VertexAI Embeddings
Generative Language PaLM API 允许开发人员使用 PaLM 模型构建生成式 AI 应用程序。大语言模型 (LLM) 是一种功能强大且用途广泛的机器学习模型类型,它使计算机能够通过一系列提示理解和生成自然语言。PaLM API 基于 Google 的下一代 LLM,PaLM。它擅长各种不同的任务,如代码生成、推理和写作。你可以使用 PaLM API 为内容生成、对话代理、摘要和分类系统等用例构建生成式 AI 应用程序。
The Generative Language PaLM API allows developers to build generative AI applications using the PaLM model. Large Language Models (LLMs) are a powerful, versatile type of machine learning model that enables computers to comprehend and generate natural language through a series of prompts. The PaLM API is based on Google’s next generation LLM, PaLM. It excels at a variety of different tasks like code generation, reasoning, and writing. You can use the PaLM API to build generative AI applications for use cases like content generation, dialogue agents, summarization and classification systems, and more.
基于 Models REST API。
Based on the Models REST API.
Prerequisites
要访问 PaLM2 REST API,你需要获取 makersuite 的访问 API KEY 表单。
To access the PaLM2 REST API you need to obtain an access API KEY form makersuite.
目前 PaLM API 在美国境外不可用,但您可以使用 VPN 进行测试。 |
Currently the PaLM API it is not available outside US, but you can use VPN for testing. |
Spring AI 项目定义了一个名为 spring.ai.vertex.ai.api-key
的配置属性,你应该将其设置为获得的 API Key
的值。导出环境变量是设置该配置属性的一种方法:
The Spring AI project defines a configuration property named spring.ai.vertex.ai.api-key
that you should set to the value of the API Key
obtained.
Exporting an environment variable is one way to set that configuration property:
export SPRING_AI_VERTEX_AI_API_KEY=<INSERT KEY HERE>
Add Repositories and BOM
Spring AI 工件发布在 Spring Milestone 和 Snapshot 存储库中。有关将这些存储库添加到你的构建系统的说明,请参阅 Repositories 部分。
Spring AI artifacts are published in Spring Milestone and Snapshot repositories. Refer to the Repositories section to add these repositories to your build system.
为了帮助进行依赖项管理,Spring AI 提供了一个 BOM(物料清单)以确保在整个项目中使用一致版本的 Spring AI。有关将 Spring AI BOM 添加到你的构建系统的说明,请参阅 Dependency Management 部分。
To help with dependency management, Spring AI provides a BOM (bill of materials) to ensure that a consistent version of Spring AI is used throughout the entire project. Refer to the Dependency Management section to add the Spring AI BOM to your build system.
Auto-configuration
Spring AI 为 VertexAI Embedding 客户端提供了 Spring Boot 自动配置。要启用它,请将以下依赖项添加到项目的 Maven pom.xml
文件:
Spring AI provides Spring Boot auto-configuration for the VertexAI Embedding Client.
To enable it add the following dependency to your project’s Maven pom.xml
file:
<dependency>
<groupId>org.springframework.ai</groupId>
<artifactId>spring-ai-vertex-ai-palm2-spring-boot-starter</artifactId>
</dependency>
或添加到 Gradle build.gradle
构建文件中。
or to your Gradle build.gradle
build file.
dependencies {
implementation 'org.springframework.ai:spring-ai-vertex-ai-palm2-spring-boot-starter'
}
|
Refer to the Dependency Management section to add the Spring AI BOM to your build file. |
Embedding Properties
前缀 spring.ai.vertex.ai
用作属性前缀,它允许你连接到 VertexAI。
The prefix spring.ai.vertex.ai
is used as the property prefix that lets you connect to VertexAI.
Property | Description | Default |
---|---|---|
spring.ai.vertex.ai.ai.base-url |
The URL to connect to |
[role="bare"]https://generativelanguage.googleapis.com/v1beta3 |
spring.ai.vertex.ai.api-key |
The API Key |
- |
前缀 spring.ai.vertex.ai.embedding
是一个属性前缀,可让您为 VertexAI Chat 配置嵌入客户端实现。
The prefix spring.ai.vertex.ai.embedding
is the property prefix that lets you configure the embedding client implementation for VertexAI Chat.
