the same webdriver session, for that purpose, identify the element uniquely with the help of any of the Selenium Locators like id, name, class, tagname, link text, partial link text, xpath, and css selector along with findElement() method. If there are multiple elements with the same value of locator, only the first matching element should be identified.
用 xpath 定位器标识元素的语法 −
Syntax to identify an element with xpath locator −
WebElement e = driver.findElement(By.xpath("<value of xpath>"));
Step 5 − 使用 sendKeys() 方法对前一步中标识的元素执行任务。输入文本 - Selenium,要输入的文本作为参数传递给该方法。
Step 5 − Perform a task on the element identified on the previous step using the sendKeys() method. Enter the text - Selenium and text to be entered is passed as an argument to the method.
Syntax
e.sendKeys("Selenium");
Step 6 − 使用 getAttribute() 方法请求有关输入框输入值的的信息,并将值作为参数传递给该方法。
Step 6 − Request the information on the value entered on input box using the getAttribute() method and value is passed as an argument to the method.
Syntax
String text = driver.findElement(By.xpath("<value of xpath>")).getAttribute("value");
Step 7 − 使用 clear() 方法清除输入的文本。
Step 7 − Wipe out the text entered with the clear() method.
Syntax
e.clear();
Step 8 − 使用 getAttribute() 方法再次请求有关输入框中的值的信息(以证明在使用 clear() 方法后输入框中没有值),并将值作为参数传递给该方法。
Step 8 − Again request the information on the value on the input box using the getAttribute() method(to prove there is no value within the input box after using the clear() method) and value is passed as an argument to the method.
Syntax
String text = driver.findElement(By.xpath("<value of xpath>")).getAttribute("value");
Step 9 − 使用 quit() 方法终止驱动程序会话,该方法还将关闭已打开的浏览器窗口。
Step 9 − Terminate the driver session using the quit() method which would also close the opened browser window.
Example
右键单击以下网页,然后在 Chrome 浏览器中单击“检查”按钮。若要标识页面上的第一个文本框,单击可见 HTML 代码顶部的左上箭头,如下所示突出显示。
Right click on the web page below, and then click on the Inspect button in the Chrome browser. For identifying the first text box on the page, click on the left upward arrow at the top of the visible HTML code as highlighted below.

一旦单击并将箭头指向输入框(在以下图片中突出显示),其 HTML 代码就会出现,反映具有文本值 type 属性的输入标记名。
Once we had clicked and pointed the arrow to the input box(highlighted in the below image), its HTML code appeared, reflecting the input tagname and the type attribute having the value as text.
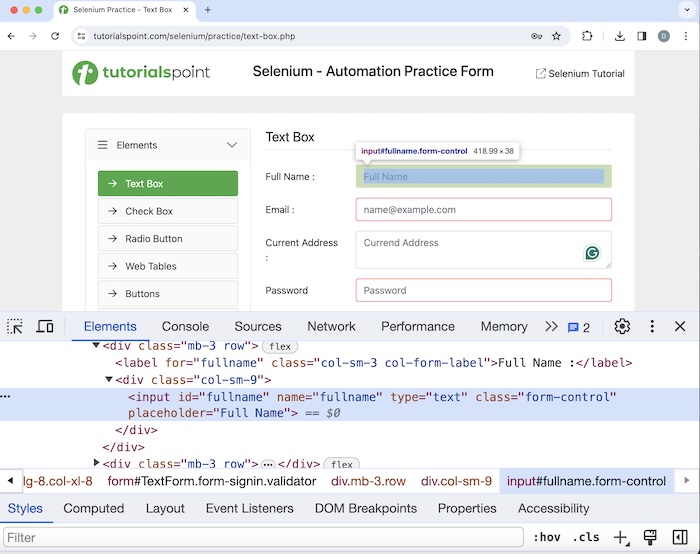
我们以上述页面的示例为例,在这个示例中,我们首先使用 sendKeys() 方法在文本框中输入文本。之后,我们使用 clear() 方法清除文本。
Let us take an example of the above page, where we would first input text in the text box with the help of the sendKeys() method. Later, we would clear out the text with the help of the clear() method.
代码实现
Code Implementation
package org.example;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import java.util.concurrent.TimeUnit;
public class FrstJavaTestScript {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 15 secs
driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
// Opening the webpage where we will identify edit box to enter driver.get("https://www.tutorialspoint.com/selenium/practice/text-box.php");
// getting current URL
System.out.println("Getting the Current URL: " + driver.getCurrentUrl());
// Identify the input box with xpath locator
WebElement e = driver.findElement(By.xpath("//*[@id='fullname']"));
// enter text in input box
e.sendKeys("First Test Script");
// Get the value entered
String text = e.getAttribute("value");
System.out.println("Entered text is: " + text);
// clear the text entered
e.clear();
// Get no text after clearing text
String text1 = e.getAttribute("value");
System.out.println("Get text after clearing: " + text1);
// Closing browser
driver.quit();
}
}
Getting the Current URL:
https://www.tutorialspoint.com/selenium/practice/text-box.php
Entered text is: First Test Script
Get text after clearing:
Process finished with exit code 0
在上述示例中,我们首先使用以下控制台消息获取了当前 URL: Getting the Current URL: Selenium Automation Practice Form 。然后我们在输入框中输入了文本 First Test Script ,我们使用消息 - Entered text is: First Test Script 检索了控制台中输入的值。接下来,我们清除了输入的值,因此在同一文本框中没有获取到值。
In the above example, we had first obtained the current URL with the message in the console: Getting the Current URL: Selenium Automation Practice Form . Then we had entered the text First Test Script in the input box, We had retrieved the value entered in the console with the message - Entered text is: First Test Script. Next, we had cleared the value entered, and hence, obtained no value in the same text box.
最后,收到了消息 Process finished with exit code 0 ,表示代码成功执行。
Finally, the message Process finished with exit code 0 was received, signifying successful execution of the code.
Conclusion
这总结了我们对 Selenium Webdriver 第一个测试脚本教程的全面介绍。我们从描述创建第一个测试脚本的先决条件步骤、创建第一个测试脚本的步骤以及举例说明如何在 Selenium Webdriver 中创建第一个测试脚本开始。这让你深入了解 Selenium Webdriver - 第一个测试脚本。明智的做法是不断练习你所学的内容,并探索其他与 Selenium 相关的主题,以加深你的理解并拓宽你的视野。
This concludes our comprehensive take on the tutorial on Selenium Webdriver First Test Script. We’ve started with describing prerequisite steps to create the First Test Script, steps to create the First Test Script, and an example to illustrate how to create the first test script in Selenium Webdriver. This equips you with in-depth knowledge of the Selenium Webdriver - First Test Script. It is wise to keep practicing what you’ve learned and exploring others relevant to Selenium to deepen your understanding and expand your horizons.