Selenium 简明教程
Selenium WebDriver - Alerts & Popups
Selenium Webdriver 可用于处理警报和弹出窗口。网页上的警报旨在显示警告消息或信息,或获取用户的授权以进行进一步的操作。
Basic Methods to Handle Alerts & Popups in Selenium
Selenium 中提供了多种方法,可用于基于警报和弹出窗口使测试实现自动化。要访问警报,我们必须首先使用 switchTo().alert() 方法将驱动程序上下文切换到警报。让我们详细讨论 Alert 接口的一些方法 −
-
driver.switchTo().alert().accept() − 用于通过单击警报上显示的“确定”按钮来接受警报。
-
driver.switchTo().alert().dismiss() − 用于通过单击警报上显示的“取消”按钮来关闭警报。
-
driver.switchTo().alert().getText() − 用于获取显示在警报上的文本。
-
driver.switchTo().alert().sendKeys("value to be entered") − 用于输入显示在警报上输入文本的文本。
请注意,在使用 Alert 接口时,我们需要在测试中添加导入语句 import org.openqa.selenium.Alert 。
Example 1 - Alerts
让我们讨论一下网页上常见的警报。单击页面上的 Alert 按钮时,将会生成警报,其文本为 - Hello world! ,如下所示。

代码实现
package org.example;
import org.openqa.selenium.Alert;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import java.util.concurrent.TimeUnit;
public class Alrts {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 12 secs
driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS);
// Opening the webpage where we will get alert
driver.get("https://www.tutorialspoint.com/selenium/practice/alerts.php");
// identify button for clicking to get alert
WebElement c = driver.findElement(By.xpath("//button[text()='Alert']"));
c.click();
// switch driver context to alert
Alert alrt = driver.switchTo().alert();
// dismiss alert
alrt.dismiss();
//again get the alert
c.click();
// Get alert text
String s = alrt.getText();
System.out.println("Alert text is: " + s);
// accept alert
alrt.accept();
// quitting the browser
driver.quit();
}
}
Alert text is: Hello world!
Process finished with exit code 0
在上面的示例中,我们捕获了警报上的文本并在控制台中收到了消息 - Alert text is: Hello world! 。
最后,收到了消息 Process finished with exit code 0 ,表示代码成功执行。
Example 2 - Confirmation Alerts
让我们讨论一下网页上的另一个警报,如下所示。单击第二个 Click Me 按钮时,我们会在网页上收到文本为 - Press a button! 的警报。

单击警报上的“确定”按钮后,我们将在网页上收到文本 You pressed OK! 。
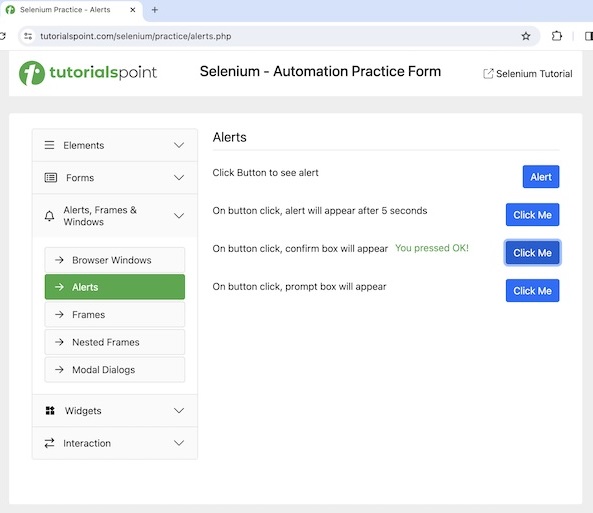
单击警报上的“取消”按钮后,我们将在页面上收到文本“您按下了取消!”。