Property | Description | Default |
---|---|---|
spring.ai.vertex.ai.embedding.enabled |
Enable Vertex AI PaLM API Embedding client. |
true |
spring.ai.vertex.ai.embedding.model |
This is the Vertex Embedding model to use |
embedding-gecko-001 |
Sample Controller (Auto-configuration)
Create 一个新的 Spring Boot 项目,并将 spring-ai-vertex-ai-palm2-spring-boot-starter
添加到你的 pom(或 gradle)依赖项。
Create a new Spring Boot project and add the spring-ai-vertex-ai-palm2-spring-boot-starter
to your pom (or gradle) dependencies.
在 src/main/resources
目录下添加一个 application.properties
文件,以启用并配置 VertexAi Chat 客户端:
Add a application.properties
file, under the src/main/resources
directory, to enable and configure the VertexAi Chat client:
spring.ai.vertex.ai.api-key=YOUR_API_KEY
spring.ai.vertex.ai.embedding.model=embedding-gecko-001
将 |
replace the |
这会创建一个您可以注入到类中的 VertexAiPaLm2EmbeddingClient
实现。以下是一个使用嵌入客户端进行文本生成的简单 @Controller
类的示例。
This will create a VertexAiPaLm2EmbeddingClient
implementation that you can inject into your class.
Here is an example of a simple @Controller
class that uses the embedding client for text generations.
@RestController
public class EmbeddingController {
private final EmbeddingClient embeddingClient;
@Autowired
public EmbeddingController(EmbeddingClient embeddingClient) {
this.embeddingClient = embeddingClient;
}
@GetMapping("/ai/embedding")
public Map embed(@RequestParam(value = "message", defaultValue = "Tell me a joke") String message) {
EmbeddingResponse embeddingResponse = this.embeddingClient.embedForResponse(List.of(message));
return Map.of("embedding", embeddingResponse);
}
}
Manual Configuration
VertexAiPaLm2EmbeddingClient实现`EmbeddingClient`,并且使用Low-level VertexAiPaLm2Api Client连接到VertexAI服务。
The VertexAiPaLm2EmbeddingClient implements the EmbeddingClient
and uses the Low-level VertexAiPaLm2Api Client to connect to the VertexAI service.
将 spring-ai-vertex-ai
依赖项添加到项目的 Maven pom.xml
文件:
Add the spring-ai-vertex-ai
dependency to your project’s Maven pom.xml
file:
<dependency>
<groupId>org.springframework.ai</groupId>
<artifactId>spring-ai-vertex-ai-palm2</artifactId>
</dependency>
或添加到 Gradle build.gradle
构建文件中。
or to your Gradle build.gradle
build file.
dependencies {
implementation 'org.springframework.ai:spring-ai-vertex-ai-palm2'
}
|
Refer to the Dependency Management section to add the Spring AI BOM to your build file. |
接下来,创建一个 VertexAiPaLm2EmbeddingClient
并将它用于文本生成:
Next, create a VertexAiPaLm2EmbeddingClient
and use it for text generations:
VertexAiPaLm2Api vertexAiApi = new VertexAiPaLm2Api(< YOUR PALM_API_KEY>);
var embeddingClient = new VertexAiPaLm2EmbeddingClient(vertexAiApi);
EmbeddingResponse embeddingResponse = embeddingClient
.embedForResponse(List.of("Hello World", "World is big and salvation is near"));
Low-level VertexAiPaLm2Api Client
VertexAiPaLm2Api 为 VertexAiPaLm2Api Chat API 提供轻量级 Java 客户端。
The VertexAiPaLm2Api provides is lightweight Java client for VertexAiPaLm2Api Chat API.
