Selenium 简明教程
Selenium WebDriver - File Upload
Selenium Webdriver 可用于自动化需要在网页上上传文件的测试。在 HTML 术语中,文件上传元素由称为 {s1} 的标签名称标识。此外,它应具有值为 {s3} 的属性 {s2}。
Identify Upload Functionality in HTML
右键单击下方所示页面,然后单击 Chrome 浏览器中的“检查”按钮。结果,整个页面的完整 HTML 代码将可见。要检查页面上的“选择文件”按钮,请单击左上角箭头,如下所示。
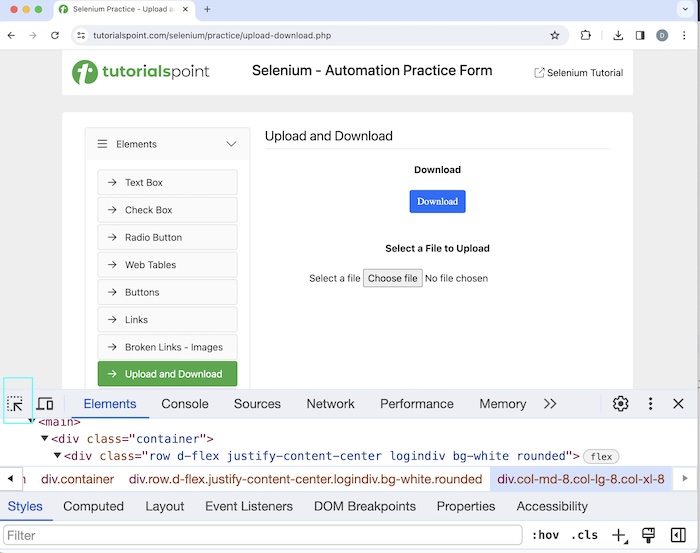
单击并指向文本 {s4} 旁边的该按钮后,其 HTML 代码将可见,其中反映了 {s5} 标签名,以及值为 {s7} 的 {s6} 属性。

<input id="uploadFile" type="file" lang="en" class="form-control-file">
要上传文件,我们将使用 {s8} 方法。要上传的文件的路径作为该方法的参数传递。
Syntax
WebDriver driver = new ChromeDriver();
// identify element the element
WebElement l = driver.findElement(By.xpath("value of xpath locator"));
// getting file path to be uploaded
File f = new File("./Picture.png");
System.out.println("Getting the file path to be uploaded: " + f.getAbsolutePath());
// uploading file with path of file uploaded
m.sendKeys(f.getAbsolutePath());
代码实现
package org.example;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import java.io.File;
import java.util.concurrent.TimeUnit;
public class FilesUpload {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 15 secs
driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
// Opening the webpage where we will upload a file
driver.get("https://www.tutorialspoint.com/selenium/practice/upload-download.php");
// identify element with xpath for file upload
WebElement m = driver.findElement(By.xpath("//*[@id='uploadFile']"));
// getting file path to be uploaded
File f = new File("./Picture.png");
System.out.println("Getting the file path to be uploaded: " + f.getAbsolutePath());
// uploading file with path of file uploaded
m.sendKeys(f.getAbsolutePath());
// check if file uploaded successfully
if (m.getAttribute("value").equalsIgnoreCase("Picture.png")) {
System.out.println("File uploaded successfully ");
} else {
System.out.println("File uploaded unsuccessfully ");
}
// Closing browser
driver.quit();
}
}
在 pom.xml 文件中添加的依赖项:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>SeleniumJava</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>16</maven.compiler.source>
<maven.compiler.target>16</maven.compiler.target>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java -->
<dependencies>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.11.0</version>
</dependency>
</dependencies>
</project>
Exception with Incorrect File Path
让我们再举一个示例,其中将要上传的文件的错误路径作为参数传递给 sendKeys() 方法(即将上传的文件名命名为 {s14},而不是 Picture.png)。在这种情况下,将不会上传文件,并且会引发异常。
Example
package org.example;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import java.io.File;
import java.util.concurrent.TimeUnit;
public class FilesExcUpload {
public static void main(String[] args) throws InterruptedException {
// Initiate the Webdriver
WebDriver driver = new ChromeDriver();
// adding implicit wait of 15 secs
driver.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
// Opening the webpage where we will upload a file
driver.get("https://www.tutorialspoint.com/selenium/practice/upload-download.php");
// identify element with xpath for file upload
WebElement m = driver.findElement(By.xpath("//*[@id='uploadFile']"));
// getting file path to be uploaded
File f = new File("./Picture1.png");
System.out.println("Getting the file path to be uploaded: " + f.getAbsolutePath());
// uploading file with path of file uploaded
m.sendKeys(f.getAbsolutePath());
// check if file uploaded successfully
if (m.getAttribute("value").equalsIgnoreCase("Picture1.png")) {
System.out.println("File uploaded successfully ");
} else {
System.out.println("File uploaded unsuccessfully ");
}
// Closing browser
driver.quit();
}
}
Getting the file path to be uploaded:
/Users/IdeaProjects/Selenium Java/./Picture1.png
Exception in thread "main"
org.openqa.selenium.InvalidArgumentException: invalid argument:
File not found : /Users/IdeaProjects/Selenium Java/./Picture1.png
Process finished with exit code 1
在上述示例中,我们通过控制台中的消息 {s15} 获得了要上传的文件的路径。然后收到异常,因为发送了不正确的文件路径以进行上传。
最后,收到了消息 Process finished with exit code 1 ,表示代码执行失败。