代码实现
package org.example;
import org.openqa.selenium.Alert;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import java.util.concurrent.TimeUnit;
public class AlertJS {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 12 secs
driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS);
// Opening the webpage where we will get alert
driver.get("https://www.tutorialspoint.com/selenium/practice/alerts.php");
// identify button for clicking to get alert
WebElement c =driver.findElement
(By.xpath("/html/body/main/div/div/div[2]/div[3]/button"));
c.click();
// switch driver context to alert
Alert alrt = driver.switchTo().alert();
// Get alert text
String s = alrt.getText();
System.out.println("Alert text is: " + s);
//accept alert
alrt.accept();
// get text after accepting alert
WebElement text =driver.findElement(By.xpath("//*[@id='desk']"));
System.out.println("Text appearing after alert accept: " + text.getText());
// again get the alert
c.click();
// switch driver context to alert
Alert alrt1 = driver.switchTo().alert();
// now dismiss alert
alrt1.dismiss();
// get text after dismissing alert
WebElement text1 =driver.findElement(By.xpath("//*[@id='desk']"));
System.out.println("Text appearing after alert dismiss: " + text1.getText());
// quitting the browser
driver.quit();
}
}
Alert text is: Press a button!
Text appearing after alert accept: You pressed OK!
Text appearing after alert dismiss: You pressed Cancel!
在上面的示例中,我们捕获了警报上的文本并在控制台中收到了消息 - Alert text is: Press a button! 。然后在接受警报后,我们捕获了显示在页面上的文本,其中控制台上显示的消息为 - Text appearing after alert accept: You pressed OK! 。另外,在关闭警报时,我们捕获了显示在页面上的文本,其中控制台上显示的消息为 - Text appearing after alert dismiss: You pressed Cancel! 。
Example 3 - Prompt Alerts
让我们在网页上讨论另一个弹出框,如下所示。点击第三个 Click Me 按钮,我们会在网页上得到一个带有 What is your name? 文本和输入框的弹出框。

代码实现
package org.example;
import org.openqa.selenium.Alert;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import java.util.concurrent.TimeUnit;
public class AlertsPromp {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 12 secs
driver.manage().timeouts().implicitlyWait(12, TimeUnit.SECONDS);
// Opening the webpage where we will get alert
driver.get("https://www.tutorialspoint.com/selenium/practice/alerts.php");
// identify button for clicking to get alert
WebElement c =driver.findElement
(By.xpath("/html/body/main/div/div/div[2]/div[4]/button"));
c.click();
// switch driver context to alert
Alert alrt = driver.switchTo().alert();
// Get alert text
String s = alrt.getText();
System.out.println("Alert text is: " + s);
// enter text then accept alert
alrt.sendKeys("Selenium");
alrt.accept();
// again get the alert
c.click();
// switch driver context to alert
Alert alrt1 = driver.switchTo().alert();
// again enter text then dismiss alert
alrt1.sendKeys("Selenium");
alrt1.dismiss();
// quitting the browser
driver.quit();
}
}
Alert text is: What is your name?
在上述示例中,我们在弹出框中捕获了文本,并收到控制台中的信息 - Alert text is: What is your name?
Example 4 - Alert with Wait Condition
我们在网页上讨论另一个弹出框,如下所示。点击第一个 Click Me 按钮,我们会在 5 秒后得到一个带有 Hello just appeared 文本的弹出框。
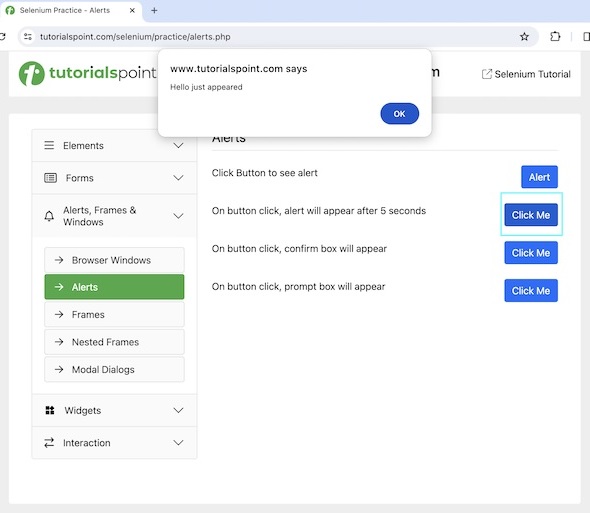
代码实现
package org.example;
import org.openqa.selenium.Alert;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import java.time.Duration;
public class AlertsPromp {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// Opening the webpage where we will get alert
driver.get("https://www.tutorialspoint.com/selenium/practice/alerts.php");
// identify button for clicking to get alert
WebElement c = driver.findElement
(By.xpath("/html/body/main/div/div/div[2]/div[2]/button"));
c.click();
// explicit wait to expected condition for presence alert
WebDriverWait wt = new WebDriverWait(driver, Duration.ofSeconds(6));
wt.until(ExpectedConditions.alertIsPresent());
// switch driver context to alert
Alert alrt = driver.switchTo().alert();
// Get alert text
String s = alrt.getText();
System.out.println("Text is: " + s);
// accept alert
alrt.accept();
// quitting the browser
driver.quit();
}
}
Text is: Hello just appeared