Partitioning with the RabbitMQ Binder
RabbitMQ 本身不支持分区。
有时,向特定分区发送数据非常有利,例如,当你希望对消息处理进行严格的排序时,特定客户的所有消息都应该发送到相同的分区。
RabbitMessageChannelBinder
通过为每个分区绑定一个队列到目标交换区来提供分区。
以下 Java 和 YAML 示例展示如何配置生产者:
Producer
@SpringBootApplication
public class RabbitPartitionProducerApplication {
private static final Random RANDOM = new Random(System.currentTimeMillis());
private static final String[] data = new String[] {
"abc1", "def1", "qux1",
"abc2", "def2", "qux2",
"abc3", "def3", "qux3",
"abc4", "def4", "qux4",
};
public static void main(String[] args) {
new SpringApplicationBuilder(RabbitPartitionProducerApplication.class)
.web(false)
.run(args);
}
@Bean
public Supplier<Message<?>> generate() {
return () -> {
String value = data[RANDOM.nextInt(data.length)];
System.out.println("Sending: " + value);
return MessageBuilder.withPayload(value)
.setHeader("partitionKey", value)
.build();
};
}
}
application.yml
spring:
cloud:
stream:
bindings:
generate-out-0:
destination: partitioned.destination
producer:
partitioned: true
partition-key-expression: headers['partitionKey']
partition-count: 2
required-groups:
- myGroup
前面示例中的配置使用默认分区( |
下面的配置调配了一个主题交换区:

以下队列绑定到该交换区:

以下绑定将队列与交换区关联:
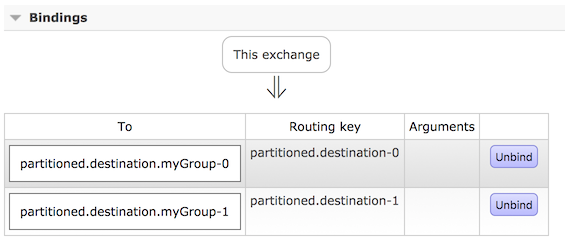
以下 Java 和 YAML 示例延续了前面的示例,并展示了如何配置消费者:
Consumer
@SpringBootApplication
public class RabbitPartitionConsumerApplication {
public static void main(String[] args) {
new SpringApplicationBuilder(RabbitPartitionConsumerApplication.class)
.web(false)
.run(args);
}
@Bean
public Consumer<Message<String>> listen() {
return message -> {
String queue =- message.getHeaders().get(AmqpHeaders.CONSUMER_QUEUE);
System.out.println(in + " received from queue " + queue);
};
}
}
application.yml
spring:
cloud:
stream:
bindings:
listen-in-0:
destination: partitioned.destination
group: myGroup
consumer:
partitioned: true
instance-index: 0
The RabbitMessageChannelBinder
不支持动态扩展。每个分区都必须至少有一个使用者。使用者的 instanceIndex
用于指示消耗了哪个分区。诸如 Cloud Foundry 之类的平台只能使用 instanceIndex
拥有一个实例。