Python Pillow 简明教程
Python Pillow - Blur an Image
Blurring an Image 是图像处理中一个基本的概念,用于降低图像的细节或锐度级别。模糊的主要目的是使图像看起来更光滑或不太锐利。模糊可通过各种数学运算、卷积核或滤镜实现。
Python 的 Pillow 库在 ImageFilter 模块中提供了几个标准图像滤镜,通过调用 image.filter() 方法,针对图像执行不同的模糊操作。在本教程中,我们将看到 ImageFilter 模块提供的不同图像模糊滤镜。
Applying Blur Filter to an Image
可以使用 ImageFilter.BLUR 滤镜模糊图像,此滤镜是 Pillow 库当前版本中可用的内置滤镜选项之一,用于在图像中创建模糊效果。
以下是应用图像模糊的步骤 −
-
使用 Image.open() 函数加载输入图像。
-
将 filter() 函数应用于加载的 Image 对象,并将 ImageFilter.BLUR 作为参数提供给该函数。该函数将以 PIL.Image.Image 对象的形式返回模糊图像。
以下是本章所有示例中使用的输入图像。
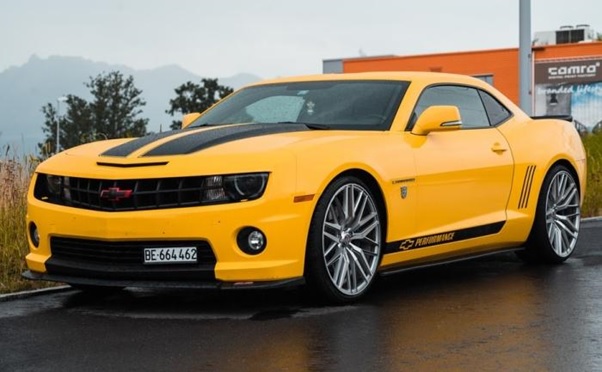
Example
以下是使用带有 ImageFilter.BLUR 核滤镜的 Image.filter() 方法的一个示例。
from PIL import Image, ImageFilter
# Open an existing image
original_image = Image.open('Images/car_2.jpg')
# Apply a blur filter to the image
blurred_image = original_image.filter(ImageFilter.BLUR)
# Display the original image
original_image.show()
# Display the blurred mage
blurred_image.show()

Applying the BoxBlur Filter
BoxBlur 滤镜用于通过将每个像素的值设置为向每个方向延伸指定像素数的方框内像素平均值来模糊图像。为此,你可以使用 Pillow 的 ImageFilter 模块中的 BoxBlur() 类。
以下是 ImageFilter.BoxBlur() 类的语法 −
class PIL.ImageFilter.BoxBlur(radius)
PIL.ImageFilter.BoxBlur() 类接受一个参数 −
-
radius −此参数指定用于在每个方向模糊的正方形大小。它可以作为两个数字序列(用于 x 和 y 方向)或适用于两个方向的单个数字提供。
如果半径设置为 1,它将取 3x3 方框内的像素值平均值(每个方向 1 个像素,总共 9 个像素)。半径值为 0 不模糊图像并返回相同图像。
Example
以下是一个演示如何使用 BoxBlur() 类进行图像模糊的示例。
from PIL import Image, ImageFilter
# Open an existing image
original_image = Image.open('Images/car_2.jpg')
# Apply a Box Blur filter to the image
box_blurred_image = original_image.filter(ImageFilter.BoxBlur(radius=3))
# Display the Box Blurred image
box_blurred_image.show()

Applying the Gaussian Blur to an Image
GaussianBlur 滤镜用于通过应用高斯模糊(一种近似于高斯分布的特定类型的模糊)来模糊图像。这可以通过使用 Pillow 的 ImageFilter.GaussianBlur() 类实现。
以下是 ImageFilter.GaussianBlur() 类的语法 −
class PIL.ImageFilter.GaussianBlur(radius=2)
ImageFilter.GaussianBlur() 类接受一个参数 −
-
radius −此参数指定高斯核的标准偏差。标准偏差控制模糊的范围。你可以将其提供为两个数字序列(用于 x 和 y 方向)或适用于两个方向的单个数字。
Example
以下是一个演示如何使用 GaussianBlur() 类进行图像模糊的示例。
from PIL import Image, ImageFilter
# Open an existing image
original_image = Image.open('Images/car_2.jpg')
# Apply a Gaussian Blur filter to the image
gaussian_blurred_image = original_image.filter(ImageFilter.GaussianBlur(radius=2))
# Display the Gaussian Blurred image
gaussian_blurred_image.show()