下方的类图阐释了 VertexAiPaLm2Api
嵌入接口和构建模块:
Following class diagram illustrates the VertexAiPaLm2Api
embedding interfaces and building blocks:
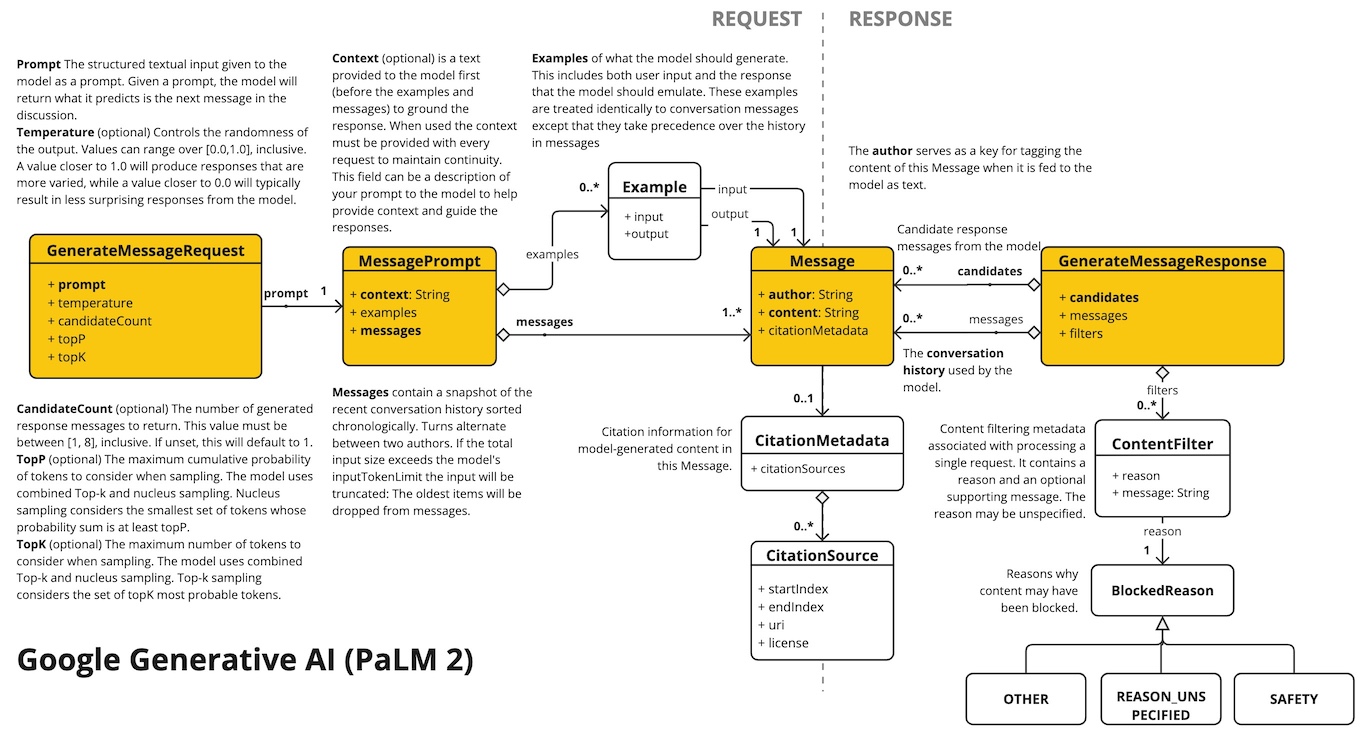
下面是一个简单的片段,说明如何以编程方式使用 API:
Here is a simple snippet how to use the api programmatically:
VertexAiPaLm2Api vertexAiApi = new VertexAiPaLm2Api(< YOUR PALM_API_KEY>);
// Generate
var prompt = new MessagePrompt(List.of(new Message("0", "Hello, how are you?")));
GenerateMessageRequest request = new GenerateMessageRequest(prompt);
GenerateMessageResponse response = vertexAiApi.generateMessage(request);
// Embed text
Embedding embedding = vertexAiApi.embedText("Hello, how are you?");
// Batch embedding
List<Embedding> embeddings = vertexAiApi.batchEmbedText(List.of("Hello, how are you?", "I am fine, thank you!"));