Python Pillow 简明教程
Python Pillow - Batch Processing Images
使用 Python Pillow 批量处理图像可以让你有效地将相同的编辑或操作同时应用到多张图像上。这种方法对于诸如调整大小、裁剪、重命名、添加水印或格式化图像等任务非常有价值,因为它增强了工作流效率并优化了输出。
Batch processing images in Python Pillow allows you to efficiently apply the same edits or operations to multiple images simultaneously. This approach is valuable for tasks like resizing, cropping, renaming, watermarking, or formatting images, as it enhances workflow efficiency and optimizes output.
当使用大量图像时,这一点特别有用,因为它可以显著提高计算机的速度和效率。它让你对每张图像应用相同的操作,从而节省时间和精力。
This is particularly useful when working with a large number of images, as it can significantly impact the speed and efficiency of your computer. It allows you to apply the same operation to each image, saving time and effort.
Steps for Batch Processing Images
使用 Python Pillow 批量处理图像涉及以下步骤:
The steps involved in batch processing images with Python Pillow are as follows −
-
*Create a List of Image Files: * Generate a list of file paths to the images you want to process.
-
*Iterate Through the List of Images: *Use loops to traverse the list of image files. You can use 'for' or 'while' loops to handle each image one at a time.
-
*Perform Image Processing Operations: *Within the loop, apply the desired image processing operations to each image. This may include resizing, cropping, applying filters, adding text or watermarks, or any other operation necessary.
-
*Save Processed Images: *After performing the processing operations on each image, save the processed image to the desired location.
Resizing Images using Batch Processing
使用 Python Pillow 批量处理调整图像大小是图像处理中的一项常见任务。它涉及将多张图像的尺寸调整到特定大小或宽高比。
Resizing images using Python Pillow batch processing is one of the common tasks in image processing. It involves adjusting the dimensions of multiple images to a specific size or aspect ratio.
Example
这里有一个例子,演示了如何使用 Python Pillow 批量处理一次调整多张图像的大小。在这个例子中,一个指定文件夹中的一系列 JPG 图像被调整到特定大小(例如,700x400)。
Here is an example that demonstrates the resizing multiple images at once using the Python Pillow batch processing. In this example, a collection of JPG images in a specified folder is resized to a specific size (e.g., 700x400).
from PIL import Image
import glob
import os
# Get a list of image files in the 'Images for Batch Operation' directory
input_directory = "Images for Batch Operation"
output_directory = "Output directory for Batch Operation"
image_files = [f for f in glob.glob(os.path.join(input_directory, "*.jpg"))]
for file in image_files:
image = Image.open(file)
# Resize the image to a specific size (e.g., 700x400)
image = image.resize((700, 400))
# Save the resized image to the 'Output directory for Batch Operation' directory
output_path = os.path.join(output_directory, os.path.basename(file))
image.save(output_path)
以下图像显示了输入目录中可用的图像文件列表:
Following image represents the list of image files available in the input directory −
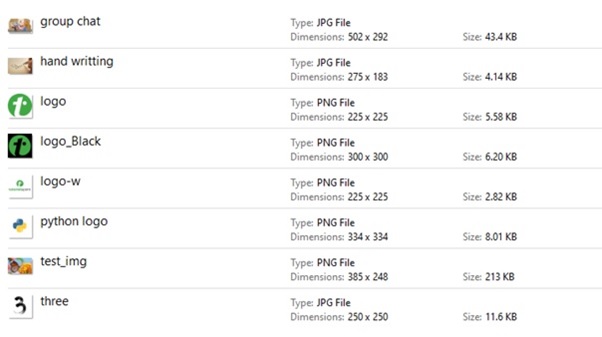
如果我们导航到输出图像保存的目录(即,“批量操作的输出目录”),我们将能够看到如下所示的调整大小的图像:
If we navigate to the directory where the output image was saved (i.e, "Output directory for Batch Operation"), we will be able to observe resized images as shown below −
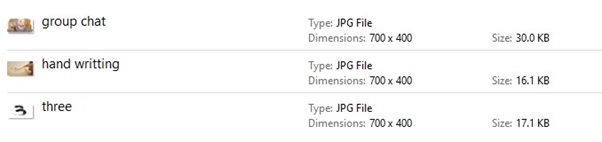
Renaming Images using the Batch Processing
重命名图像是在批量处理中经常执行的另一项任务。它涉及根据指定模式更改多张图像的文件名。
Renaming images is another frequently performed task in batch processing. It involves changing the filenames of multiple images according to a specified pattern.
Example
以下示例给指定 input_directory 中的一批 PNG 图像的名称添加前缀“New_”。它重命名这些图像并将它们保存到 output_directory 中,并使用了新名称。
The following example adds the prefix "New_" to the names of a batch of PNG images located in the specified input_directory. It renames these images and saves them in the output_directory with the new names.
from PIL import Image
import glob
import os
# Get a list of image files in the 'Images for Batch Operation' directory
input_directory = "Images for Batch Operation"
output_directory = "Output directory for Batch Operation"
image_files = [f for f in glob.glob(os.path.join(input_directory, "*.png"))]
for file in image_files:
image = Image.open(file)
# Save the image with the new name to the 'Output directory for Batch Operation' directory
output_path = os.path.join(output_directory, 'New_'+os.path.basename(file))
image.save(output_path)
以下图像显示了输入目录中可用的图像文件列表:
Following image represents the list of image files available in the input directory −
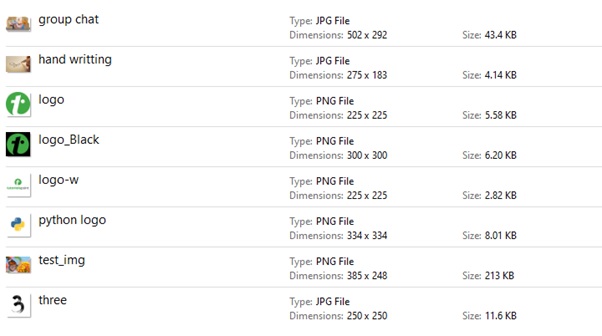
如果我们导航到输出图像保存的目录(即,“批量操作的输出目录”),我们将能够看到如下所示的重命名的图像:
If we navigate to the directory where the output image was saved (i.e, "Output directory for Batch Operation"), we will be able to observe renamed images as shown below −
